import sys import json from collections import deque with open('config.json', 'r') as config_file: config = json.load(config_file) # Define the process data class class Process: def __init__(self, name, runtime, arrival_time, io_frequency): self.name = name self.runtime = runtime self.arrival_time = arrival_time self.io_frequency = io_frequency self.remaining_time = runtime self.io_counter = 0 def shortest_job_first(processes, quantum): current_time = 0 queue = [] scheduled_order = [] io_queue = deque() while processes or queue or io_queue: # Add arriving processes to the queue while processes and processes[0].arrival_time <= current_time: queue.append(processes.pop(0)) if not queue and processes: current_time = processes[0].arrival_time queue.append(processes.pop(0)) if queue: # Find the process with the shortest remaining time min_remaining_time = min(p.remaining_time for p in queue) selected_process = next(p for p in queue if p.remaining_time == min_remaining_time) queue.remove(selected_process) # Check for I/O interrupt for the current process if selected_process.io_frequency > 0 and selected_process.io_counter == selected_process.io_frequency: scheduled_order.append('!' + selected_process.name) selected_process.io_counter = 0 io_queue.append(selected_process) else: scheduled_order.append(selected_process.name) # Check if the process can be completed within the quantum if selected_process.remaining_time <= quantum: current_time += selected_process.remaining_time selected_process.remaining_time = 0 else: current_time += quantum selected_process.remaining_time -= quantum selected_process.io_counter += 1 queue.append(selected_process) while io_queue: io_process = io_queue.popleft() queue.append(io_process) return scheduled_order def main(): # Check if the correct number of arguments is provided if len(sys.argv) != 2: return 1 # Extract the input file name from the command line arguments input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" # Define the number of processes num_processes = 0 # Initialize an empty list for process data data_set = [] # Open the file for reading try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print("Error opening the file.") return 1 quantum = 1 # Modify this value as per your requirements # Execute the shortest job first algorithm scheduled_order = shortest_job_first(data_set, quantum) # Generate output string based on the scheduled order output = ' '.join(scheduled_order) # Write the output to a file try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, "w") as output_file: output_file.write(output) except IOError: print("Error opening the output file.") return 1 return 0 if __name__ == "__main__": exit_code = main() sys.exit(exit_code) decrease the response and turnaround of this schedular
import sys import json from collections import deque with open('config.json', 'r') as config_file: config = json.load(config_file) # Define the process data class class Process: def __init__(self, name, runtime, arrival_time, io_frequency): self.name = name self.runtime = runtime self.arrival_time = arrival_time self.io_frequency = io_frequency self.remaining_time = runtime self.io_counter = 0 def shortest_job_first(processes, quantum): current_time = 0 queue = [] scheduled_order = [] io_queue = deque() while processes or queue or io_queue: # Add arriving processes to the queue while processes and processes[0].arrival_time <= current_time: queue.append(processes.pop(0)) if not queue and processes: current_time = processes[0].arrival_time queue.append(processes.pop(0)) if queue: # Find the process with the shortest remaining time min_remaining_time = min(p.remaining_time for p in queue) selected_process = next(p for p in queue if p.remaining_time == min_remaining_time) queue.remove(selected_process) # Check for I/O interrupt for the current process if selected_process.io_frequency > 0 and selected_process.io_counter == selected_process.io_frequency: scheduled_order.append('!' + selected_process.name) selected_process.io_counter = 0 io_queue.append(selected_process) else: scheduled_order.append(selected_process.name) # Check if the process can be completed within the quantum if selected_process.remaining_time <= quantum: current_time += selected_process.remaining_time selected_process.remaining_time = 0 else: current_time += quantum selected_process.remaining_time -= quantum selected_process.io_counter += 1 queue.append(selected_process) while io_queue: io_process = io_queue.popleft() queue.append(io_process) return scheduled_order def main(): # Check if the correct number of arguments is provided if len(sys.argv) != 2: return 1 # Extract the input file name from the command line arguments input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}" # Define the number of processes num_processes = 0 # Initialize an empty list for process data data_set = [] # Open the file for reading try: with open(input_file_name, "r") as file: # Read the number of processes from the file num_processes = int(file.readline().strip()) # Read process data from the file and populate the data_set list for _ in range(num_processes): line = file.readline().strip() name, duration, arrival_time, io_frequency = line.split(',') process = Process(name, int(duration), int(arrival_time), int(io_frequency)) data_set.append(process) except FileNotFoundError: print("Error opening the file.") return 1 quantum = 1 # Modify this value as per your requirements # Execute the shortest job first algorithm scheduled_order = shortest_job_first(data_set, quantum) # Generate output string based on the scheduled order output = ' '.join(scheduled_order) # Write the output to a file try: output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}" with open(output_path, "w") as output_file: output_file.write(output) except IOError: print("Error opening the output file.") return 1 return 0 if __name__ == "__main__": exit_code = main() sys.exit(exit_code) decrease the response and turnaround of this schedular
Chapter3: Data Representation
Section: Chapter Questions
Problem 3RP
Related questions
Question
import sys
import json
from collections import deque
with open('config.json', 'r') as config_file:
config = json.load(config_file)
# Define the process data class
class Process:
def __init__(self, name, runtime, arrival_time, io_frequency):
self.name = name
self.runtime = runtime
self.arrival_time = arrival_time
self.io_frequency = io_frequency
self.remaining_time = runtime
self.io_counter = 0
def shortest_job_first(processes, quantum):
current_time = 0
queue = []
scheduled_order = []
io_queue = deque()
while processes or queue or io_queue:
# Add arriving processes to the queue
while processes and processes[0].arrival_time <= current_time:
queue.append(processes.pop(0))
if not queue and processes:
current_time = processes[0].arrival_time
queue.append(processes.pop(0))
if queue:
# Find the process with the shortest remaining time
min_remaining_time = min(p.remaining_time for p in queue)
selected_process = next(p for p in queue if p.remaining_time == min_remaining_time)
queue.remove(selected_process)
# Check for I/O interrupt for the current process
if selected_process.io_frequency > 0 and selected_process.io_counter == selected_process.io_frequency:
scheduled_order.append('!' + selected_process.name)
selected_process.io_counter = 0
io_queue.append(selected_process)
else:
scheduled_order.append(selected_process.name)
# Check if the process can be completed within the quantum
if selected_process.remaining_time <= quantum:
current_time += selected_process.remaining_time
selected_process.remaining_time = 0
else:
current_time += quantum
selected_process.remaining_time -= quantum
selected_process.io_counter += 1
queue.append(selected_process)
while io_queue:
io_process = io_queue.popleft()
queue.append(io_process)
return scheduled_order
def main():
# Check if the correct number of arguments is provided
if len(sys.argv) != 2:
return 1
# Extract the input file name from the command line arguments
input_file_name = f"Process_List/{config['dataset']}/{sys.argv[1]}"
# Define the number of processes
num_processes = 0
# Initialize an empty list for process data
data_set = []
# Open the file for reading
try:
with open(input_file_name, "r") as file:
# Read the number of processes from the file
num_processes = int(file.readline().strip())
# Read process data from the file and populate the data_set list
for _ in range(num_processes):
line = file.readline().strip()
name, duration, arrival_time, io_frequency = line.split(',')
process = Process(name, int(duration), int(arrival_time), int(io_frequency))
data_set.append(process)
except FileNotFoundError:
print("Error opening the file.")
return 1
quantum = 1 # Modify this value as per your requirements
# Execute the shortest job first algorithm
scheduled_order = shortest_job_first(data_set, quantum)
# Generate output string based on the scheduled order
output = ' '.join(scheduled_order)
# Write the output to a file
try:
output_path = f"Schedulers/template/{config['dataset']}/template_out_{sys.argv[1].split('_')[1]}"
with open(output_path, "w") as output_file:
output_file.write(output)
except IOError:
print("Error opening the output file.")
return 1
return 0
if __name__ == "__main__":
exit_code = main()
sys.exit(exit_code)
decrease the response and turnaround of this schedular
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
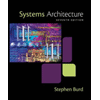
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
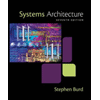
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning