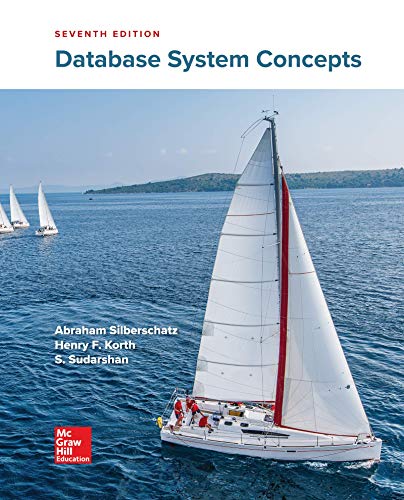
Python code:
import random
def main(): # main function definition
aGrades=[] # empty list to store Grades
for i in range(7): # loop to read 7 inputs
print("Input scores ",i+1," : ", end="") # a message to enter grade
n=int(input()) # input grade
aGrades.append(n) # add grade into list
print("Grade list before randomize: ",aGrades) # print list
random.shuffle(aGrades) # randomize list
numExams=len(aGrades)-1 # compute numExams
FinalExam=aGrades[-1] # store final grade
TotalPoints=sum(aGrades)-aGrades[-1] # compute TotalPoints
TestAverage=TotalPoints/numExams # compute TestAverage
FinalAverage=TestAverage*.6 + FinalExam*.4 # compute FinalAverage
print("Grade list after randomize is: ",aGrades) # print list
print("Test Average = %.2f"%TestAverage) # print test average
print("Final Average = %.2f "%FinalAverage) # print final average
main() # calling main function
Can you explain in a word doc explaining each line of code and their variables. .

Step by stepSolved in 2 steps

- Random list indexes (python) + FLOW CHARTarrow_forwardThe chapter is nested lists Create code in python using for loops Thanks!arrow_forward*Can you produce a flowchart for this code. import randomdef main(): # main function definitionaGrades=[] # empty list to store Gradesfor i in range(7): # loop to read 7 inputsprint("Input scores ",i+1," : ", end="") # a message to enter graden=int(input()) # input gradeaGrades.append(n) # add grade into listprint("Grade list before randomize: ",aGrades) # print list random.shuffle(aGrades) # randomize listnumExams=len(aGrades)-1 # compute numExamsFinalExam=aGrades[-1] # store final gradeTotalPoints=sum(aGrades)-aGrades[-1] # compute TotalPointsTestAverage=TotalPoints/numExams # compute TestAverageFinalAverage=TestAverage*.6 + FinalExam*.4 # compute FinalAverageprint("Grade list after randomize is: ",aGrades) # print listprint("Test Average = %.2f"%TestAverage) # print test averageprint("Final Average = %.2f "%FinalAverage) # print final averagemain() # calling main functionarrow_forward
- When using a for-loop to modify a list, you always have to make a copy of the list. (a) True (b) False Thank you!arrow_forwardPython code please help, indentation would be greatly appreciatedarrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- Parallel Lists JumpinJive.py >- Terminal Summary 1 # JumpinJava.py - This program looks up and prints t and prices of coffee orders. 2 # Input: In this lab, you use what you have learned about parallel lists to complete a partially completed Interactive Python program. 3 # Output: Name and price of coffee orders or error if add-in is not found 4 The program should either print the name and price for a coffee add-in from the Jumpin' Jive 5 # Declare variables. Coffee Shop or it should print the message "Sorry, we do not carry that.". 6 NUM_ITEMS = 5 # Named constant 8 # Initialized list of add-ins 9 addIns = ["Cream", "Cinnamon", "Chocolate", "Amarett "Whiskey"] Read the problem description carefully before you begin. The data file provided for this lab includes the necessary input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints 10 the error message if the add-in is not…arrow_forwardPythonarrow_forwardPython code Could you write the code in a different way but get the same result.arrow_forward
- C Programming Language Task: Deviation Write a program that prompts the user to enter N numbers and calculates which of the numbers has the largest deviation from the average of all numbers. You program should first prompt the user to enter how many numbers that will specify. The program should then scan for each number, separated by a newline. You should calculate the average value and return the number from the list which is furthest away from this average (to 2dp). Try using dynamic memory functions to store the incoming array of numbers on the heap. Code to build from: + 1 #include 2 #include 3 4 int main(void) { 5 6} 7 Output Example: deviation.c How many numbers? 5 Enter them: 1.0 2.0 6.0 3.0 4.0 Average: 3.20 Largest deviation from average: 6.00arrow_forward# This function takes a list of points and returns a new list of points, # beginning with the first point and then at each stage, moving to the closest point that has not yet been visited.# Note: the function should return a new list, not modify the existing list.# Hint: use a while loop to repeat while the list of remaining points is not emptyarrow_forward***python only*** Write a program that prompts the user for floating-point numbers until the user enters the word 'end' all by itself. The program should store the user-entered numbers in a list.The program will then randomly select half of those numbers and print these numbers (separated by a blank space). Hint: use a random number generator to make the randomselection.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
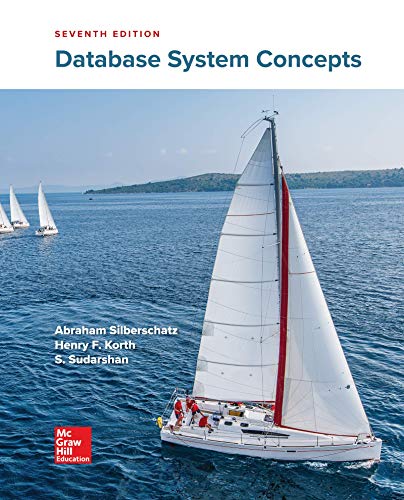
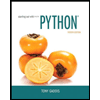
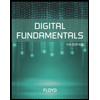
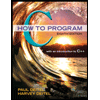
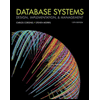
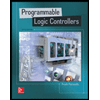