in c++ Modify “Producer and Consumer Problem” from the lecture note so that it can use all buffer space, not “buffersize – 1” as in the lecture note. This program should work as follows: 1. The user will run the program and will enter two numbers on the command line.Those numbers will be used for buffersize and counter limit.2. The main program will then create separate producer and consumer threads.3. The Producer thread generates a random number through a random number generator function, inserts it into the buffer, prints the number, and increment counter.4. The Consumer thread goes to the buffer, takes a number in the proper order, prints it out, and increment counter.5. After the counter reaches its limit, both threads should be terminated and returned to the main.6. Main program terminates.
in c++ Modify “Producer and Consumer Problem” from the lecture note so that it can use all buffer
space, not “buffersize – 1” as in the lecture note. This
1. The user will run the program and will enter two numbers on the command line.
Those numbers will be used for buffersize and counter limit.
2. The main program will then create separate producer and consumer threads.
3. The Producer thread generates a random number through a random number
generator function, inserts it into the buffer, prints the number, and increment
counter.
4. The Consumer thread goes to the buffer, takes a number in the proper order, prints it
out, and increment counter.
5. After the counter reaches its limit, both threads should be terminated and returned to
the main.
6. Main program terminates.

Step by step
Solved in 2 steps

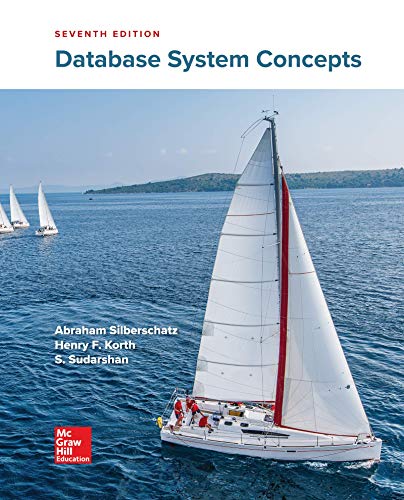
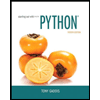
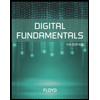
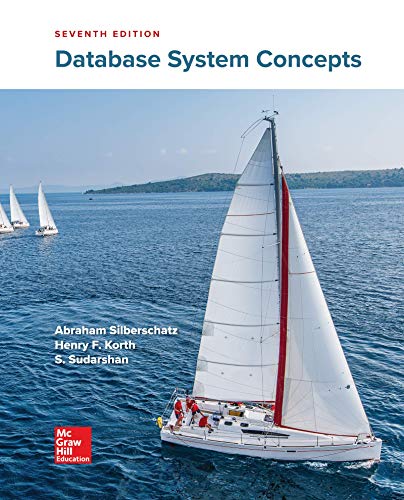
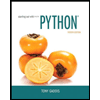
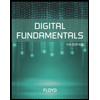
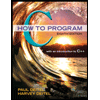
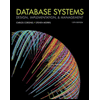
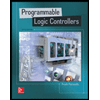