In Python: Using class Point that you implemented in assignment 9, implement a class called ThreeDPoint that inherits class Point. The class has (other than the inherited x, and y) another integer named z. Class ThreeDPoint has the following: Default initializer, used to create objects of type ThreeDPoint, by default x, y, and z are set to 1. initializer with three parameters for x, y, and z. set and get methods for z. Override Method __str__, which returns a string that represent the ThreeDPoint (e.g., p = [4,5, 6]).
In Python: Using class Point that you implemented in assignment 9, implement a class called ThreeDPoint that inherits class Point. The class has (other than the inherited x, and y) another integer named z. Class ThreeDPoint has the following: Default initializer, used to create objects of type ThreeDPoint, by default x, y, and z are set to 1. initializer with three parameters for x, y, and z. set and get methods for z. Override Method __str__, which returns a string that represent the ThreeDPoint (e.g., p = [4,5, 6]).
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter10: Object-oriented Programming
Section: Chapter Questions
Problem 16RQ
Related questions
Question
In Python:
Using class Point that you implemented in assignment 9, implement a class called ThreeDPoint that inherits class Point. The class has (other than the inherited x, and y) another integer named z. Class ThreeDPoint has the following:
- Default initializer, used to create objects of type ThreeDPoint, by default x, y, and z are set to 1.
- initializer with three parameters for x, y, and z.
- set and get methods for z.
- Override Method __str__, which returns a string that represent the ThreeDPoint (e.g., p = [4,5, 6]).
![#Implement a class called Point, the class contains two values of type integer (x and y). Define both as private. Add the following to the class:
# and get methods for x.
#Set and get methods for y.
#Method
str
which returns a string that represent the Point (e.g., p = [4,5]).
class Point:
# parameterized constructor
(self, x=1, y=1):
def
init
self.
X
self.
# setter for private member x
def set x (self, x):
self.
X = X
# getter for private member x
def get_x(self):
return self.
X
# setter for private member y
def set y (self, y):
self._y = y
# getter for private member y
def get y (self):
return self.
_y
# string representation
(self):
def
str
return "[{},{}]".format(self. x, self. y)
if
name
main
Point ()
p =
print(p)
p.set_x(4)
p.set_y(5)
print(p)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7983954-7793-415b-9e4b-01bf8f0007b8%2F7a57b586-4518-4224-be7f-78c5f2da369e%2Fqpte5fd_processed.png&w=3840&q=75)
Transcribed Image Text:#Implement a class called Point, the class contains two values of type integer (x and y). Define both as private. Add the following to the class:
# and get methods for x.
#Set and get methods for y.
#Method
str
which returns a string that represent the Point (e.g., p = [4,5]).
class Point:
# parameterized constructor
(self, x=1, y=1):
def
init
self.
X
self.
# setter for private member x
def set x (self, x):
self.
X = X
# getter for private member x
def get_x(self):
return self.
X
# setter for private member y
def set y (self, y):
self._y = y
# getter for private member y
def get y (self):
return self.
_y
# string representation
(self):
def
str
return "[{},{}]".format(self. x, self. y)
if
name
main
Point ()
p =
print(p)
p.set_x(4)
p.set_y(5)
print(p)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
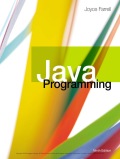
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
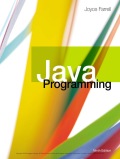
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT