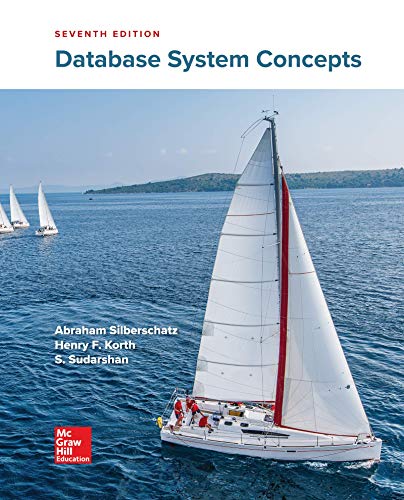
In c++
The Fibonacci numbers are a series of numbers that exhibit the following pattern:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, …
The series starts with 0 and 1, and each subsequent number in the series is the sum of the previous two numbers. The Fibonacci sequence, Fn, is defined by the following recurrence relation:
Fn = Fn-1 + Fn-2
Where the initial values are F0 = 0 and F1 = 1. Write a

The algorithm of the code:-
Step 1: Start
Step 2: Declare an integer a = 0, b = 1, c, and i.
Step 3: Check if N is less than or equal to 0, if yes return 0.
Step 4: Set the loop, for i = 2 to N do the following steps.
Step 5: Calculate the value of c = a + b.
Step 6: Set a = b.
Step 7: Set b = c.
Step 8: End loop.
Step 9: Return the value of b.
Step 10: Stop
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- C++arrow_forwardPython problem Problem statementDevelop a program that receives a matrix and determines whether it corresponds to a magic square. A magic square is one that the sum of its elements in each of the rows is equal to the sum of the elements in each of the columns and also equal to the sum of the elements of each of the diagonals. The condition that the numbers from 1 to n ** 2 are used must also be met, where n is the size of the magic square, which is a matrix of nxn. https://upload.wikimedia.org/wikipedia/commons/thumb/e/e4/Magicsquareexample.svg/1200px-Magicsquareexample.svg.png EntryThe first line corresponds to the number n. After this, n lines, each line contains a list of integers separated by a space. DepartureIf the square is magic, it must print the value that has the sum of the numbers by columns, rows and main diagonals. If it is not a magic square, the word "Error" must be printed without quotation marks. Examples Input Example 1 416 3 2 135 10 11 89 6 7 124 15 14 1Output…arrow_forwardrecursion using cpparrow_forward
- IN C PROGRAMMING LANGUAGE AND COMMENT EVERY LINE PLEASE SO I CAN UNDERSTAND EVERY STEP , The selection sort is one of several techniques for sorting an array. A selection sort compares every element of an array with all the other elements of the array and exchanges their values if they are out of order. After the first pass of a selection sort, the first array element is in the correct position; after the second pass the first two elements of the array are in the correct position, and so on. Thus, after each pass of a selection sort, the unsorted portion of the array contains one less element. Write and test a function that implements this sorting method.arrow_forwardNonearrow_forwardGiven a function uint16_t func(int16_t x) write a function callPassedFunc() that takes two arguments: • a pointer to func • a int16_t and returns a uint16_t. A call to callPassed Func() should have the same effect and return value as a call to func(), as in the example. Note that it should not matter what func() does. To test this question, write a few random function bodies for func(). For example: Test uint16_t func(int16_t x) { return x/2; //Only an example could be anything. } int16_t arg = 10; uint16_t directRetVal= func(arg); uint16_t indirect RetVal = callPassed Func(&func, arg); printf("%d\n", directRetVal == indirectRetVal); Result 1arrow_forward
- Genere Program Contexts for given statement: If a node n is traversed that corresponds to the pre-header node of a loop L, then function init prehender(n) is invoked which initializes the recurrence variables of L in context c_{b}(n) with the value of u.the folding operator (c, c1, c2 and cs are contexts)arrow_forwardbsfdb sdfd ddfvxcvarrow_forwardplease solve number C I solved numbers a and b so I do not need number a and b Problem (taken from page 308 of the textbook)A parking lot has 31 visitor spaces, numbered from 0 to 30. Visitors are assigned parking spaces usingthe hashing function h(k) = k mod 31, where k is the number formed from the first three digits on avisitor’s license plate.a) Which spaces are assigned by the hashing function to cars that have these first three digits ontheir license plates: 317, 918, 007, 100, 111, 310?b) Describe a procedure visitors should follow to find a free parking space when the space they areassigned is occupied.c) A large parking-systems company would like to automate your assignment procedure forselfparking cars. You have been hired to implement a ”simple” proof-of-concept program in C++, fornow customers will enter their 3 digit plate numbers and your software will assign a parkingspace. Your implementation should a find a free parking space if the original assigned space isoccupied.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
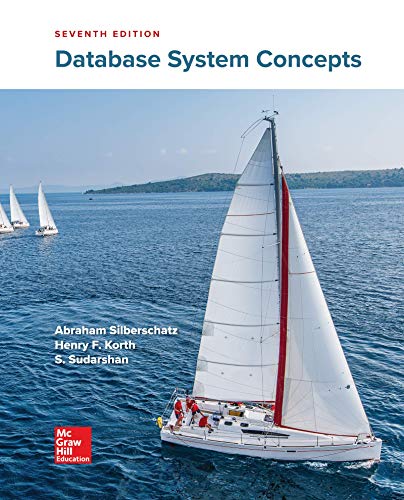
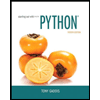
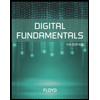
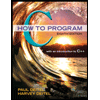
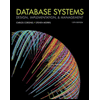
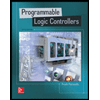