in C# Sometimes figuring letter grades is hard. Write a program that asks for, and reads from the user, a midterm exam grade and a final exam grade. Compute the average of those two grades and ouput a letter grade based on the scale shown below. CHALLENGE PROBLEM: One of my graduate school classes modified the above calculation. This professor said that the final grade would be determined by the simple average of the midterm and the final, however, if the student scored better on the final than they did on the midterm, the professor would weight the midterm exam at 25%, and the final exam at 75% when computing the final grade. Modify your code to use this professors modification in determining letter grade. Here is the grading scale:
in C#
Sometimes figuring letter grades is hard. Write a
and a final exam grade. Compute the average of those two grades and ouput a letter grade based on the scale shown
below. CHALLENGE PROBLEM: One of my graduate school classes modified the above calculation. This professor said
that the final grade would be determined by the simple average of the midterm and the final, however, if the student
scored better on the final than they did on the midterm, the professor would weight the midterm exam at 25%, and
the final exam at 75% when computing the final grade. Modify your code to use this professors modification in
determining letter grade. Here is the grading scale:


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

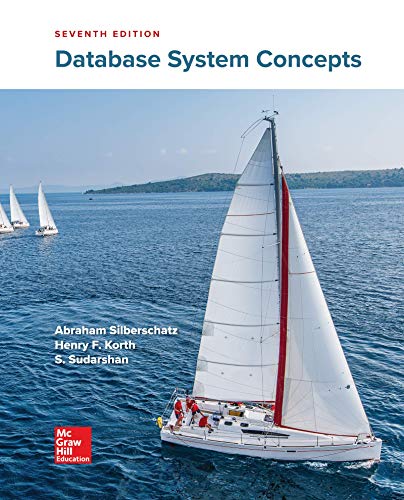
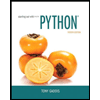
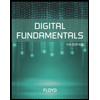
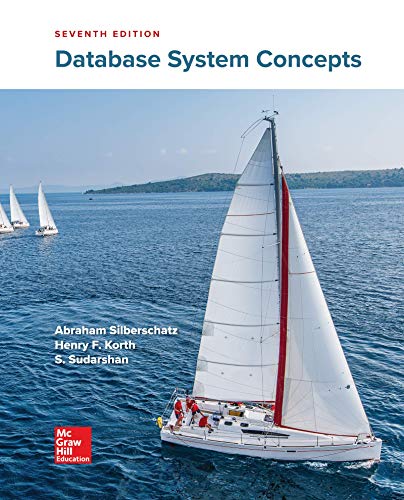
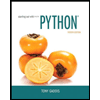
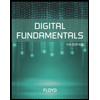
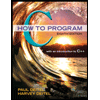
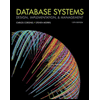
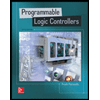