In Lab 2, you created a menu for a simple calculator program. In this lab, you will add some functionality to the menu. Also, it is important to let the user know if they entered an invalid choice. Write a program (or modify the one written for Lab 2) that displays the following menu and prompts the user for a selection. Once the selection has been made, display the selection back to the user. Then, ask the user for two numbers and perform the selected operation (+, -, *, /). Display the results back to the user (e.g. 2 + 3 = 5). When dividing, display an error message if the second number (denominator) is 0. 1) Add two numbers 2) Subtract two numbers 3) Multiply two numbers 4) Divide two numbers 9) Exit program The program should: contain header comments as shown in class display a "hello" message before presenting the menu display the menu prompt the user for selection display the selection back to the user if the selection was invalid, display error message to user and then go to goodbye message if the selection was valid, prompt the user for two numbers if the operation is division, and the second number is 0, display error message to user and then go to goodbye message for any other scenario, perform the operation and display the results display a "goodbye" message before exiting the program HINT: The switch statement is ideal for menu-driven programs... SAMPLE OUTPUT FOR VALID MENU SELECTION You entered 2, subtract two numbers SAMPLE OUTPUT FOR INVALID MENU SELECTION You entered 5, an invalid selection SAMPLE OUTPUT FOR ADDPlease enter two numbers, separated by a space: 2 3 2 + 3 = 5 SAMPLE OUTPUT FOR DIVISION ERRORPlease enter two numbers, separated by a space: 2 0 Division by zero is not possible LANGUAGE IS C++
In Lab 2, you created a menu for a simple calculator
Write a program (or modify the one written for Lab 2) that displays the following menu and prompts the user for a selection. Once the selection has been made, display the selection back to the user. Then, ask the user for two numbers and perform the selected operation (+, -, *, /). Display the results back to the user (e.g. 2 + 3 = 5). When dividing, display an error message if the second number (denominator) is 0.
1) Add two numbers
2) Subtract two numbers
3) Multiply two numbers
4) Divide two numbers
9) Exit program
The program should:
- contain header comments as shown in class
- display a "hello" message before presenting the menu
- display the menu
- prompt the user for selection
- display the selection back to the user
- if the selection was invalid, display error message to user and then go to goodbye message
- if the selection was valid, prompt the user for two numbers
- if the operation is division, and the second number is 0, display error message to user and then go to goodbye message
- for any other scenario, perform the operation and display the results
- display a "goodbye" message before exiting the program
HINT: The switch statement is ideal for menu-driven programs...
SAMPLE OUTPUT FOR VALID MENU SELECTION
You entered 2, subtract two numbers
SAMPLE OUTPUT FOR INVALID MENU SELECTION
You entered 5, an invalid selection
SAMPLE OUTPUT FOR ADDPlease enter two numbers, separated by a space: 2 3
2 + 3 = 5
SAMPLE OUTPUT FOR DIVISION ERRORPlease enter two numbers, separated by a space: 2 0
Division by zero is not possible
LANGUAGE IS C++

Trending now
This is a popular solution!
Step by step
Solved in 10 steps with 10 images

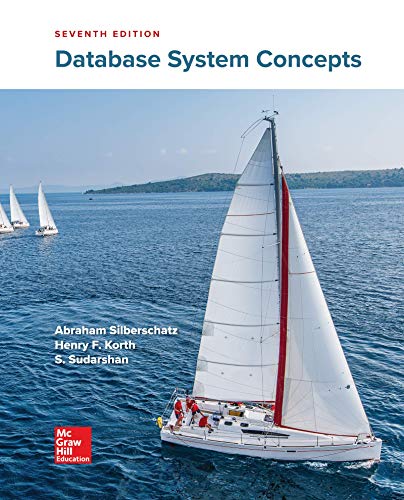
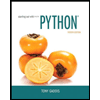
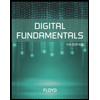
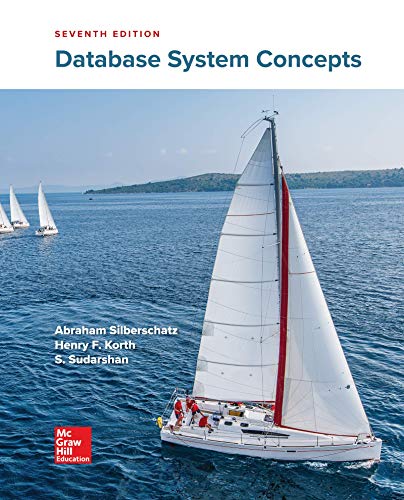
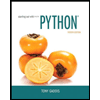
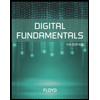
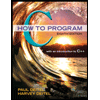
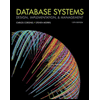
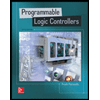