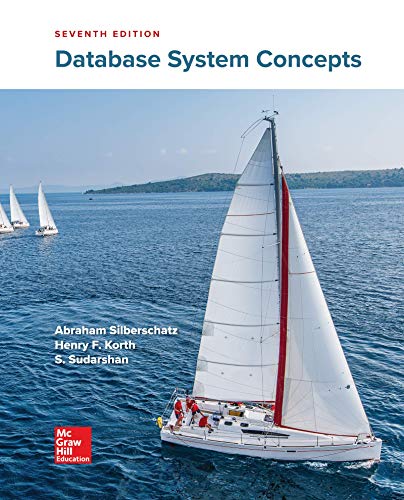
Don't Copy from other website.
ALL THREE ANSWER PLZ
Exercise 4
Import and edit the ShoppingCart02 project.
• Declare and initialize numeric fields:
– price (double)
– tax (double)
– quantity (int)
• Declare a double totalPrice:
– Assign a value, calculated from price , tax , and quantity.
Change message to include quantity:
– (example: “Alex wants to purchase 2 Shirts.”)
• Print another message showing the total cost.
Your program should produce a similar output:
Alex wants to purchase 2 Shirts
Total cost with tax is: $25.78
ShoppingCart02.java
package shoppingcart02;
public class ShoppingCart02 {
public static void main(String[] args) {
String custName = "Alex";
String itemDesc = "Shirts";
String message = custName+" wants to purchase a "+itemDesc;
// Declare and initialize numeric fields: price, tax, quantity.
// Declare and assign a calculated totalPrice
// Modify message to include quantity
System.out.println(message);
// Print another message with the total cost
}
}
Exercise 5
Import and edit the Casting01 project
Casting01.java
package casting01;
public class Casting01 {
public static void main(String[] args) {
//Declare and initialize a byte with a value of 128
//Observe NetBeans or eclipse complaint
//Declare and initialize a short with a value of 128
//Create a print statement that casts this short to a byte
//Declare and initialize a byte with a value of 127
//Add 1 to this variable and print it
//Add 1 to this variable again and print it again
}
}
Exercise 6
Import and edit the Parsing01 project.
• Declare and initialize 3 Strings with the following data:
String Variable Description Example Values
shirtPrice Text to be converted to an int: "15"
taxRate Text to be converted to a double: "0.05"
gibberish Gibberish "887ds7nds87dsfs"
Parse and multiply shirtPrice*taxRate to find the tax.
– Print this value.
• Try to parse taxRate as an int.
– Observe the error message.
• Try to parse gibberish as an int.
– Observe the error message.
Parsing01.java
package parsing01;
public class Parsing01 {
public static void main(String[] args) {
//Declare and intitialize 3 Strings: shirtPrice, taxRate, and gibberish
//Parse shirtPrice and taxRate, and print the total tax
//Try to parse taxRate as an int
//Try to parse gibberish as an int
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Expert this is warming, don't use any AI platform and don't give plagiarised response to me. If I see plagiarism and AI detection I'll report your account and reduce rating.You have a basic User class for a social media platform. Your task is to enhance the class by introducing a new method called 'post_status.' This method should take a single parameter, which is the status message that the user wants to post. The objective is to implement this method to update the user's status.Next, instantiate a new object of the User class, naming it 'SocialUser.' Utilize the extended class with the newly added 'post_status' method. Your next step is to post three distinct status messages for 'SocialUser'—namely, 'Excited for the weekend!', 'Just finished a great book!', and 'Exploring new hobbies!'To conclude, utilize the 'display_info' method to showcase the updated information about 'SocialUser.' Verify that the output includes details about the newly added status messages, ensuring that they…arrow_forwardThis is for the game brick breaker use Java to code what's posted down below.arrow_forwardCreate "People.java" Class for a "Person" with following functionality. People object should have following attributes/fields.. (make them private) their name as String, their address in next String and their phone number in 7 digit integer Put all this information in “People" object by doing the following Create a default Blank constructor - (Name should be "No Name", Address should be "000 Street, 000 City, North Pole, Earth", and Phone number should be 1234567) Create a full constructor Create accessors for all fields Create mutators for all fields (use proper this.) Create toString method for Peoplearrow_forward
- Create a new project named lab7_1. You will be implementing a Tacos class. For the class attributes, let’s use to type of taco, number of tacos, and price. Note that the price per taco of any type is 99 cents. Therefore, allow the taco type and number of tacos to be set directly, but not the price! The price should be set automatically based on the number of tacos entered. So a good idea may be to call the setter for the price inside the function that sets the number of tacos. Some other requirements: A default constructor and a constructor with parameters for your taco type and number of tacos member variables. You should also include setters and getters for these private member variables. However, make sure that the setter for price is private! You don’t want anyone outside the class modifying price. This setter should be called anytime you change the number of tacos. Your constructor needs to call a set method that allows you to set the two member variables, thus takes two…arrow_forwardGuide to working through the project Steps: Write the GradeBook constructor that reads student information from the data file into the ArrayList roster). For now, ignore the grades. Test your GradeBook class before proceeding to the next step. Create the Grade class. Test your Grade class before proceeding to the next step. Modify the Student class to include an ArrayList of Grade. Test the Student class before proceeding to the next step. Finish writing the GradeBook constructor to process the grades as they are read in from the data file. Test the GradeBook class before proceeding to the next step. Add additional functionality to the GradeBook and Student classes one method at a time. Here is Grade.jave code is shown below. public class Grade { // TODO: complete this class as described in this task write-up } GradeBook Class The GradeBook class has a single instance variable roster, an ArrayList of Student. This class performs all operations related to the scores of all…arrow_forwardSave the Word document and close them. Go to D2L and submit it by attaching the document file.You mayarrow_forward
- ques7 plz provide handwritten ans asaparrow_forwardMay you help with this code!!!! PLEASE, ASAP!arrow_forwardHow do I create this class in Java? Contact Class Requirements The contact object shall have a required unique contact ID string that cannot be longer than 10 characters. The contact ID shall not be null and shall not be updatable. The contact object shall have a required firstName String field that cannot be longer than 10 characters. The firstName field shall not be null. The contact object shall have a required lastName String field that cannot be longer than 10 characters. The lastName field shall not be null. The contact object shall have a required phone String field that must be exactly 10 digits. The phone field shall not be null. The contact object shall have a required address field that must be no longer than 30 characters. The address field shall not be null.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
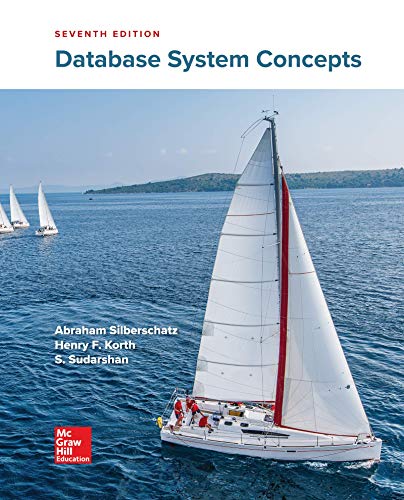
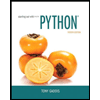
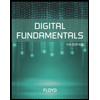
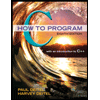
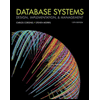
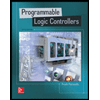