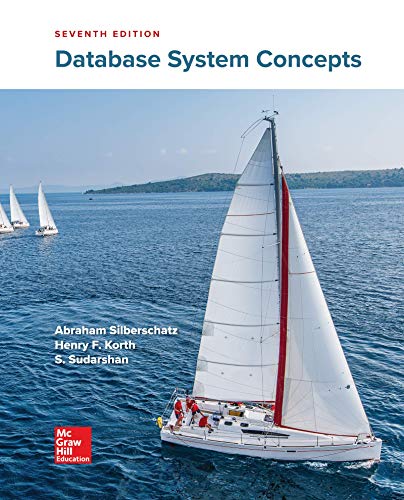
In this program I'm currently working on I need help with figuring out:
Write a C++ program that reads text from a file called letter_count.txt. The program determines which alphabetic character occurs most frequently in the text and which alphabetic character appears least frequently in the text.
Currently, I need help with figuring out how to accumulate a total for the lowercase letters and ignore the special characters.
Here is the letter_count.text data:
To be, or not to be--that is the question:
Whether 'tis nobler in the mind to suffer
The slings and arrows of outrageous fortune
Or to take arms against a sea of troubles
And by opposing end them. To die, to sleep--
No more--and by a sleep to say we end
The heartache, and the thousand natural shocks
That flesh is heir to. 'Tis a consummation
Devoutly to be wished. To die, to sleep--
To sleep--perchance to dream: ay, there's the rub,
For in that sleep of death what dreams may come
When we have shuffled off this mortal coil,
Must give us pause. There's the respect
That makes calamity of so long life.
For who would bear the whips and scorns of time,
Th' oppressor's wrong, the proud man's contumely
The pangs of despised love, the law's delay,
The insolence of office, and the spurns
That patient merit of th' unworthy takes,
When he himself might his quietus make
With a bare bodkin? Who would fardels bear,
To grunt and sweat under a weary life,
But that the dread of something after death,
The undiscovered country, from whose bourn
No traveller returns, puzzles the will,
And makes us rather bear those ills we have
Than fly to others that we know not of?
Thus conscience does make cowards of us all,
And thus the native hue of resolution
Is sicklied o'er with the pale cast of thought,
And enterprise of great pitch and moment
With this regard their currents turn awry
And lose the name of action. -- Soft you now,
The fair Ophelia! -- Nymph, in thy orisons
Be all my sins remembered.
Here is my code so far:
// Lab02.cpp : This program reads from the text found in the file and determines
// which letter has occured the most and the least.
//Project done by Alisha Erickson
#include <iostream>
#include <fstream>
#include <string>
#include <cctype>
#include <iomanip>
using namespace std;
//Function prototypes
/*void bubbleSort(char results[], string input);*/
int main()
{
string input; //To hold the line in the file.
ifstream inputFile; //File stream object.
const int ALPHABET = 26;
char result[ALPHABET] = { 'a' + 1};
char lowerCase;
char checkCharacter = 'a';
int count = 0;
int letterCount;
//Opens the file
inputFile.open("letter_count.txt", ios::out);
//If it was successfully opened, continue.
if (inputFile)
{
//Reads an item from the file.
while (getline(inputFile, input)) //To be or not to be, that is the question; = input;
{
cout << input << endl;
int x = input.size();
for (int i = 0; i < x; i++) //T = input[0]
{
lowerCase = tolower(input[i]); //t
cout << lowerCase << endl;
for ( int count = 0; count < 26; count++) //compare t to a
{
if (lowerCase == 'a' + count)
{
letterCount[count + ‘a’] = letterCount[count – ‘a’] + 1;
}
}
/* bubbleSort(result, input);*/
}
}
//Continues on from the last successful read
//operation.
//Close file.
inputFile.close();
}
//If an error happens, cout error message.
else
{
cout << "ERROR: The file that was input could not be read.";
}
return 0;
}
/*
void bubbleSort(char results[], string input)
{
int maxElement;
int index;
for (maxElement = - 1; maxElement > 0; maxElement--)
{
for (index = 0; index < maxElement; index++)
{
if (results[index] > results[index + 1])
{
swap(results[index], results[index + 1]);
}
}
}
}
*/

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- Create a program that reads in a word from the user and counts the number of occurrences of that word in a file called words.txt. -- words.txt This is a file that contains many words. Yes it does have so many words. Many, many words. Well, maybe it is not that many after all. So, just how many is MANY? in c++ pleasearrow_forwardin the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forwardPlease write a SIMPLE C++ Code that takes the equation numbers of A,B and C from the input file, adds the 2 polynomials equations adjacent to each other together and then print out the results to an output file. Please include all files that should be in the code to your response. also make sure that the code is runnable. The prompt with all other directions is down below. A mini sample code is also there if you need any helparrow_forward
- Java Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardHi, I need to solve this problem with C++ programming language using Visual Studio. Thank you.arrow_forward The fields below repeat for each customer: o Customer name (String)o Customer ID (numeric integer) o Bill balance (numeric)o EmailAddress (String)o Tax liability (numeric or String) The customers served by the office supply store are of two types: tax-exempt or non-tax- exempt. For a tax-exempt customer, the tax liability field on the file is the reason for the tax exemptions: education, non-profit, government, other (String). For a non-tax exempt customer, the tax liability field is the percent of tax that the customer will pay (numeric) based on the state where the customer’s business resides. Program requirements: From the information provided, write a solution that includes the following: A suitable inheritance hierarchy which represents the customers serviced by the office supply company. It is up to you how to design the inheritance hierarchy. I suggest a Customer class and appropriate subclasses.. For all classes include the following: o Instance variables o…arrow_forward
- please help on this python problem, produce new codarrow_forwardNeed to do this in language as a while little confused on how to do it?arrow_forward9.Code please. In this exercise you will create a program that will be used in a digital library to format and sort journal entries based on the authors last name. Each entry has room to store only the last name of the author. Begin by removing the first name "Isaac" from the string variable journal_entry_1 by using the string function erase. Do not forget to also remove the whitespace so that the string variable journal_entry_1 will then contain the string "Newton" with no whitespaces. The journal entries should be sorted alphabetically based on the authors last name. For example, the last name "Brown" should come before the last name "Davis" Create an if statement so that if the last name contained within journal_entry_2 is alphabetically less than the last name contained within journal_entry_1, then the string values are swapped using the string function swap. You may use either of the comparison operators < > in the if statement but remember that following ASCII, "A" is…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
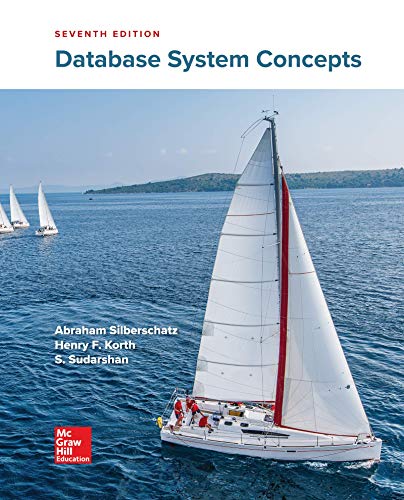
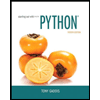
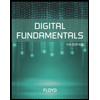
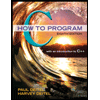
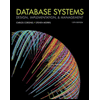
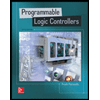