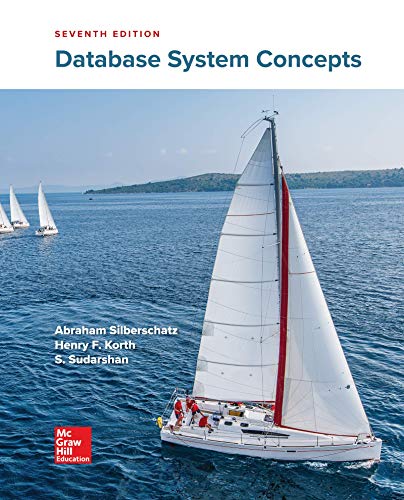
Concept explainers
In pyhton please
Explore a specific way to delete the root node of
the Binary Search Tree (BST) while maintaining the Binary Search Tree
(BST) property after deletion.
Implementation will be as stated below:
[1] Delete the root node value of the BST and replace the root value with
the appropriate value of the existing BST .
[2] Perform the BST status check by doing an In-Order Traversal of the
BST such that even after deletion the BST is maintained.
# Class to represent Tree node
class Node:
# A function to create a new node
def __init__(self, key):
self.data = key
self.left = None
self.right = None
The root element of the Binary Search Tree is given to you. Below is an illustrated sample of Binary Search Tree nodes for your reference, which in-fact is
the same example we discussed in the lecture.
root = Node(4)
root.left = Node(2)
root.right = Node(6)
root.left.left = Node(1)
root.left.right = Node(3)
root.right.left = Node(5)
root.right.right = Node(7)
Delete the root node value provided and replace the root value
with appropriate value such that the BST property is maintaned which would
be known by performing the In-Order Traversal of the new BST. Obviously, the
In-order traversal of the resulting BST would need to be ascending order
When you follow the deletion process and the BST traversal specified - the complexity of the solution will be as below.
Time Complexity: O(n)
Space Complexity: O(n)

Step by stepSolved in 3 steps with 1 images

- Implement a priority queue using a heapordered binary tree, but use a triply linked structure instead of an array. You will needthree links per node: two to traverse down the tree and one to traverse up the tree. Yourimplementation should guarantee logarithmic running time per operation, even if nomaximum priority-queue size is known ahead of time.arrow_forwardPlease answer the following section.arrow_forwardEstablish a linked queue, and run operations in the linked queue. General operations include:Create queue, one element enqueue, one element dequeue, check if the queue is full. Implementation of algorithm Header file typedef int DataType; typedef struct Node { DataType data; struct Node *next; }Lnode; //define node type typedef struct Qu { Lnode *front; Lnode *rear; } Queue;//queue type Source file #include <stdio.h> #include <stdlib.h> #include <string.h> #include <malloc.h> #include "queue.h" Queue * Initiate_queue()//Initial queue with head node, return pointer of the queue. { } int Queue_empty (Queue *queue)//Check if the queue if empty. If it is empty return 1 else return0. { } void En_queue(Queue *queue, DataType node)//Enqueue { } DataType De_queue(Queue *queue)//Dequeue a node, return its value. { Lnode *de_node;…arrow_forward
- The following code implementation for an inorder traversal has a "visit" function as a parameter. I do not know how it works and what ways it plays a role in the traversal of the linked list in a binary tree.arrow_forwardWrite The pseudo-code for:- 1. Create a leaf node for each symbol and add it to the priority queue.2. While there is more than one node in the queue:- Remove the two nodes of highest priority from the queue.- Create a new internal node with these two nodes as children and with frequency equal to the sum ofthe two nodes' frequency.- Add the new node to the queue.3. The remaining node is the root node and the Huffman tree is completearrow_forwarda) Given a typical Queue q with elements [E,D,C,B,A] (where Front/left of Queue at E and Rear/right of Queue at A), determine and list elements of the updated Queue in the similar form (Front/left and Rear/right), after the following execution: q.enqueue( q. dequeue() ) q. enqueue( G ) q.dequeue() b) Given a typical Stack s with elements [T,W,X,Y,Z] (where Top/left of Stack at T) and a typical Queue q with elements [E,D,C,B,A] (where Front/left of Queue at E and Rear/right of Queue at A), determine and list elements of the updated Stack and those of the updated Queue in their similar forms after the following execution: q. enqueue( s.pop() ) s.push( R ) q.enqueue( s. peek () ) s. push( q.dequeue() ) q.enqueue( s. pop() ) OParrow_forward
- When presenting a menu option to the user in the main cpp file. Asking user to input in an ID, how do I code a search method implementation where it takes that ID and search it in the binary tree and either return true if found and false if not. I am trying to steer away from using item which is attached to both (ID, username). I am trying to only take the ID input and the node pointer and traversing through the binary tree to find if the ID matches any of the nodes.arrow_forwardInsert the following key values one by one into an empty AVL tree. Restore tree as needed during the process. After the tree is built, show the post order traversal result in terms of the order of the key values visited. 30 45 65 55 50 52arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
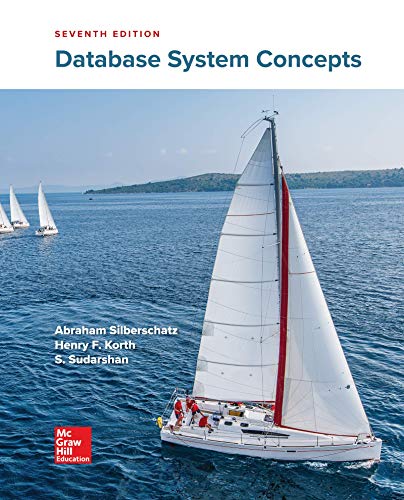
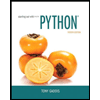
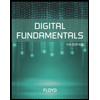
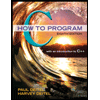
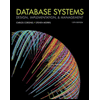
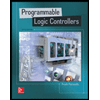