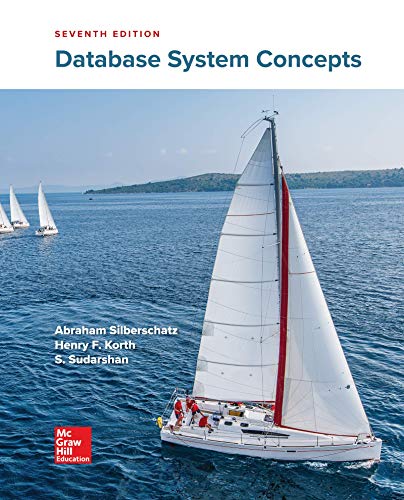
In java, Implement a method that removes all duplicates. Keep the first instance of the element but remove all subsequent duplicate elements.
public void removeDuplicates()
see the picture for the right output.
Given files:
Demo6.java
public class Demo6 {
public static void main(String[] args) {
MyLinkedList<String> list = new MyLinkedList<>();
TestBench.addToList(list);
TestBench.addToList(list);
TestBench.addToList(list);
TestBench.addToList(list);
for (int x = 0; x < list.size(); x += 1 + x/3) {
System.out.println("Removing element at index: " + x);
list.remove(x);
}
System.out.println("Result");
System.out.println(list);
System.out.println("Shuffling");
list.shuffle(7777);
System.out.println(list);
System.out.println("Removing duplicates (keeping first instance)");
list.removeDuplicates();
System.out.println(list);
}
}
TestBench.java
import java.util.AbstractList;
public class TestBench {
public static AbstractList<String> buildList() {
return addToList(new MyLinkedList<>());
}
public static AbstractList<String> addToList(AbstractList<String> list) {
String data = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
for (int x = 0; x < data.length(); x++) {
list.add(data.charAt(x) + "");
}
return list;
}
public static void test(AbstractList<String> list) {
System.out.println("--- Beginning Tests ---");
System.out.println("No changes");
System.out.println(list);
System.out.println("Testing size()");
System.out.println(list.size());
System.out.println("Testing add(int index, E element)");
list.add(0, "AAA");
list.add(0, "BBB");
list.add(10, "CCC");
list.add(15, "DDD");
list.add(list.size() - 1, "EEE");
System.out.println(list);
System.out.println("Testing get(int index)");
System.out.println("Element at 0: " + list.get(0));
System.out.println("Element at 10: " + list.get(10));
System.out.println("Element at 20: " + list.get(20));
System.out.println("Element at 26: " + list.get(26));
System.out.println("Element at last position: " + list.get(list.size() - 1));
System.out.println("Testing remove(int index)");
System.out.println(list.remove(0));
System.out.println(list.remove(0));
System.out.println(list.remove(0));
System.out.println(list.remove(10));
System.out.println(list.remove(20));
System.out.println(list.remove(list.size() - 1));
System.out.println(list);
System.out.println("Testing set(int index, E element)");
list.set(0, "QQQ");
list.set(5, "WWW");
list.set(10, "EEE");
list.set(12, "RRR");
list.set(4, "TTT");
list.set(20, "TTT");
list.set(list.size() - 1, "YYY");
System.out.println(list);
System.out.println("Testing indexOf(Object o)");
System.out.println("indexOf QQQ: " + list.indexOf("QQQ"));
System.out.println("indexOf WWW: " + list.indexOf("WWW"));
System.out.println("indexOf D: " + list.indexOf("D"));
System.out.println("indexOf HELLO: " + list.indexOf("HELLO"));
System.out.println("indexOf RRR: " + list.indexOf("RRR"));
System.out.println("indexOf TTT: " + list.indexOf("TTT"));
System.out.println("indexOf GOODBYE: " + list.indexOf("GOODBYE"));
System.out.println("Testing lastIndexOf(Object o)");
System.out.println("lastIndexOf QQQ: " + list.lastIndexOf("QQQ"));
System.out.println("lastIndexOf WWW: " + list.lastIndexOf("WWW"));
System.out.println("lastIndexOf D: " + list.lastIndexOf("D"));
System.out.println("lastIndexOf HELLO: " + list.lastIndexOf("HELLO"));
System.out.println("lastIndexOf RRR: " + list.lastIndexOf("RRR"));
System.out.println("lastIndexOf TTT: " + list.lastIndexOf("TTT"));
System.out.println("lastIndexOf GOODBYE: " + list.lastIndexOf("GOODBYE"));
System.out.println("Testing clear()");
list.clear();
System.out.println(list);
System.out.println("Testing clear() [second time]");
list.clear();
System.out.println(list);
System.out.println("Refilling the list");
addToList(list);
System.out.println(list);
System.out.println("--- Ending Tests ---");
}
}
![Test Case 1
Removing element at index: 0 \n
Removing element at index: 1
Removing element at index: 2 \n
Removing element at index: 3 \n
Removing element at index: 5
Removing element at index: 7 r
Removing element at index: 10 \n
Removing element at index: 14 \n
Removing element at index: 19 \n
Removing element at index: 26
Removing element at index: 35
Removing element at index: 47
Removing element at index: 63
Removing element at index: 85
Result n
[в, D, F, н, І, к, L, N, о, Р, R, S, т, U, w, х, Y, z, A, с, D, Е, F, G, н, I, к, L, м, N, о, р, 0, R, S, U, V, W, х, у, z, А, в, с, D, Е, F, н, I, 3, к, L, м, N, о, Р, Q. R, 5, т, U, V, w, Y, z, A, в, с, D, Е, F, G, н, I, J, к, L, м, N, о, Р, Q, R.
5, т, V, W, х, Ү, Z]|n
Shuffling n
[Q, L, к, W, с, о, R, F, м, в, F, S, z, н, D, т, V, R, T, G, N, L, т, о, Z, U, Y, S, V, z, 0, х, н, Ү, D, W, N, A, Х, D, Y, D, F, в, I, А, о, Р, А, Е, І, Р, Р, F, L, E, G, H, Мм, 3, в, Ј, с, к, к, м, S, N, н, R, С, U, I, E, Y, к, и, N, Р, Ss, w, V, L,
R, Z, Q, N, о, I, х]|m|
Removing duplicates (keeping first instance) \n
[о, L, к, м, с, R, F, М, в, S, Z, н, D, т, V, G, N, о, U, Y, х, А, I, Р, Е, J] m](https://content.bartleby.com/qna-images/question/4e8a92e6-ccd1-4b67-b436-479a3fd34a6c/351bb5d3-0be4-4e5a-9fa6-142d10bd3b2b/p59rny_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 12 images

- import java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardFix the following method printEvenIndex so that it will print out the Integers at even indices of the passed-in ArrayList list. import java.util.*; public class Test1 { public static void printEvenIndex(ArrayList<Integer> list) { for (int i) { if (i % 2 == 1) { System.out.print(list.get(i) + ", "); } } } public static void main(String[] args) { //instantiate ArrayList and fill with Integers ArrayList<Integer> values = new ArrayList<Integer>(); int[] nums = {1, 5, 7, 9, -2, 3, 2}; for (int i = 0; i < nums.length; i ++) { values.add(nums[i]); } System.out.println("Expected Result:\t 1, 7, -2, 2,"); System.out.print("Your Result:\t\t "); printEvenIndex(values); } }arrow_forwardC. package Final; import java.util.HashSet; public class LLCycle_FE { public static void main(String[] args) { Node head = buildLL(); // Given the above linked list write the 2 methods below (removeDuplicates and showLL) System.out.printf("\n --------- "); // This method will remove any duplicate LL nodes (that is, with the same color) head = removeDuplicates( head ); showLL( head ); } **public static Node removeDuplicates(Node head) { return head; } ** private static void showLL(Node head) { // ToDo: Output the entire linked list } private static Node buildLL() { // Use this code to create your LL Node head = new Node("Red", null); Node n2 = new Node("Blue", null); head.next = n2; Node n3 = new Node("Green", null); n2.next = n3; Node n4 = new Node("Yellow", null); n3.next = n4; Node n5 = new…arrow_forward
- Please use the template provided below and make sure the output matches exactly. import java.util.Scanner;import java.util.ArrayList; public class PhotoLineups { // TODO: Write method to create and output all permutations of the list of names. public static void printAllPermutations(ArrayList<String> permList, ArrayList<String> nameList) { } public static void main(String[] args) { Scanner scnr = new Scanner(System.in); ArrayList<String> nameList = new ArrayList<String>(); ArrayList<String> permList = new ArrayList<String>(); String name; // TODO: Read in a list of names; stop when -1 is read. Then call recursive method. }}arrow_forwardThis codes not complie it can you fix the code can complie it? import java.util.ArrayList; public class Heap { void heapify(ArrayList hT, int i) { int size = hT.size(); int largest = i; int l = 2 * i + 1; int r = 2 * i + 2; if (l < size && hT.get(l) > hT.get(largest)) largest = l; if (r < size && hT.get(r) > hT.get(largest)) largest = r; if (largest != i) { int temp = hT.get(largest); hT.set(largest, hT.get(i)); hT.set(i, temp); heapify(hT, largest); } } void insert(ArrayList hT, int newNum) { int size = hT.size(); if (size == 0) { hT.add(newNum); } else { hT.add(newNum); for (int i = size / 2 - 1; i >= 0; i--) { heapify(hT, i); } } } void deleteNode(ArrayList hT, int num) { int size = hT.size(); int i; for (i = 0; i < size; i++) { if (num == hT.get(i)) break; } int temp = hT.get(i); hT.set(i,…arrow_forwardJAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forward
- Code debug JAVA import java.util.ArrayList;public class ArrayList {public static ArrayList<Integer> reverse(ArrayList<Integer> list) {for (int i = 0; i < list.size(); i++) {System.out.println(list);}public static ArrayList<Integer> getReverse(ArrayList<Integer> list){for (int index = 0; index < list.size() / 2; index++) {int temp = list.get(index);list.set(index, list.get(list.size() - index - 1));//swaplist.set(list.size() - index - 1, temp); //swap}return list;}}public static void main(String[] args) {ArrayList<Integer> list = new ArrayList<>();list.add(1);list.add(2);list.add(3);System.out.println("Original elements : " + list);System.out.println("Reversed elements : " + getReverse(list));}}arrow_forwardGiven main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInDescendingOrder() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 -1 the output is: 9 8 7 6 5 4 3 2 1 import java.util.Scanner; public class SortedList { public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num; num = scnr.nextInt(); while (num != -1) {// Insert into linked list in descending order curNode = new IntNode(num);intList.insertInDescendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forwardGiven main() and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insertInAscendingOrder() method that inserts a new IntNode into the IntList in ascending order. Ex. If the input is: 8 3 6 2 5 9 4 1 7 -1 the output is: 1 2 3 4 5 6 7 8 9 import java.util.Scanner; public class SortedList {public static void main (String[] args) {Scanner scnr = new Scanner(System.in);IntList intList = new IntList();IntNode curNode;int num;num = scnr.nextInt();// Read in until -1while (num != -1) {// Insert into linked listcurNode = new IntNode(num);intList.insertInAscendingOrder(curNode);num = scnr.nextInt();}intList.printIntList();}}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
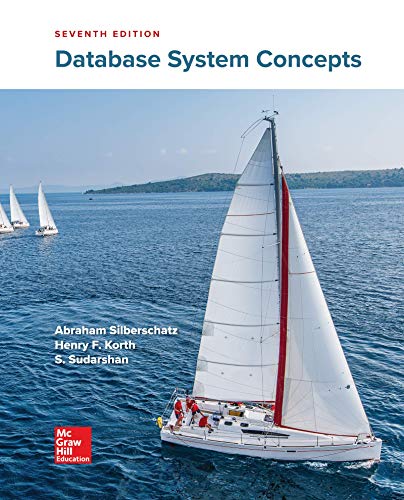
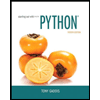
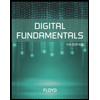
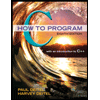
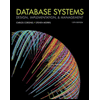
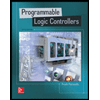