In Java The following is a java code for an old word puzzle: “Name a common word, besides tremendous, stupendous and horrendous, that ends in dous.” If you think about this for a while, it will probably come to you. However, we can also solve this puzzle by reading a text file of English words and outputting the word if it contains “dous” at the end. The text file “words.txt” contains 87314 English words, including the word that completes the puzzle. This file is available on Moodle. Your job is to insert try-catch statement in the below code, such that you surround the “risky” API with an appropriate exception handler --Edit the code if you have to! // import IO and util packages. public class WordPuzzle { public static void main(String[] args) { String s; Scanner scanner = new Scanner(Paths.get("words.txt")); while (scanner.hasNext()) { String word = scanner.next(); if (word.endsWith("dous")) System.out.println(word); } // end while } // end main } // end class
In Java
The following is a java code for an old word puzzle: “Name a common word, besides tremendous,
stupendous and horrendous, that ends in dous.” If you think about this for a while, it will probably
come to you. However, we can also solve this puzzle by reading a text file of English words and
outputting the word if it contains “dous” at the end. The text file “words.txt” contains 87314
English words, including the word that completes the puzzle. This file is available on Moodle.
Your job is to insert try-catch statement in the below code, such that you surround the “risky” API
with an appropriate exception handler --Edit the code if you have to!
// import IO and util packages.
public class WordPuzzle
{
public static void main(String[] args)
{
String s;
Scanner scanner = new Scanner(Paths.get("words.txt"));
while (scanner.hasNext())
{
String word = scanner.next();
if (word.endsWith("dous"))
System.out.println(word);
} // end while
} // end main
} // end class

Step by step
Solved in 3 steps with 1 images

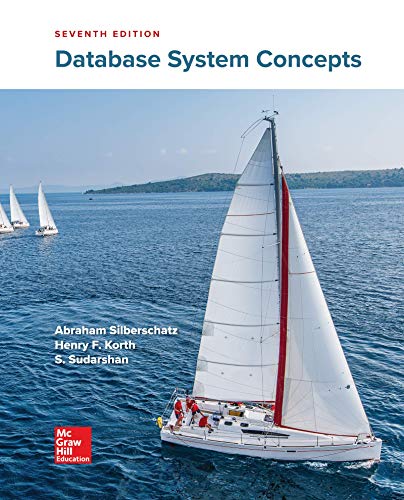
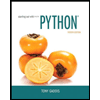
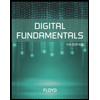
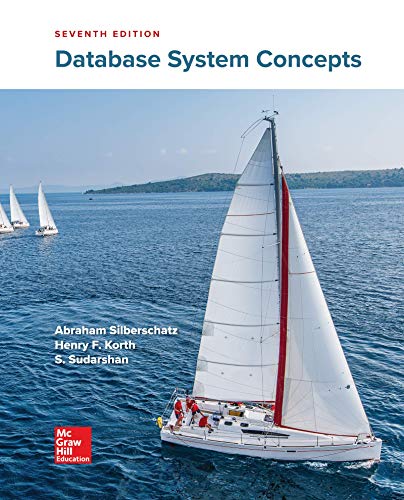
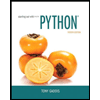
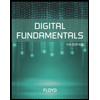
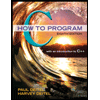
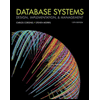
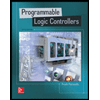