Im trying to plot three plots 1. the actual CCF data in a plot 2. the mean of the CCF data in a plot 3. the residual of the CCF data, which is the CCF data - mean I have plot 1 and 2 operating okay import matplotlib.pyplot as plt import glob from astropy.io import fits from astropy.wcs import WCS import numpy as np # Step 1: Import the necessary libraries and modules # Step 2: Create the main figure and axis objects for the initial plot fig, ax = plt.subplots(figsize=(10, 10)) # Step 3: Get the file paths of FITS files data_dir = glob.glob('/Users/petrderuyter/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits') # Step 4: Initialize empty lists for storing wavelength and flux data lams = [] fluxs = [] # Step 5: Read and process each FITS file for file_path in data_dir: hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] lams.append(lam) fluxs.append(flux) ax.plot(lam, flux) # Step 6: Calculate the mean flux mean_ccf = np.mean(fluxs, axis=0) # Step 7: Create a new figure and axis objects for the second plot (mean data) fig_mean, ax_mean = plt.subplots(figsize=(10, 10)) # Step 8: Plot the mean data on the second axis ax_mean.plot(lams[1], mean_ccf, color='k', linewidth=2) #Calculate the residual mean_ccf = np.mean(data_dir) residuals = data_dir - mean_ccf # Step 9: Customize the plot ax_mean.set_xlabel('RV [km/s]') ax_mean.set_ylabel('Normalized CCF') # Step 10: Set the title and display the plots plt.title('Mean CCF') plt.show() # Step 11: Print the mean flux print(mean_ccf) # Plot residuals plt.plot(residuals, marker='o') plt.axhline(0, color='red', linestyle='--') # Add a horizontal line at y=0 plt.xlabel('Index') plt.ylabel('Residual') plt.title('Residual Plot of CCF Data') plt.grid(True) plt.show()
Im trying to plot three plots
1. the actual CCF data in a plot
2. the mean of the CCF data in a plot
3. the residual of the CCF data, which is the CCF data - mean
I have plot 1 and 2 operating okay
import matplotlib.pyplot as plt
import glob
from astropy.io import fits
from astropy.wcs import WCS
import numpy as np
# Step 1: Import the necessary libraries and modules
# Step 2: Create the main figure and axis objects for the initial plot
fig, ax = plt.subplots(figsize=(10, 10))
# Step 3: Get the file paths of FITS files
data_dir = glob.glob('/Users/petrderuyter/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits')
# Step 4: Initialize empty lists for storing wavelength and flux data
lams = []
fluxs = []
# Step 5: Read and process each FITS file
for file_path in data_dir:
hdul = fits.open(file_path)
data = hdul[1].data
h1 = hdul[1].header
flux = data[1]
w = WCS(h1, naxis=1, relax=False, fix=False)
lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0]
lams.append(lam)
fluxs.append(flux)
ax.plot(lam, flux)
# Step 6: Calculate the mean flux
mean_ccf = np.mean(fluxs, axis=0)
# Step 7: Create a new figure and axis objects for the second plot (mean data)
fig_mean, ax_mean = plt.subplots(figsize=(10, 10))
# Step 8: Plot the mean data on the second axis
ax_mean.plot(lams[1], mean_ccf, color='k', linewidth=2)
#Calculate the residual
mean_ccf = np.mean(data_dir)
residuals = data_dir - mean_ccf
# Step 9: Customize the plot
ax_mean.set_xlabel('RV [km/s]')
ax_mean.set_ylabel('Normalized CCF')
# Step 10: Set the title and display the plots
plt.title('Mean CCF')
plt.show()
# Step 11: Print the mean flux
print(mean_ccf)
# Plot residuals
plt.plot(residuals, marker='o')
plt.axhline(0, color='red', linestyle='--') # Add a horizontal line at y=0
plt.xlabel('Index')
plt.ylabel('Residual')
plt.title('Residual Plot of CCF Data')
plt.grid(True)
plt.show()


Step by step
Solved in 3 steps

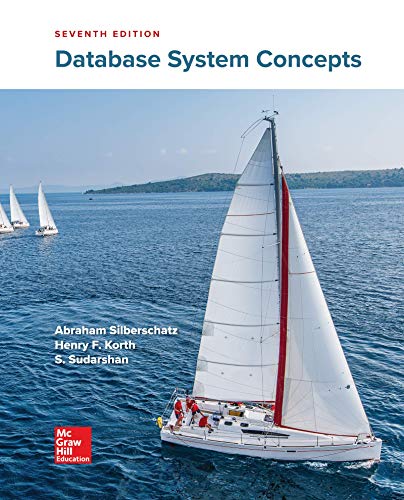
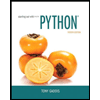
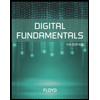
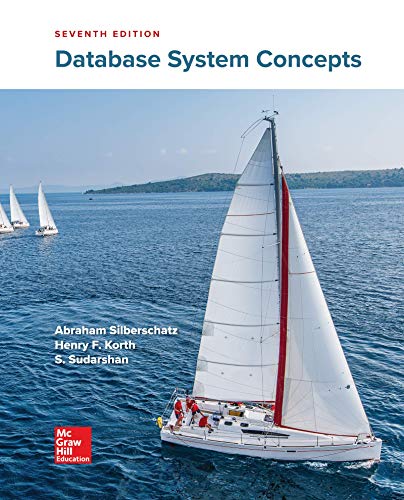
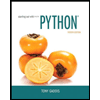
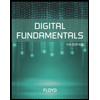
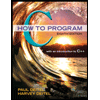
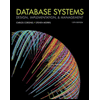
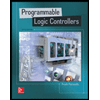