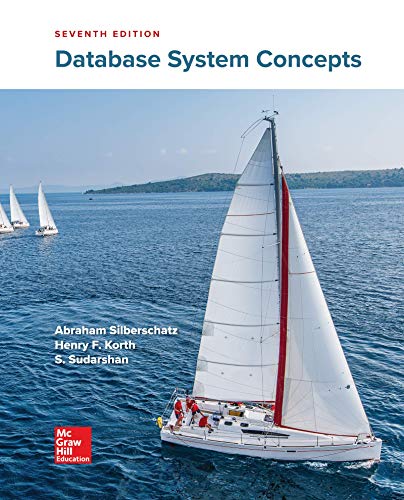
In c++, ask the user for the name of a file to store the information. Open the file, and make sure it opened
correctly.
Allow the user to process multiple records. Prompt the user at the end of each cycle whether they
want to process any more records. (Hint: what kind of a loop will you use that processes at least one
item?)
Then, for each item in the inventory, your
1. Ask the user for the product name (use getline so you can input a multi-word product name)
then read it in.
2. Ask the user for the wholesale price per item and read that in.
3. Ask the user for the quantity and read that in.
4. Calculate the total price paid.
5. Ask the user for the markup and read that in. (“Markup is usually expressed as a percentage,
and is the amount the price is raised to give a retail price).
6. Calculate the retail price.
That forms a “record”; all the information we know about this item.
Write the information to a file.
The function should print to the file, on two lines, the information in the following format:
Name on a line all by itself – that way we can have a string that contains multiple words.
On the second line, wholesale price, quantity, retail price, anticipated profit (total retail price for all
items – total wholesale price for all items). Separate your data fields with a space, no comma,
nothing more.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- In C++ I am opening a file through a command argument and reading through it. How do you write to the file that would be opened?arrow_forwardIf a file contains an index directory that allows for random access, what does it mean?arrow_forwardPython programming help I need help of question 1 to read a file from persons.txt Write a program that takes a person’s details (name, age and a city), and writes to a file (persons.txt) using a loop repeatedly. You should write three person’s to the file. The program terminates on entering any key by the user, except on (Y or y) key. Sample output of the first run of the program is shown below: Enter name: JohnEnter age: 20Enter city: SydneyData saved: John 20 SydneyPress (Y or y) to add another person, or any key to exit Question 1) Write a program that read a file (persons.txt) from previous question given on the top of this question, and shows all the records/lines from the file as a nicely formatted report with a header as shown: Name Age City +---------------------+--------------------+--------------arrow_forward
- In other words, describe the Pretest loop.arrow_forwardIn Python, Create a program that will write 100 integers created randomly in a file. The integers will be separated by a space in the file. Read the data back from the file, and display the sorted data. The program should prompt the user to enter a file name. Utilize the following function headers for this problem: Main() WriteNumbers(filename) ReadNumbers(filename) The main function will first prompt the user to enter the filename. Then the main function calls WriteNumbers-then ReadNumbers. The WriteNumbers function opens an output file and writes 100 random numbers as long a large string text. Do not use lists for this problem- please just write a random number followed by a space 100 times. The ReadNumbers function will then read the text file and display the numbers sorted. In order to sort the numbers, read the big string and then split it into a list. Now convert them into integers by using list comprehension and then sort the list. Loop through the list and print each…arrow_forwardSummary In this lab, you write a while loop that uses a sentinel value to control a loop in a Python program. You also write the statements that make up the body of the loop. Each theater patron enters a value from 0 to 4 indicating the number of stars that the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. Instructions Make sure the file MovieGuide.py is selected and open. Write thewhile loop using a sentinel value to control the loop, and also write the statements that make up the body of the loop. Execute the program by clicking the Run button at the bottom of the screen. Input the following as star ratings: 0, 3, 4, 4, 1, 1, 2, -1 must be written with this code: """ MovieGuide.py This program allows each theater patron to enter a value from 0 to 4 indicating the number of stars that the patron awards to the Guide's featured movie of the week. The program executes…arrow_forward
- The Springfork Amateur Golf Club has a tournament every weekend. The club president has asked you to write a program that will read each player's name and score as keyboard input, and then save these as records in a file named golf.txt . First, the program should ask the user for the number of players. Then, it should ask the user for each name and score individually. The file golf.txt should be structured so that there is a line with the player's name, followed by their score on the next line. Here is an example: Emily 30 Mike 20 Jonathan 23 Look carefully at the following sample run of the program. In particular, notice the wording of the messages and the placement of spaces and colons. Your program's output must match this. Sample Run (User input shown in bold) Enter number of players :4↵ Enter name of player number 1 : Jimmy↵ Enter score of player number 1 : 30↵ Enter name of player number 2 : Carly↵ Enter score…arrow_forwardThis is phython not Java Lab: Write a file copying program. The program asks for the name of the file to copy from (source file) and the name of the file to copy to (destination file). The program opens the source file for reading and the destination file for writing. As the program reads each line from the source file and it writes the line to the destination file. When every line from the source file has been written to the destination file, it close both files and print “Copy is successful.” In the sample run, “add.py” is the source file and “add-copy.py” is the destination file. Note that both “add-copy.py” is identical to “add.py” because “add-copy.py” is a copy of “add.py”. Sample run: Enter file to copy from: add.py Enter file to copy to : add-copy.py Copy is successful. Source file: add.py print("This program adds two numbers") a = int(input("Enter first number: ")) b = int(input("Enter second number: ")) print(f"{a} + {b} = {a+b}") Destination…arrow_forwardWrite a while loop that asks people why they like programming. Each time someone enters a reason, add their reason to a file that stores all the responses.arrow_forward
- With the program provided, apply it to create a simple student record system that does the following: 1. Displays a Menu (do.. while loop) with choices for user to choose from (similar to the one shown below) MENU Add a student to the record. Remove a student from the record. Display the whole record list. Modify the student record. Exit 2. Enables the user to choose the choice of action by typing the number between (1 to 5): 1) adding a student to the record; 2) removing a student from the record; 3) displaying the whole record; 4) modify the student record; 5) exit. The record contains students' full name, student number, and general weighted average. NOTE: All the code must be well commented per line. Must be a singly linked list. Use dynamic memory allocation. Use an array. Has the following C++ features: insert, delete, get full list, and empty list. (THESE ALL ARE REQUIRED)arrow_forward9b_act2. Please help me answer this in python programming.arrow_forwardWrite a C++ program that asks the user for a file name and a word for which to search. The program should search the file for every occurrence of the word. When the word is found, the line it contains should be displayed. After all of the occurrences have been found, the program should display the number of times the word was found in the file.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
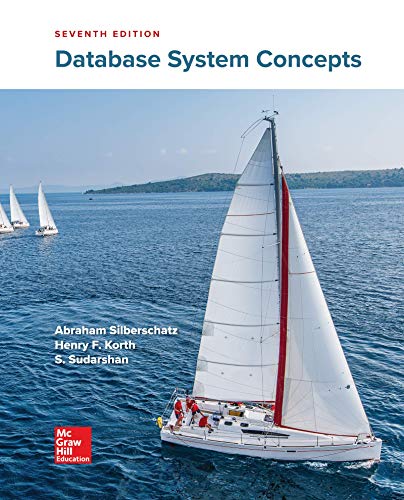
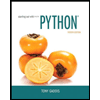
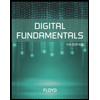
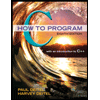
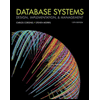
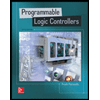