Need help developing the following in Java: Create an abstract class "Shape" with the following specifications: An abstract method "surface_area ()" of return type double An abstract method "volume()" of return type double Put your code in a Java source file named "Shape.java." I. Create a class "Sphere" that extends the Shape class with the following specifications: Attributes: Radius Constructor: Implement a parameterized constructor needed to initialize the data. toString: Implement a "toString" method that prints out the surface area and volume. Implement methods to compute the surface area and volume. II. Create a class "Cylinder" that extends the Shape class with the following specifications: Attributes: Radius, height - both of type double Constructor: Implement a parameterized constructor to initialize the data. toString: Implement a "toString" method that prints out the surface area and volume. Implement methods to compute the surface area and volume. III. Create a class "Cone" that extends the Shape class with the following specifications: Attributes: Radius, height - both of type double Constructor: Implement a parameterized constructor to initialize the data. toString: Implement a "toString" method that prints out the surface area and volume. Implement methods to compute the surface area and volume. IV. Create a driver class named "ShapeArray" with the following specifications: Instantiate one sphere. Instantiate one cylinder. Instantiate one cone. Store the class instances into an array named "shapeArray." Loop through the array and print out the instance data of each object using the object instance's "toString" method.
Need help developing the following in Java:
Create an abstract class "Shape" with the following specifications:
- An abstract method "surface_area ()" of return type double
- An abstract method "volume()" of return type double
Put your code in a Java source file named "Shape.java."
I.
Create a class "Sphere" that extends the Shape class with the following specifications:
- Attributes:
- Radius
- Constructor:
- Implement a parameterized constructor needed to initialize the data.
- toString:
- Implement a "toString" method that prints out the surface area and volume.
Implement methods to compute the surface area and volume.
II.
Create a class "Cylinder" that extends the Shape class with the following specifications:
- Attributes:
- Radius, height - both of type double
- Constructor:
- Implement a parameterized constructor to initialize the data.
- toString:
- Implement a "toString" method that prints out the surface area and volume.
Implement methods to compute the surface area and volume.
III.
Create a class "Cone" that extends the Shape class with the following specifications:
- Attributes:
- Radius, height - both of type double
- Constructor:
- Implement a parameterized constructor to initialize the data.
- toString:
- Implement a "toString" method that prints out the surface area and volume.
Implement methods to compute the surface area and volume.
IV.
Create a driver class named "ShapeArray" with the following specifications:
- Instantiate one sphere.
- Instantiate one cylinder.
- Instantiate one cone.
- Store the class instances into an array named "shapeArray."
- Loop through the array and print out the instance data of each object using the object instance's "toString" method.

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 1 images

Is it possible to add a GUI interface for the program?
Can comments be added to describe the functionality of each class/method and any other important detail worth knowing about? Also, does the
Thank you
Can there be any improvements to the code to maximize efficiency, readability, and maintainability? What would that look like?
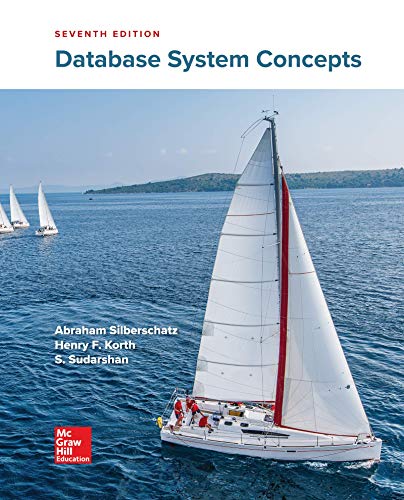
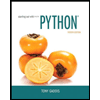
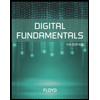
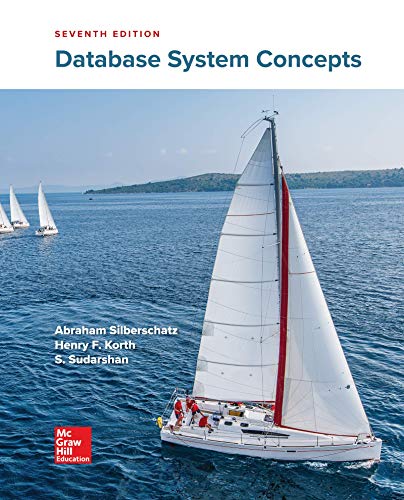
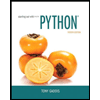
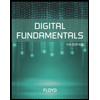
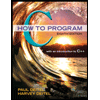
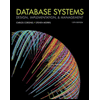
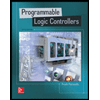