# In[ ]: |def minIndexAfter (items, startPos): minIndex = startPos i = startPos + 1 while (i < len (items)): if (items [i] < items[minIndex]): minIndex = i i = i + 1 return minIndex Define a function called selectionSort below. In the body of the function, you will need to call the 'swap function and the 'minlndexAfter' function. - Define 'selectionSort' here items = [395,2,-4,20,47,200,-50,-12] selectionSort (items) print (items)
# In[ ]: |def minIndexAfter (items, startPos): minIndex = startPos i = startPos + 1 while (i < len (items)): if (items [i] < items[minIndex]): minIndex = i i = i + 1 return minIndex Define a function called selectionSort below. In the body of the function, you will need to call the 'swap function and the 'minlndexAfter' function. - Define 'selectionSort' here items = [395,2,-4,20,47,200,-50,-12] selectionSort (items) print (items)
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18PE
Related questions
Question
![Run the code below to define the function
# In[ ]:
E def minIndexAfter (items, startPos) :
minIndex = startPos
i = startPos + 1
while (i < len (items)) :
if (items[i] < items[minIndex]):
minIndex = i
i = i + 1
return minIndex
Define a function called `selectionSort` below. In the body of the function, you will need to call the `swap
function and the `minlndexAfter` function.
- Define 'selectionSort' here
items = [395,2,-4,20, 47,200,-50,-12]
selectionSort (items)
print (items)
Use the code above to test your 'selectionSort' function](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa77530ed-50c9-4436-8e59-00fe5392100f%2Fd4cbbb71-7129-4e6b-9765-47534c1c0065%2Fltzbf68b_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Run the code below to define the function
# In[ ]:
E def minIndexAfter (items, startPos) :
minIndex = startPos
i = startPos + 1
while (i < len (items)) :
if (items[i] < items[minIndex]):
minIndex = i
i = i + 1
return minIndex
Define a function called `selectionSort` below. In the body of the function, you will need to call the `swap
function and the `minlndexAfter` function.
- Define 'selectionSort' here
items = [395,2,-4,20, 47,200,-50,-12]
selectionSort (items)
print (items)
Use the code above to test your 'selectionSort' function
![Run the code below to call the function
# In[ ]:
otherList = [395,2,-4,20,47,200,-50,-12] #a list for testing
print ('Before sorting: ', otherList)
bubbleSort (otherList)
print ('After sorting:', otherList)
a) What are the contents of otherList after a single iteration of the outer loop of 'bubbleSort?
b) How many iterations of the outer loop happen when sorting `otherList?
* Then run both blocks of code (the def block and the test block) again.
* Examine the output to answer the question about iterations.
Create a new list below with * elements known as "yourList" - a list where you can get 'bubbleSort` to stop
early (do fewer iterations). How many times did the outer loop run for 'yourList?
# In[ ]:
yourList = [] #fill in this list with 8 elements separated by commas
print ('Before sorting:', yourList)
bubbleSort (yourList)
print ('After sorting:', yourList)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa77530ed-50c9-4436-8e59-00fe5392100f%2Fd4cbbb71-7129-4e6b-9765-47534c1c0065%2Fydp3x59_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Run the code below to call the function
# In[ ]:
otherList = [395,2,-4,20,47,200,-50,-12] #a list for testing
print ('Before sorting: ', otherList)
bubbleSort (otherList)
print ('After sorting:', otherList)
a) What are the contents of otherList after a single iteration of the outer loop of 'bubbleSort?
b) How many iterations of the outer loop happen when sorting `otherList?
* Then run both blocks of code (the def block and the test block) again.
* Examine the output to answer the question about iterations.
Create a new list below with * elements known as "yourList" - a list where you can get 'bubbleSort` to stop
early (do fewer iterations). How many times did the outer loop run for 'yourList?
# In[ ]:
yourList = [] #fill in this list with 8 elements separated by commas
print ('Before sorting:', yourList)
bubbleSort (yourList)
print ('After sorting:', yourList)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
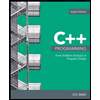
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
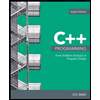
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning