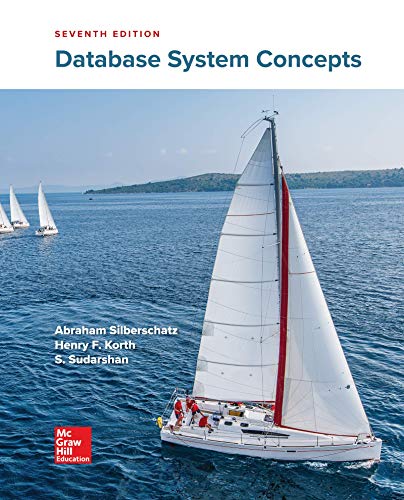
In python. Please do not copy elsewhere as it is all incorrect, and include docstring so that I can understand the information. Thanks.
import the **json** module.
Write a class named SatData that reads a [JSON file containing data on 2010 SAT results for New York City](https://data.cityofnewyork.us/api/views/zt9s-n5aj/rows.json?accessType=DOWNLOAD) and writes the data to a text file in CSV format. That same JSON file will be provided as a local file in Gradescope named **sat.json**. Your code does not need to access the internet.
CSV is a very simple format - just commas separating columns and newlines separating rows (see note below about commas that are part of field names). You'll see an example of CSV format below. There is a csv module for Python, but you will not use it for this project.
Your class should have:
* an init method that reads the file and stores it in whatever data member(s) you prefer. Any data members of the SatData class must be **private**.
* a method named **save_as_csv** that takes as a parameter a list of DBNs (district bureau numbers) and saves a CSV file that looks like [this](https://data.cityofnewyork.us/api/views/zt9s-n5aj/rows.csv?accessType=DOWNLOAD), but with only the rows that correspond to the DBNs in the list (and also the row of column headers). **To see what CSV syntax looks like, open the file as a text file rather than as a spreadsheet.** You may assume that all of the DBNs in the list passed to your method are present in the JSON file. The rows in the CSV file must be sorted in ascending order by DBN. The name of the output file must be **output.csv**.
For example, your class could be used like this:
```
sd = SatData()
dbns = ["02M303", "02M294", "01M450", "02M418"]
sd.save_as_csv(dbns)
```
You may hardcode the column headers instead of having to read them from the JSON file.
Some of the school names contain one or more commas. How should you preserve such a name as a single field in CSV format, since commas normally separate fields? The correct way to handle that is to enclose such names in double quotes in your CSV file. If you open up the example CSV file in a text editor, you can see that's what it does.
Don't let the CSV part intimidate you. You're just taking the values for a row, making them into a string that has commas between the values (and with double quotes around the values that contain commas), and then writing those strings to a file.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- A client with a completed main function is provided. The main function should not be modified. You must update the client by creating and implementing the various functions described below. readFile – Loads the parameter array with Billionaire objects. The data for each Billionaire is read from the given data file which is in CSV format (comma delimited - 1 line per record with fields separated by a comma.) The function should read each line from the file, pass the line read to the Billionaire class constructor and store the resulting object in the parameter array. The second parameter represents the maximum number of Billionaire objects that can be stored. displayAll - Displays a list of the Billionaires stored in the array. getRange – Determines the smallest wealth value and the largest wealth value within the array. These values are returned to the caller via reference parameters. getWealthiest – Returns the Billionaire within the array with the greatest wealth. getUS –…arrow_forwardNo written by hand solutionarrow_forwardcodearrow_forward
- only Voicemail.java files can have input added inbetween lines 4 and 7. not in the gery code sections.arrow_forwardI am trying to read a CSV file and then store it into an ArrayList. For each row, I'm trying to create a new Country class instance and add it to the list. However, it is giving me the following as the output: edu.uga.cs1302.quiz.Country@7c9bb6b8 edu.uga.cs1302.quiz.Country@441847 edu.uga.cs1302.quiz.Country@2621fba7 edu.uga.cs1302.quiz.Country@4fa6e7a1 edu.uga.cs1302.quiz.Country@6e29b69b edu.uga.cs1302.quiz.Country@4e0f0d39 edu.uga.cs1302.quiz.Country@67da3b9c edu.uga.cs1302.quiz.Country@1f394329 edu.uga.cs1302.quiz.Country@3c07b33b edu.uga.cs1302.quiz.Country@a570747 edu.uga.cs1302.quiz.Country@3ce7cb4a edu.uga.cs1302.quiz.Country@6912e7f4 edu.uga.cs1302.quiz.Country@680d0f86 edu.uga.cs1302.quiz.Country@5a5250ff edu.uga.cs1302.quiz.Country@58ed7d64 edu.uga.cs1302.quiz.Country@26be1cca edu.uga.cs1302.quiz.Country@26cf56a4 edu.uga.cs1302.quiz.Country@6ed22f2a edu.uga.cs1302.quiz.Country@5de769c9 edu.uga.cs1302.quiz.Country@b6966f3 edu.uga.cs1302.quiz.Country@574f6b4c…arrow_forwardUse the "College" data set from the package "ISLR", do the following: Get familiar with the data set. How many variables the data set has? What is the class of the variable "Private"? Add a new column "Enroll.rate" which is the ratio of Enroll v.s. Accept, to the data set. What are the top 10 Universities in terms of "Enroll.rate" (highest student enrollment ratio)? Get a new data frame "Mysubset" with all rows with an "Outstate" fee between 8000-9000 USD. solve using r codearrow_forward
- Please help me with my code in python. Can you change the start_tag and end_tag to strip. Since we have not discussed anything about tag yet. Thank you def read_data(): with open("simple.xml", "r") as file: content = file.read() return content def extract_data(tag, string): data = [] start_tag = f"<{tag}>" end_tag = f"</{tag}>" while start_tag in string: start_index = string.find(start_tag) + len(start_tag) end_index = string.find(end_tag) value = string[start_index:end_index] data.append(value) string = string[end_index + len(end_tag):] return data def get_names(string): names = extract_data("name", string) return names def get_calories(string): calories = extract_data("calories", string) return calories def get_descriptions(string): descriptions = extract_data("description", string) return descriptions def get_prices(string): prices = extract_data("price", string) return prices def…arrow_forwardWrite a complete program that sorts dword unsigned integer array in descending order. Assume that the user doesn’t enter more than 40 integers. You MUST use the template-1-2.asm Download template-1-2.asm and follow all the directions there. Note: you have to review 3 peer assignments. You can’t add any more procedures to the template. The procedures can’t use any global variables (variables that are inside .data segment). The caller of any procedures sends its argument through the stack. Inside any procedures, if you need to use a register, you have to preserve its original value. You can't use uses, pushad operators. The callee is in charge of cleaning the stack Sample run: Enter up to 40 unsigned dword integers. To end the array, enter 0. After each element press enter: 1 4 3 8 99 76 34 5 2 17 0 Initial array: 1 4 3 8 99 76 34 5 2 17 Array sorted in descending order: 99 76 34 17 8 5 4 3 2 1 template-1-2.asm include irvine32.inc ;…arrow_forwardThe shape class variable is used to save the shape of the forestfire_df dataframe. Save the result to ff_shape. # TODO 1.1 ff_shape = print(f'The forest fire dataset shape is: {ff_shape}') todo_check([ (ff_shape == (517,13),'The shape recieved for ff_shape did not match the shape (517, 13)') ])arrow_forward
- Complete the docstring using the information attached (images):def upgrade_stations(threshold: int, num_bikes: int, stations: List["Station"]) -> int: """Modify each station in stations that has a capacity that is less than threshold by adding num_bikes to the capacity and bikes available counts. Modify each station at most once. Return the total number of bikes that were added to the bike share network. Precondition: num_bikes >= 0 >>> handout_copy = [HANDOUT_STATIONS[0][:], HANDOUT_STATIONS[1][:]] >>> upgrade_stations(25, 5, handout_copy) 5 >>> handout_copy[0] == HANDOUT_STATIONS[0] True >>> handout_copy[1] == [7001, 'Lower Jarvis St SMART / The Esplanade', \ 43.647992, -79.370907, 20, 10, 10] True """arrow_forwardPlease make sure to follow what is given!!!arrow_forwardThank you very much for explaining the absyract concept, it was very helpful and easier to understanc after you explained it. Today we are asked to modify the GridWriter class by adding additional collection style functionality, to throw an exception in case of an error. The GridWriter class should get two new methods: public int size() should return the number of GridItems stored in the GridWriter public GridItem get(int index) should return the stored GridItems by index. Consider the following code. The first line creates a GridWriter object. Then two items are added to the GridWriter. The index of the items will be 0, and 1. Notice how the for loop uses the size and get methods to print out the areas of the two items GridWriter gw = new GridWriter(40, 50); gw.add(new MyCircle(10, 10, 9)); gw.add(new MyRectangle(40, 0, 10, 10)); for (int i = 0; i < gw.size(); i++) { System.out.println(gw.get(i).getArea()); } Once you have these two methods working you…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
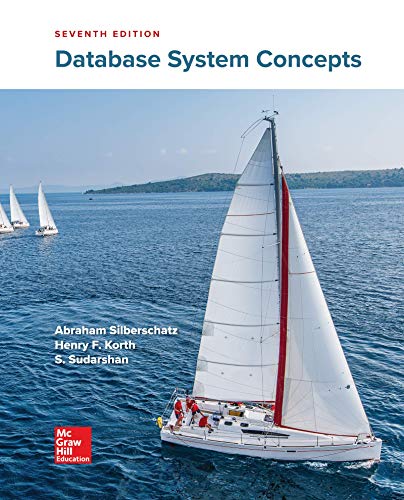
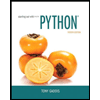
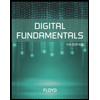
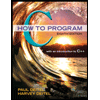
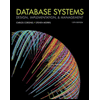
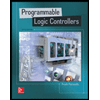