#include #include #include int main(void){ int money_start, bet, money_bet; int money_result; int randomNumber = 0; int n = 0; srand(time(0)); randomNumber = rand() % n; printf("Entering the casino, how much money do you have? "); scanf("%d", &money_start); printf("We are playing roulette, odd or even bets only.\nPlace your bet!"); printf("Enter 1 for odd, 2 for even, 0 to quit: "); scanf("%d", &bet); printf("How much money do you want to bet? "); scanf("%d", &money_bet); while (( bet == 1 || bet == 2 )) { if (( bet == 1)) { printf("You bet $%d on odd numbers.", money_bet); if ((randomNumber % 2 == 0)) { money_result = money_start - money_bet; printf("You lose!"); printf("Your balance is $%d!", money_result);} else { if ((randomNumber % 2 == 1)) { money_result = money_start + money_bet; printf("You win!"); printf("Your balance is $%d!", money_result); } } if ((bet == 2)) printf("You bet $%d on even numbers.", money_bet); if ((randomNumber % 2 == 0)) { money_result = money_start + money_bet; printf("You win!"); printf("Your balance is $%d!", money_result); } else { if ((randomNumber % 2 == 1)) { money_result = money_start - money_bet; printf("You lose!");}} printf("Your balance is $%d!", money_result); } } if ((bet == 0)) printf("You exit the casino with %d", money_result); return 0; } This is my code, but I keep getting "Floating exception (core dumped)" what should I do to fix this?
#include <stdlib.h>
#include <time.h>
#include <stdio.h>
int main(void){
int money_start, bet, money_bet;
int money_result;
int randomNumber = 0;
int n = 0;
srand(time(0));
randomNumber = rand() % n;
printf("Entering the casino, how much money do you have? ");
scanf("%d", &money_start);
printf("We are playing roulette, odd or even bets only.\nPlace your bet!");
printf("Enter 1 for odd, 2 for even, 0 to quit: ");
scanf("%d", &bet);
printf("How much money do you want to bet? ");
scanf("%d", &money_bet);
while (( bet == 1 || bet == 2 ))
{
if (( bet == 1))
{
printf("You bet $%d on odd numbers.", money_bet);
if ((randomNumber % 2 == 0))
{ money_result = money_start - money_bet;
printf("You lose!");
printf("Your balance is $%d!", money_result);}
else { if ((randomNumber % 2 == 1))
{ money_result = money_start + money_bet;
printf("You win!");
printf("Your balance is $%d!", money_result);
}
}
if ((bet == 2))
printf("You bet $%d on even numbers.", money_bet);
if ((randomNumber % 2 == 0))
{ money_result = money_start + money_bet;
printf("You win!");
printf("Your balance is $%d!", money_result);
}
else { if ((randomNumber % 2 == 1))
{ money_result = money_start - money_bet;
printf("You lose!");}}
printf("Your balance is $%d!", money_result);
}
}
if ((bet == 0))
printf("You exit the casino with %d", money_result);
return 0;
}
This is my code, but I keep getting "Floating exception (core dumped)" what should I do to fix this?

Objective: A program is provided for implementing the roulette game where a player will play the game and he may win or lose it. We need to fix errors in this given program.
Programming language: C
Answer:
#include <stdlib.h>
#include <stdio.h>
#include <time.h>
int main(void){
int money_start, bet, money_bet;
int money_result;
int randomNumber = 0;
int n = 0;
printf("Entering the casino, how much money do you have? ");
scanf("%d", &money_start);
printf("We are playing roulette, odd or even bets only.\nPlace your bet!");
do
{
printf("Enter 1 for odd, 2 for even, 0 to quit: ");
scanf("%d", &bet);
srand(time(0));
randomNumber = rand();
if ( bet == 1)
{
printf("How much money do you want to bet? ");
scanf("%d", &money_bet);
printf("You bet $%d on odd numbers.", money_bet);
if (randomNumber % 2 == 0)
{ money_result = money_start - money_bet;
printf("You lose!");
printf("Your balance is $%d!", money_result);
}
else if (randomNumber % 2 == 1)
{ money_result = money_start + money_bet;
printf("You win!");
printf("Your balance is $%d!", money_result);
}
}
if (bet == 2){
printf("How much money do you want to bet? ");
scanf("%d", &money_bet);
printf("You bet $%d on even numbers.", money_bet);
if (randomNumber % 2 == 0)
{
money_result = money_start + money_bet;
printf("You win!");
printf("Your balance is $%d!", money_result);
}
else if (randomNumber % 2 == 1)
{
money_result = money_start - money_bet;
printf("You lose!");
printf("Your balance is $%d!", money_result);
}
}
if (bet == 0)
printf("You exit the casino with %d", money_result);
} while( bet == 1 || bet == 2 );
return 0;
}
Step by step
Solved in 3 steps with 1 images

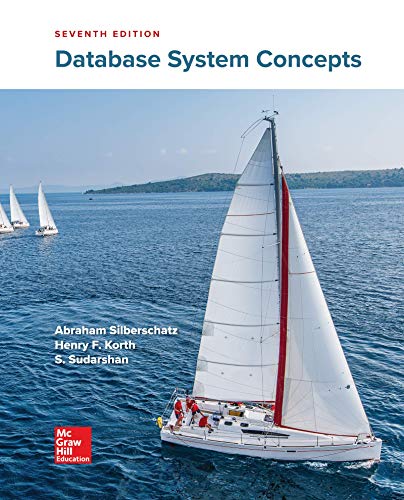
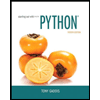
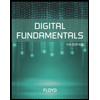
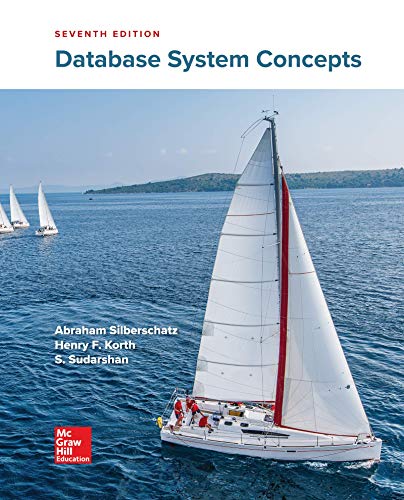
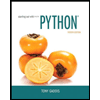
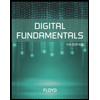
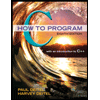
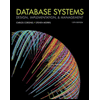
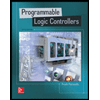