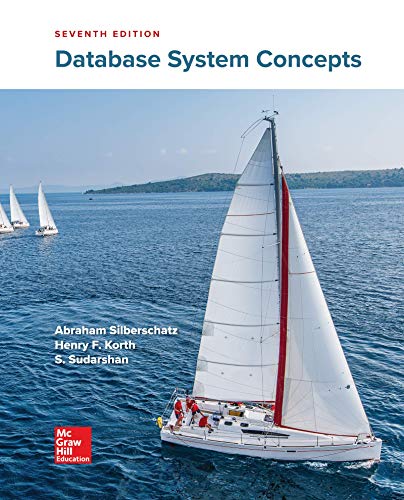
Concept explainers
In this
Here is the table structure:
CREATE TABLE USER_ (
USER_ID INT NOT NULL AUTO_INCREMENT,
CREATE_DATE DATE NOT NULL,
PROF_DESC VARCHAR (100),
PROF_PIC VARCHAR(40),
LOCATION VARCHAR (40) NOT NULL,
PRIMARY KEY (USER_ID)
);
CREATE TABLE USER_INFO(
USER_ID INT NOT NULL,
SU_EMAIL CHAR(18),
USER_FNAME VARCHAR(15),
USER_LNAME VARCHAR (15),
USER_DOB DATE,
USER_GENDER CHAR(1),
PRIMARY KEY (USER_ID),
FOREIGN KEY (USER_ID) REFERENCES USER_(USER_ID) ON UPDATE CASCADE
);
CREATE TABLE STUDENT (
SU_ID INT NOT NULL,
USER_ID INT NOT NULL,
YEAR_ VARCHAR (10),
MAJOR VARCHAR (20),
LOCATION VARCHAR (40) NOT NULL,
PRIMARY KEY (SU_ID),
FOREIGN KEY (USER_ID) REFERENCES USER_(USER_ID) ON UPDATE CASCADE
);
CREATE TABLE INSTRUCTOR (
SU_ID INT NOT NULL,
USER_ID INT NOT NULL,
DEPARTMENT VARCHAR (40),
INSTR_TYPE VARCHAR (40),
PRIMARY KEY (SU_ID),
FOREIGN KEY (USER_ID) REFERENCES USER_(USER_ID) ON UPDATE CASCADE
);
CREATE TABLE GROUP_(
GROUP_ID INT NOT NULL AUTO_INCREMENT,
GROUP_NAMEVARCHAR(50) NOT NULL,
CREATE_DATE DATE NOT NULL,
PRIMARY KEY (GROUP_ID)
);
CREATE TABLE MEMBERSHIP (
GROUP_ID INT NOT NULL,
USER_ID INT NOT NULL,
MEMBER_DESC VARCHAR (10),
PRIMARY KEY (GROUP_ID, USER_ID),
FOREIGN KEY (GROUP_ID) REFERENCES GROUP_(GROUP_ID) ON UPDATE CASCADE,
FOREIGN KEY (USER_ID) REFERENCES USER_(USER_ID) ON UPDATE CASCADE
);
CREATE TABLE MEDIA(
MEDIA_ID VARCHAR(40) NOT NULL,
MEDIA_TYPE CHAR(1) NOT NULL,
MEDIA_TITTLE VARCHAR(50),
PRIMARY KEY (MEDIA_ID)
);
CREATE TABLE POST(
POST_ID CHAR(4) NOT NULL,
USER_ID INT NOT NULL,
MEDIA_ID VARCHAR(40) NOT NULL,
POST_DATE DATE NOT NULL,
PRIMARY KEY (POST_ID),
FOREIGN KEY (USER_ID) REFERENCES USER_(USER_ID) ON UPDATE CASCADE,
FOREIGN KEY (MEDIA_ID) REFERENCES MEDIA(MEDIA_ID) ON UPDATE CASCADE
);
CREATE TABLE VIDEO(
MEDIA_ID VARCHAR(40),
V_LENGTH INT,
V_DESCRIPTION VARCHAR (100),
PRIMARY KEY (MEDIA_ID),
FOREIGN KEY (MEDIA_ID) REFERENCES MEDIA(MEDIA_ID) ON UPDATE CASCADE
);
CREATE TABLE PHOTO(
MEDIA_ID VARCHAR(40),
P_RESOLUTION INT,
P_CAPTION VARCHAR (100),
PRIMARY KEY (MEDIA_ID),
FOREIGN KEY (MEDIA_ID) REFERENCES MEDIA(MEDIA_ID) ON UPDATE CASCADE
);
CREATE TABLE TEXT_(
MEDIA_ID VARCHAR(40),
T_LENGTH INT,
T_CONTENT VARCHAR (1000),
PRIMARY KEY (MEDIA_ID),
FOREIGN KEY (MEDIA_ID) REFERENCES MEDIA(MEDIA_ID) ON UPDATE CASCADE
Fot this database write sql code for the following:
(1) Queries involving subqueries
(1) Queries involving GROUP BY and HAVING clause
(1) Queries involving left outer join or right outer join of two tables

In this question we have to write SQL QUERIES on (1) Queries involving subqueries
(2) Queries involving GROUP BY and HAVING clause
(3) Queries involving left outer join or right outer join of two tables
Let's query and hope this helps if you find any query on solution, utilize threaded question feature.
Step by stepSolved in 2 steps

- Sales Database: Customers(custId, lastName, firstName, address, phone, creditLimit) Orders(ordNumber, itemNumber, qtyOrdered.) Items(itemNumber, itemName, price) For the Sales Database referenced above, write the SQL command to create the LineItem table, assuming the Orders table and items table already exist.arrow_forwardIn SQL This sample database consists of the following tables(see image for tables and there is one more at the bottom in this text):• Customers: stores customer’s data• Products: stores a list of scale model cars• ProductLines: stores a list of product line categories• Orders: stores sales orders placed by customers• OrderDetails: stores sales order line items for each sales order• Payments: stores payments made by customers based on their accounts• Employees: stores all employee information as well as the organization structuresuch as who reports to whom• Offices: stores sales office data Write SQL code for the following:We want to add a new sale order for the customer (customerNumber = 145) in thedatabase. The steps of adding a sale order are described as follows:(1) Get latest sale order number from “orders” table, and use the next sale ordernumber as the new sale order number(2) Insert a new sale order into “orders” table for the customer (customerNumber =145). For this order, the…arrow_forwardwith it, paste your code in a text file named Student.txt and save it in your task folder. Write the SQL code to create a table called Student. The table structure is summarised in the table below (Note that STU_NUM is the primary key): STU_NUM Attribute Name STU_SNAME STU_FNAME STU_INITIAL STU_STARTDATE COURSE_CODE PROJ_NUM STU STU_S STU_F STU_INITIAL NUM NAME NAME 01 02 Snow John E CHAR(6) After you have created the table in question 1, write the SQL code to enter the first two rows of the table as below: Stark Arya с VARCHAR(15) VARCHAR(15) CHAR(1) DATE CHAR(3) INT(2) Data Type STU_STARTDATE COURSE_ PROJ_ CODE NUM 05-Apr-14 12-Jul-17 201 305 6 11 Assuming all the data in the Student table has been entered as shown below, write the SQL code that will list all attributes for a COURSE_CODE of 305.arrow_forward
- create database GA2gouse GA2gocreate table salesman(salesman_id int primary key, name varchar(30), city varchar(20), commission varchar(30));insert into salesman (salesman_id, name, city, commission) values (5001, 'James Hoog', 'New York', 0.15); insert into salesman (salesman_id, name, city, commission) values (5002, 'Nail Knite', 'Paris', 0.13); insert into salesman (salesman_id, name, city, commission) values (5005, 'Pit Alex', 'London', 0.11); insert into salesman (salesman_id, name, city, commission) values (5006, 'Mc Lyon', 'Paris', 0.14); insert into salesman (salesman_id, name, city, commission) values (5007, 'Paul Adam', 'Rome', 0.13); insert into salesman (salesman_id, name, city, commission) values (5003, 'Lauson Hen', 'San Jose', 0.12); Create table Customer (customer_id int primary key, cust_name varchar(30), city varchar(10), grade varchar(30), salesman_id int,CONSTRAINT FK_salesman_id FOREIGN KEY (salesman_id) REFERENCES salesman(salesman_id));insert into customer…arrow_forwardMySql Workbench CREATE TABLE students ( id INT PRIMARY KEY, first_name VARCHAR(50), last_name VARCHAR(50), age INT, major VARCHAR(50), faculty VARCHAR(50)); CREATE TABLE location ( id INT PRIMARY KEY, name VARCHAR(50), rooms INT); CREATE TABLE faculty ( id INT PRIMARY KEY, name VARCHAR(50), department_id INT); 1. List last name of all students whose first name is longer than 4 letters in ascending order accordingto the last name. Duplicated rows should be removed from the output.2. Count the total number of rooms in Location.3. Find the number of students in each major.4. Find the number of employees in each department who get no commission or have salary less than5000.5. Find the maximum salary of employees in each department that the employee was hired 15 yearsbefore now. *hint: user TIMESTAMPDIFF(<unit type>,<Date_value 1>,<Date_value 2>), the unitcan be YEAR, MONTH, DAY, HOUR, etc...arrow_forwardProblem: JMS TechWizards is a local company that provides technical services to several small businesses in the area. The company currently keeps its technicians and clients’ records on papers. The manager requests you to create a database to store the technician and clients’ information. The following table contains the clients’ information. Client Number Client Name Street City State Postal Code Telephone Number Billed Paid Technician Number AM53 Ashton-Mills 216 Rivard Anderson TX 78077 512-555-4070 $315.50 $255.00 22 AR76 The Artshop 722 Fisher Liberty Corner TX 78080 254-555-0200 $535.00 $565.00 23 BE29 Bert's Supply 5752 Maumee Liberty Corner TX 78080 254-555-2024 $229.50 $0.00 23 DE76 D & E Grocery 464 Linnell Anderson TX 78077 512-555-6050 $485.70 $400.00 29 GR56 Grant Cleaners 737 Allard Kingston TX 78084 512-555-1231 $215.00 $225.00 22…arrow_forward
- 1-Write the syntax to insert into a relational table called students the address column references an object table called addresses that was created using an address_type. Other columns exist in the students table, but you are only inserting into the ones below. Aliases used should be the first letter of the table name, eg students would be s, addresses would be a Relational Table Name students attribute student_id attribute surname attribute addressarrow_forwardAssume that a database has a table named Stock, with the following columns:Column Name TypeTrading_Symbol nchar(10)Company_Name nchar(25)Num_Shares intPurchase_Price moneySelling_Price moneyWrite a Select statement that returns the Trading_Symbol column only from the rows where Purchase_Price is greater than $25.00.arrow_forwardCreate a stored procedure that will return the number of customers in a given state. The parameter for your stored procedure should accept the state abbreviation ('UT') and return the results of a query that returns the number of customers in that state.arrow_forward
- You have given a table from a database and the name of the table is Employees Please display the following from the Employees 1) The Employee Id, Last Name and First Name for each employee in order in terms of Last Name. 2) The employees who is title is Sales Representative 3) Delete the employee whose title of courtesy is Dr. 4) Update the title of the employee whose title is Sales Manager to Senior Sales Manager.arrow_forwardAccess Assignment Problem: JMS TechWizards is a local company that provides technical services to several small businesses in the area. The company currently keeps its technicians and clients’ records on papers. The manager requests you to create a database to store the technician and clients’ information. The following table contains the clients’ information. Client Number Client Name Street City State Postal Code Telephone Number Billed Paid Technician Number AM53 Ashton-Mills 216 Rivard Anderson TX 78077 512-555-4070 $315.50 $255.00 22 AR76 The Artshop 722 Fisher Liberty Corner TX 78080 254-555-0200 $535.00 $565.00 23 BE29 Bert's Supply 5752 Maumee Liberty Corner TX 78080 254-555-2024 $229.50 $0.00 23 DE76 D & E Grocery 464 Linnell Anderson TX 78077 512-555-6050 $485.70…arrow_forwardThe Save Transaction button depicted in the screen attached is used to save relevant data to the sales table and the salesdetails tables from the depicted schema. When this button is clicked it calls the saveTransaction() function that is within the PosDAO class, it passes to this function an ArrayList of salesdetails object, this list contains the data entered into the jTable which is the products and qty being sold.Write the saveTransaction function. You are to loop through the items and get the total sales, next you are to insert the current date and the total sales into the sales table. Reminder that the sales table SalesNumber field is set to AUTO-INCREMENT, hence the reason for only entering the total sales and current date in sales table.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
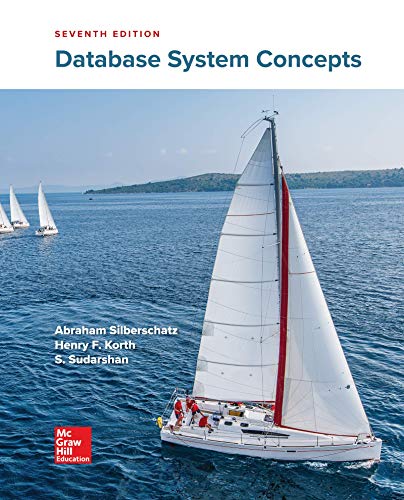
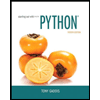
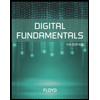
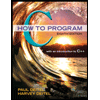
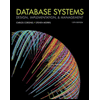
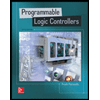