In ShipTest: • make two objects with declared type Ship but actual type CruiseShip. • make one object of declared type Ship but actual type CargoShip. • make one object of declared type CargoShip and actual type CargoShip. • make a ship of declared type WarShip and actual type WarShip. • using the array initializer syntax, code all of these ships into an array of type Ship named fleet. • code a for loop that processes the fleet array to show display all ships and their data. • use a method of class Arrays to create an ArrayList of type Ship from the fleet array. • pass this ArrayList to a method named shipShow that also displays all ships and returns an integer. • report the value of the integer returned by shipShow. In the shipShow method: • from the ArrayList, remove the CargoShip that was declared as type Ship. • add another WarShip instance to the ArrayList. • use a foreach loop to process the ArrayList and print the data for each ship. • count the number of ships that are not afloat. Return this count to main for printing.
In ShipTest: • make two objects with declared type Ship but actual type CruiseShip. • make one object of declared type Ship but actual type CargoShip. • make one object of declared type CargoShip and actual type CargoShip. • make a ship of declared type WarShip and actual type WarShip. • using the array initializer syntax, code all of these ships into an array of type Ship named fleet. • code a for loop that processes the fleet array to show display all ships and their data. • use a method of class Arrays to create an ArrayList of type Ship from the fleet array. • pass this ArrayList to a method named shipShow that also displays all ships and returns an integer. • report the value of the integer returned by shipShow. In the shipShow method: • from the ArrayList, remove the CargoShip that was declared as type Ship. • add another WarShip instance to the ArrayList. • use a foreach loop to process the ArrayList and print the data for each ship. • count the number of ships that are not afloat. Return this count to main for printing.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help with this assignment. Need to create 4 classes and need to have the output as specified.
See images.
Thank you!

Transcribed Image Text:<<Java Class>>
Cruise Ship
chap11new
passengers: int
azone: String
Cruise Ship(String,int, boolean,int, String)
getPassengers():int
getZone(): String
toString(): String
<<Java Class>>
Ship
chap11new
name: String
year: int
isAfloat: boolean
Ship(String,int, boolean)
isAfloat():boolean
●setAfloat(boolean):void
●getName(): String
●get Year():int
toString(): String
<<Java Class>>
WarShip
chap11new
type: String
nation: String
WarShip(String,int, boolean, String, String)
toString(): String
<<Java Class>>
CargoShip
chap11new
a cargo: String
capacity: int
CargoShip(String,int, boolean, String.int)
toString(): String
getCapacity:int

Transcribed Image Text:Create the four data classes as above. Do NOT add any attributes or methods that don't appear in the UML diagrams. Create another, executable class named
ShipTest as shown below to test these classes.
In ShipTest:
• make two objects with declared type Ship but actual type CruiseShip.
• make one object of declared type Ship but actual type CargoShip.
• make one object of declared type CargoShip and actual type CargoShip.
• make a ship of declared type WarShip and actual type WarShip.
• using the array initializer syntax, code all of these ships into an array of type Ship named fleet.
•
code a for loop that processes the fleet array to show display all ships and their data.
• use a method of class Arrays to create an ArrayList of type Ship from the fleet array.
• pass this ArrayList to a method named shipShow that also displays all ships and returns an integer.
• report the value of the integer returned by shipShow.
In the shipShow method:
• from the ArrayList, remove the CargoShip that was declared as type Ship.
• add another WarShip instance to the ArrayList.
• use a foreach loop to process the ArrayList and print the data for each ship.
• count the number of ships that are not afloat. Return this count to main for printing.
SAMPLE OUTPUT
AN ARRAY OF SHIPS IN MAIN
Ship name: Magic, year launched: 1998, is afloat: true
2700 passenger capacity, operating in the Caribbean
Ship name: Titanic, year launched: 1912, is afloat: false
1300 passenger capacity, operating in the Atlantic Ocean
Ship name: El Faro, year launched: 1974, is afloat: false
Capacity of containers is 391
Ship name: Seawise Giant, year launched: 1979, is afloat: false
Capacity of crude oil is 564763
Ship name: USS Nimitz, year launched: 1972, is afloat: true
Type: super carrier, operated by United States Navy
ARRAYLIST OF SHIPS FROM A METHOD
Ship name: Magic, year launched: 1998, is afloat: true
2700 passenger capacity, operating in the Caribbean
Ship name: Titanic, year launched: 1912, is afloat: false
1300 passenger capacity, operating in the Atlantic Ocean
Ship name: Seawise Giant, year launched: 1979, is afloat: false
Capacity of crude oil is 564763
Ship name: USS Nimitz, year launched: 1972, is afloat: true
Type: super carrier, operated by United States Navy
Ship name: USS John Warner, year launched: 2015, is afloat: true
Type: attack submarine, operated by United States Navy
2 of these ships sank!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
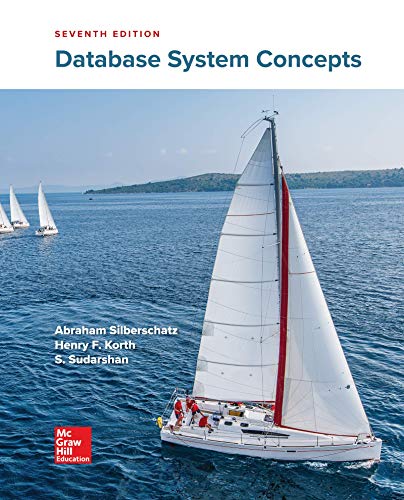
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
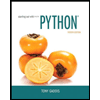
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
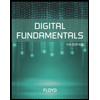
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
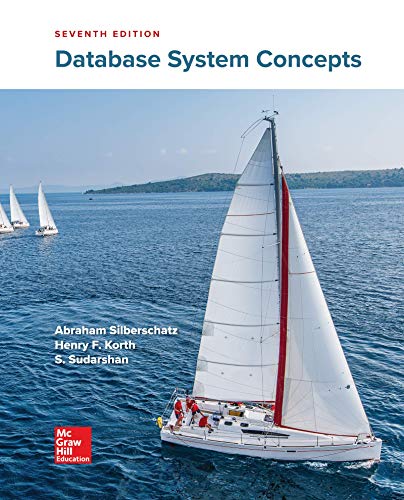
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
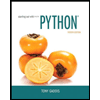
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
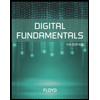
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
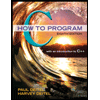
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
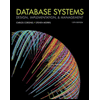
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
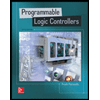
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education