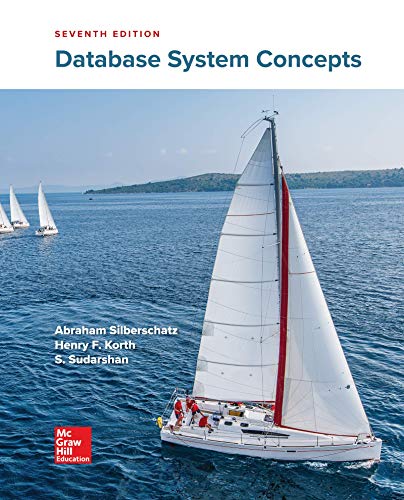
Concept explainers
In Java, what are the best practices for implementing the Comparable interface to ensure consistent and predictable sorting behavior across different scenarios?

You must utilise Java's Comparable interface to establish a natural ordering for the items in your class. You should abide by the following best practices and suggestions to provide predictable and consistent sorting behaviour in a variety of situations:
Train yourself to use the Comparable interface:
The compare To method must be replaced by the Comparable interface in your class. Depending on how the current object compares to the item being compared to, this function should either return a negative integer, zero, or a positive integer.
The following is a basic structure:
1public class YourClass implements Comparable<YourClass> {
2 // ...
3
4 @Override
5 public int compareTo(YourClass other) {
6 // Compare this object with 'other' and return:
7 // -1 if this < other
8 // 0 if this == other
9 // 1 if this > other
10 }
11}
12
Step by stepSolved in 3 steps

- Discuss the role of dynamic dispatch or late binding in method overriding and its impact on runtime behavior in object-oriented languages.arrow_forwardExplore the challenges and benefits of method overriding in multithreaded and concurrent programming.arrow_forwardExplain why it is easier to share a reentrant module using segmentation than it is to do so when pure paging is used.arrow_forward
- How does a decimal value waste memory space? What are the arguments for and against Java's implicit heap storagerecovery compared with the explicit heap storage recovery required in C++? Consider real-timesystems.arrow_forwardWhat is significance of the comparable interface in object sorting?arrow_forwardPlease give a detailed answer to the questions below along with code examples.What is a singleton class in Java?What is multi-threading?Difference between the final and finally keywords?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
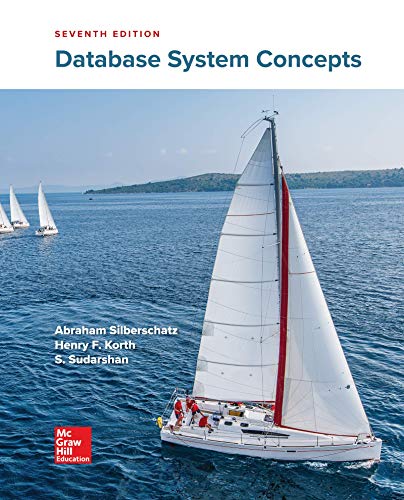
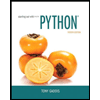
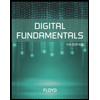
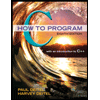
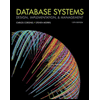
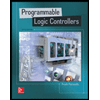