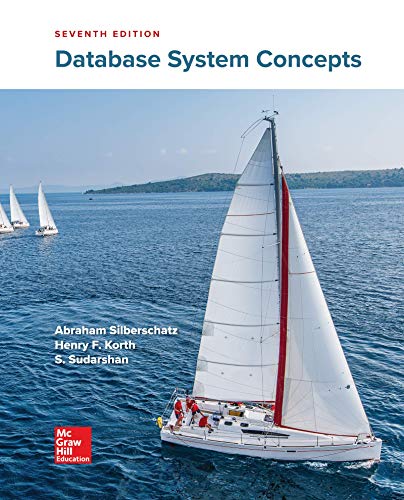
Concept explainers
python question
Implement the following:
1) Use a loop to continuously request an integer input from the console.
2) As long as the value is in the range from -10 to 10 inclusive, request new values.
3) Once the value is not in the range above, end the loop.
4) Prompt a message and print the sum of all valid values.
Input text can be any content. Just make sure to precisely match the output format below.
Write your code in the ANSWER area provided below (must include comments if using code is not covered in the course).
Example Output
Enter a number (-10, 10): 0
Enter a number (-10, 10): 1
Enter a number (-10, 10): -1
Enter a number (-10, 10): 11
Number out of range entered. Loop terminates.
0

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Unlabeled Break: Create a Java program that uses a "while" loop to output the numbers from 1 to 10 to the console. Add an "if" statement inside the loop to check if the number being outputted is greater than 5. If it is, use a "break" statement to exit the loop. Labeled Break: Create a Java program that uses a labeled "for" loop to output the numbers from 1 to 5, followed by the letters A to C to the console. Add an "if" statement inside the loop to check if the number being outputted is greater than 3. If it is, use a labeled "break" statement to exit the loop completely.arrow_forwardPlease write a loop that prints multiples of 3 from 300 down to 3. This will be a java programming line.arrow_forward4. Randomly generate an integer number in range [10, 99]. Check if the digit at 10°s position is greater than the digit at 1's position. If yes, swap these 2 digits to generate a new number. Display original number and new number. For example, if the number is 43, after process, the new number will be 34. If original number is 12, no process needed. If the number is 50, the new number will be 5arrow_forward
- Write a code that asks the user to enter several numbers that are a multiple of 5. If the user enters a value that is not a multiple of 5 it should print a message and prompt the user to enter a new number. The user can stop the process by inputting the string *done*. At the end, print the total number of values entered that are a multiple of 5.arrow_forwardP print out of range QUESTION 5 Implement the following: 1) Request an integer input by the user. 2) Display the sum value of 1/10 + 2/10 + 3/10 + ... + n/10 to two decimal places. 3) Display the average to two decimal places. Input text can be any content. Just make sure to precisely match the output format below. Write your entire program in the Answer area provided below (must include comments if using code is not covered in the course). Example Output Enter an integer: 50 The sum 1/10 + 2/10 + 3/10 +. + n/10 (n = 50) is 127.50. The average is 2.55. For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). BIUS Paragraph V Arial 10pt Click Save and Submit to save and submit. Click Save All Answers to save all answers. Programs Ev Ev A V Varrow_forwardA for statement is a loop that goes through a list of things. Therefore, it keeps running as long as there are objects to process. Is this a true or a false statement?arrow_forward
- Write a program that displays a weekly payroll report. A loop in the program should ask the user for the employee number, gross pay, state tax, federal tax, and FICA withholdings. The loop will terminate when 0 is entered for the employee number. After the date is entered, the program should display totals for gross pay, state tax, federal tax, FICA withholdings, and net pay.arrow_forward2. Randomly generate an integer number in range [100, 999]. (a) Check if this number is even or odd, display message “[number] is an even number" or “[number] is an odd number". For example, if the number is 10, display message “10 is an even number". (b) Check if this number is divisible by 7 and 11. Display message showing result. (c) Check if this number is divisible by 9 or 13. Display message showing result. (d) Check if this number is divisible by 5 or 12, but not both. Display resultarrow_forwardGiven a positive integer n, the following rules will always create a sequence that ends with 1, called the hailstone sequence: If n is even, divide it by 2 If n is odd, multiply it by 3 and add 1 (i.e. 3n +1) Continue until n is 1 Write a program that reads an integer as input and prints the hailstone sequence starting with the integer entered. Format the output so that ten integers, each separated by a tab character (\t), are printed per line. The output format can be achieved as follows:print(n, end='\t') Ex: If the input is: 25 the output is: 25 76 38 19 58 29 88 44 22 11 34 17 52 26 13 40 20 10 5 16 8 4 2 1 this is what i have n = int(input())count = 1while n != 1: if count%10==0: print(n,'\t') else: print(n, end='\t') if n%2==0: n = int(n/2) else: n = int(n*3 + 1) count = count + 1 print(n) the output is correct but theres whitespaces that arnt neededarrow_forward
- String personName is read from input. Output personName left aligned with a width of 6, followed by " Taylor". End with a newline. Ex: If the input is Juno, then the output is: Juno Taylorarrow_forwardIn PYTHON Using one of the loop construct draw:- Ten dashed Horizontal lines- Each line of length 150 units (or pixels)- All lines aligned at the same left vertical margin- Lines 10 units apart each other Note- Set the length of solid segment to 10 units- Set the length of the non-visible segment to 5 units Assumption- Use of Turtle library (i will check the answer, it has to be correct)arrow_forwardPrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
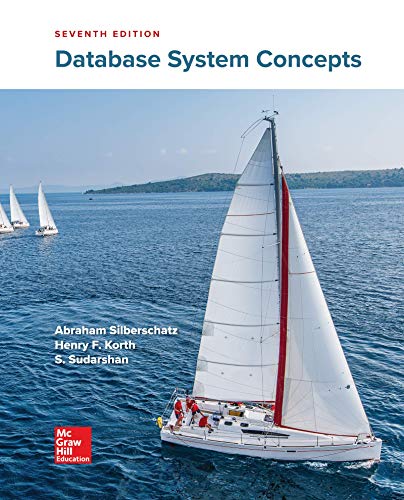
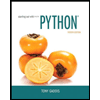
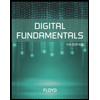
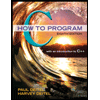
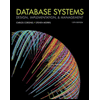
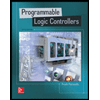