#include winclude #include using namespace std; // TODo: fill in the code to create a new board // The board is an array of arrays, create the container array // Then add arrays for a diagonal with 1 more than the row index // Initialize ALi elements to e to avoid corrupting the count int** createNewBoard(int rows) I/ ToDo: delete the arrays that make up the board // The board is an array of arrays, delete the arrays void freeboard(int *board[], int rows) // runsimulation does the logic to run the Galton board // to see how the balls drop through_the pegs. // @param: board is a two-dimensional diagonal array. // @param: nTests is the number of tests to run. void runsimulations(int *board[], int rows, int nTests) int i, j; // Loop to run the number of tests for (int trials - ®; trials < nTests; trials++) !/ Dropping a ball in always hits the first peg. i-j- e; board[i][]]++; simulate the ball dropping. // It either falls straight down or bounces to the right. // But gravity always takes it down to the bottom for (i - 1; i < rows; i++) int right = rand()%2; if (right -- 1) j++; board[i][j]++; // ToDo: display the board values as a nice triangle chart. // use setw(4) to separate values in a row. // eparam: board is a two-dimensional diagonal array void display(int *board[], int rows) int main() int boardsize, numTests, seed; cout « "Enter the size of the board: "; cin » boardsize ; cout « "Enter the number of tests: "; cin >» numTests; cout « "Enter the random seed: "; cin » seed; srand(seed); I/ create the Galton Board, run simulation, display results and free int "board - createNewBoard(boardsize); runsimulations(board, boardsize, numTests); display(board, boardsize); freeboard(board, boardsize); return e;
#include winclude #include using namespace std; // TODo: fill in the code to create a new board // The board is an array of arrays, create the container array // Then add arrays for a diagonal with 1 more than the row index // Initialize ALi elements to e to avoid corrupting the count int** createNewBoard(int rows) I/ ToDo: delete the arrays that make up the board // The board is an array of arrays, delete the arrays void freeboard(int *board[], int rows) // runsimulation does the logic to run the Galton board // to see how the balls drop through_the pegs. // @param: board is a two-dimensional diagonal array. // @param: nTests is the number of tests to run. void runsimulations(int *board[], int rows, int nTests) int i, j; // Loop to run the number of tests for (int trials - ®; trials < nTests; trials++) !/ Dropping a ball in always hits the first peg. i-j- e; board[i][]]++; simulate the ball dropping. // It either falls straight down or bounces to the right. // But gravity always takes it down to the bottom for (i - 1; i < rows; i++) int right = rand()%2; if (right -- 1) j++; board[i][j]++; // ToDo: display the board values as a nice triangle chart. // use setw(4) to separate values in a row. // eparam: board is a two-dimensional diagonal array void display(int *board[], int rows) int main() int boardsize, numTests, seed; cout « "Enter the size of the board: "; cin » boardsize ; cout « "Enter the number of tests: "; cin >» numTests; cout « "Enter the random seed: "; cin » seed; srand(seed); I/ create the Galton Board, run simulation, display results and free int "board - createNewBoard(boardsize); runsimulations(board, boardsize, numTests); display(board, boardsize); freeboard(board, boardsize); return e;
Chapter7: Using Methods
Section: Chapter Questions
Problem 20RQ
Related questions
Question
Please complete the code, in C++ to pass the tests, the first image is the assignment, the second screenshot provides the starter code. The Test case will be:
Sample Test Case:
Enter the size of the board: 7
Enter the number of tests: 500
Enter the random seed: 17
500
248 252
135 239 126
72 188 182 58
36 132 177 124 31
19 71 180 139 71 20
7 45 130 155 105 46 12
![#include <iostream>
#include <iomanip>
#include <cstdlib>
using namespace std;
// TODO: fill in the code to create a new board
// The board is an array of arrays, create the container array
// Then add arrays for a diagonal with 1 more than the row index
// Initialize ALL elements to e to avoid corrupting the count
int** createNewBoard (int rows)
{
}
// TODO: delete the arrays that make up the board
// The board is an array of arrays, delete the arrays
void freeboard(int *board[], int rows)
// runsimulation does the logic to run the Galton board
// to see how the balls drop through the pegs.
// eparam: board is a two-dimensional diagonal array.
// eparam: rows is the board size
// eparam: nTests is the number of tests to run.
void runsimulations (int *board[], int rows, int nTests)
{
int i, j;
// Loop to run the number of tests
for (int trials = 0; trials < nTests; trials++)
{
// Dropping a ball in always hits the first peg.
i = j = e;
board[i][j]++;
// Simulate the ball dropping.
// It either falls straight down or bounces to the right.
// But gravity always takes it down to the bottom
for (i = 1; i < rows; i++)
{
int right = rand ()%2;
if (right == 1) j++;
board[i][j]++;
}
}
// TODO: display the board values as a nice triangle chart.
// Use setw(4) to separate values in a row.
// eparam: board is a two-dimensional diagonal array
// éparam: rows is the board size
void display(int *board[], int rows)
{
}
int main()
{
int boardsize, numTests, seed;
cout « "Enter the size of the board: ";
cin >> boardsize ;
cout <« "Enter the number of tests: ";
cin >> numTests;
cout « "Enter the random seed: ";
cin >> seed;
srand(seed);
// Create the Galton Board, run simulation, display results and free the board
int **board = createNewBoard(boardsize);
runsimulations (board, boardsize, numTests);
display(board, boardsize);
freeboard (board, boardsize);
return e;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff5bb9956-2539-4c8d-9ee9-02ed0be838be%2F51fed10f-aa81-4217-8b63-5cfac599f472%2Fr4dy8x_processed.png&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <iomanip>
#include <cstdlib>
using namespace std;
// TODO: fill in the code to create a new board
// The board is an array of arrays, create the container array
// Then add arrays for a diagonal with 1 more than the row index
// Initialize ALL elements to e to avoid corrupting the count
int** createNewBoard (int rows)
{
}
// TODO: delete the arrays that make up the board
// The board is an array of arrays, delete the arrays
void freeboard(int *board[], int rows)
// runsimulation does the logic to run the Galton board
// to see how the balls drop through the pegs.
// eparam: board is a two-dimensional diagonal array.
// eparam: rows is the board size
// eparam: nTests is the number of tests to run.
void runsimulations (int *board[], int rows, int nTests)
{
int i, j;
// Loop to run the number of tests
for (int trials = 0; trials < nTests; trials++)
{
// Dropping a ball in always hits the first peg.
i = j = e;
board[i][j]++;
// Simulate the ball dropping.
// It either falls straight down or bounces to the right.
// But gravity always takes it down to the bottom
for (i = 1; i < rows; i++)
{
int right = rand ()%2;
if (right == 1) j++;
board[i][j]++;
}
}
// TODO: display the board values as a nice triangle chart.
// Use setw(4) to separate values in a row.
// eparam: board is a two-dimensional diagonal array
// éparam: rows is the board size
void display(int *board[], int rows)
{
}
int main()
{
int boardsize, numTests, seed;
cout « "Enter the size of the board: ";
cin >> boardsize ;
cout <« "Enter the number of tests: ";
cin >> numTests;
cout « "Enter the random seed: ";
cin >> seed;
srand(seed);
// Create the Galton Board, run simulation, display results and free the board
int **board = createNewBoard(boardsize);
runsimulations (board, boardsize, numTests);
display(board, boardsize);
freeboard (board, boardsize);
return e;
![DESCRIPTION
Requirements
In the starter code you will find a program to simulate a Galton Board. The code for the simulation is given. It
assumes these formal parameters: a Galton Board, the board size and the number of tests to run. Function stubs
are provided for the following functions:
- int** createNewBoard (int rows);
void display (int* board[], int rows);
- void freeBoard (int* board[], int rows);
-
You must fill in the details of the function stubs to dynamically allocate the board, display it and free it once the
program is done using it. This will allow you to practice dynamic allocation and deletion of a 2-dlmenslonal
Jagged array.
Directions
The original problem can be found in Big C++ 3ed chapter 7.5. Read chapter sections 7.1 - 7.4 for supporting
content on pointers and dynamic allocation (though 7.3 is not needed for this assignment, it will be needed
later). The lectures and narrated power points with more details are on Blackboard.
int** createNewBoard (int rows);
Dynamically allocate the board array using the new operator that we learned about in class. Then loop to
allocate the individual rows. Don't forget to initialize the array elements to 0. This can be done directly when
allocating the arrays (the same as if statically allocated) or in a loop afterwards.
- void display (int* board[], int rows);
Display the individual values in a row of the array on a single line spaced 4 characters apart using setw (4) as
part of the cout Sstatement.
- void freeboard (int* board[], int rows);
Deallocate the Galton board now that we're done using it. Use the delete operator that we learned about in
class. Remember the order that we deallocate is the reverse order that we used to create the board.
Sample Use Case
Enter the size of the board: 7
Enter the number of tests: 500
Enter the random seed: 17
500
248 252
135 239 126
72 188 182 58
36 132 177 124 31
19 71 180 139 71 20
7 45 130 155 105 46 12](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff5bb9956-2539-4c8d-9ee9-02ed0be838be%2F51fed10f-aa81-4217-8b63-5cfac599f472%2Fi0k21d_processed.png&w=3840&q=75)
Transcribed Image Text:DESCRIPTION
Requirements
In the starter code you will find a program to simulate a Galton Board. The code for the simulation is given. It
assumes these formal parameters: a Galton Board, the board size and the number of tests to run. Function stubs
are provided for the following functions:
- int** createNewBoard (int rows);
void display (int* board[], int rows);
- void freeBoard (int* board[], int rows);
-
You must fill in the details of the function stubs to dynamically allocate the board, display it and free it once the
program is done using it. This will allow you to practice dynamic allocation and deletion of a 2-dlmenslonal
Jagged array.
Directions
The original problem can be found in Big C++ 3ed chapter 7.5. Read chapter sections 7.1 - 7.4 for supporting
content on pointers and dynamic allocation (though 7.3 is not needed for this assignment, it will be needed
later). The lectures and narrated power points with more details are on Blackboard.
int** createNewBoard (int rows);
Dynamically allocate the board array using the new operator that we learned about in class. Then loop to
allocate the individual rows. Don't forget to initialize the array elements to 0. This can be done directly when
allocating the arrays (the same as if statically allocated) or in a loop afterwards.
- void display (int* board[], int rows);
Display the individual values in a row of the array on a single line spaced 4 characters apart using setw (4) as
part of the cout Sstatement.
- void freeboard (int* board[], int rows);
Deallocate the Galton board now that we're done using it. Use the delete operator that we learned about in
class. Remember the order that we deallocate is the reverse order that we used to create the board.
Sample Use Case
Enter the size of the board: 7
Enter the number of tests: 500
Enter the random seed: 17
500
248 252
135 239 126
72 188 182 58
36 132 177 124 31
19 71 180 139 71 20
7 45 130 155 105 46 12
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
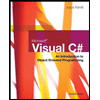
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
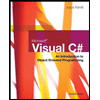
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage