int total; int i; total = 0; for (i = 10; i > 0; i--) { totali; } The following translates this into ARM assembler: /* -- sum-to-ten.s */ .text .global start _start: end: mov re, #0 mov r1, #10 again: @re: 0 @r1 = 10 add re, re, r1@re: re + r1 r1 - 1 subs r1, r1, #1 @ r1 bne again. @loop again if we haven't hit zero. mov r7, #1 swie @setup exit @exit In the above assembly language program, we first use the MOV instruction to initialize RO at 0 and R1 at 10. The ADD instruction computes the sum of RO and R1 (the second and third arguments) and places the result into RO (the first argument); this corresponds to the total += i; line of the equivalent C program. Note the use of the label at the beginning of the ADD instruction. The label is simply the relative memory address of where that instruction is when the assembler loads it into memory. The subsequent SUBS instruction decreases R1 by 1. To understand the next instruction, we need to understand that in addition to the registers RO through R15, the ARM processor also incorporates a set of four "flags," labeled the zero flag (Z), the negative flag (N), the carry flag (C), and the overflow flag (V). Whenever an arithmetic instruction has an S at its end, as SUBS does, these flags will be updated based on the result of the
int total; int i; total = 0; for (i = 10; i > 0; i--) { totali; } The following translates this into ARM assembler: /* -- sum-to-ten.s */ .text .global start _start: end: mov re, #0 mov r1, #10 again: @re: 0 @r1 = 10 add re, re, r1@re: re + r1 r1 - 1 subs r1, r1, #1 @ r1 bne again. @loop again if we haven't hit zero. mov r7, #1 swie @setup exit @exit In the above assembly language program, we first use the MOV instruction to initialize RO at 0 and R1 at 10. The ADD instruction computes the sum of RO and R1 (the second and third arguments) and places the result into RO (the first argument); this corresponds to the total += i; line of the equivalent C program. Note the use of the label at the beginning of the ADD instruction. The label is simply the relative memory address of where that instruction is when the assembler loads it into memory. The subsequent SUBS instruction decreases R1 by 1. To understand the next instruction, we need to understand that in addition to the registers RO through R15, the ARM processor also incorporates a set of four "flags," labeled the zero flag (Z), the negative flag (N), the carry flag (C), and the overflow flag (V). Whenever an arithmetic instruction has an S at its end, as SUBS does, these flags will be updated based on the result of the
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:int total;
int i;
total = 0;
for (i = 10; i > 0; i--) {
total + i;
}
The following translates this into ARM assembler:
/* -- sum-to-ten.s */
.text
.global start
_start:
mov rº, #0
mov r1, #10
again:
end:
add r0, r0, r1
subs r1, r1, #1
bne again
mov r7, #1
swi 0
@r0 := 0
@r1 = 10
@r0 := r0 + r1
@ r1 := r1 - 1
@loop again if we haven't hit zero
@setup exit
@exit
In the above assembly language program, we first use the MOV instruction to initialize RO at O and R1 at 10. The ADD instruction computes the sum of RO and R1 (the second and third
arguments) and places the result into RO (the first argument); this corresponds to the total += i; line of the equivalent C program. Note the use of the label at the beginning of the ADD
instruction. The label is simply the relative memory address of where that instruction is when the assembler loads it into memory. The subsequent SUBS instruction decreases R1 by 1.
To understand the next instruction, we need to understand that in addition to the registers RO through R15, the ARM processor also incorporates a set of four "flags," labeled the zero flag (Z),
the negative flag (N), the carry flag (C), and the overflow flag (V). Whenever an arithmetic instruction has an S at its end, as SUBS does, these flags will be updated based on the result of the
computation. In this case, if the result of decreasing R1 by 1 results in 0, the Z flag will become 1; the N, C, and V flags are also updated, but they're not pertinent to our discussion of this code.
The following instruction, BNE, will check the Z flag. If the Z flag is not set (i.e., the previous subtraction gives a nonzero result), then BNE writes the address of the label "again" into the PC
(program counter) so that the next instruction executed is the ADD instruction on the line with the label "again"; this leads to repeating the loop with a smaller value of R1. If the Z flag is set
the BNE is branch is not executed (this is the conditional execution), so the processor will simply continue on to the next instruction.
Assemble and link the sample code and verify it produces the correct sum. Once you have this sample program functioning, modify it so it sums the even numbers from 0 to 16. Submit the
modified .s file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
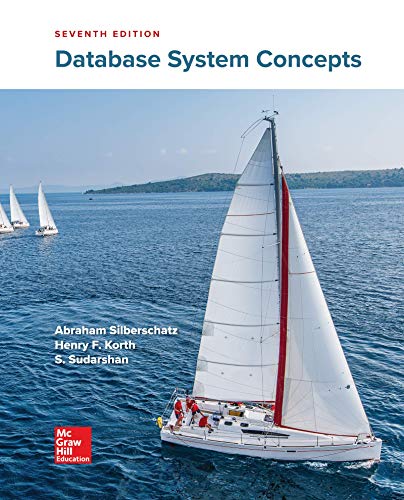
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
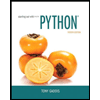
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
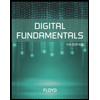
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
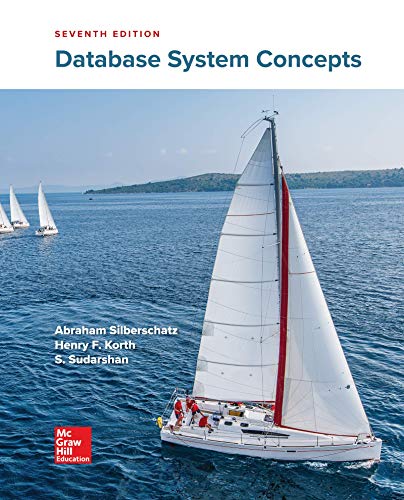
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
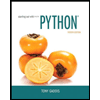
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
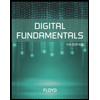
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
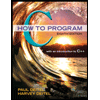
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
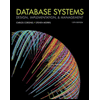
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
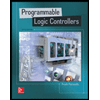
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education