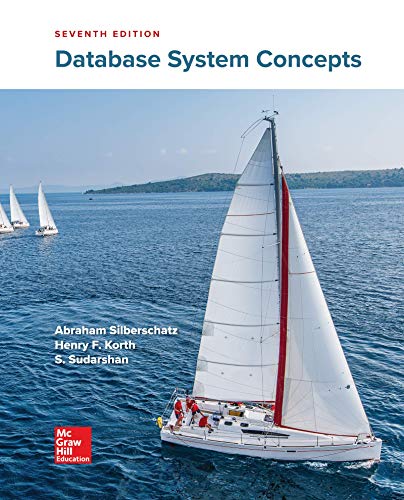
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
I would like for you to review this code and help me figure out why it isn't displaying the calculated temperatures when I test it. It seems to be an issue with the .js code.
index.html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Convert Temperatures</title>
<link rel="stylesheet" href="convert_temp.css">
</head>
<body>
<main>
<h1>Convert temperatures</h1>
<div>
<input type="radio" name="conversion_type" id="to_celsius" checked>Fahrenheit to Celsius
</div>
<div>
<input type="radio" name="conversion_type" id="to_fahrenheit">Celsius to Fahrenheit
</div>
<div>
<label id="degree_label_1">Enter F degrees:</label>
<input type="text" id="degrees_entered">
</div>
<div>
<label id="degree_label_2">Degrees Celsius:</label>
<input type="text" id="degrees_computed" disabled>
</div>
<div>
<label></label>
<input type="button" id="convert" value="Convert" />
</div>
</main>
<script src="convert_temp.js"></script>
</body>
</html>
convert_temp.css
body {
font-family: Arial, Helvetica, sans-serif;
background-color: white;
margin: 0 auto;
width: 500px;
border: 3px solid blue;
padding: 0 2em 1em;
}
h1 {
color: blue;
}
div {
margin-bottom: 1em;
}
label {
display: inline-block;
width: 11em;
text-align: right;
}
input {
margin-left: 1em;
margin-right: 0.5em;
}
span {
color: red;
}
#convert {
width: 10em;
}
/*div that's the 3rd child of its parent element (main) */
div:nth-child(3) {
margin-bottom: 1.5em;
}
convert_temp.js
"use strict";
const $ = selector => document.querySelector(selector);
/*********************
* helper functions *
**********************/
const calculateCelsius = temp => (temp-32) * 5/9;
const calculateFahrenheit = temp => temp * 9/5 + 32;
const toggleDisplay = (label1Text, label2Text) => {
document.getElementById("degree_label_1").innerHTML = label1Text;
document.getElementById("degree_label_2").innerHTML = label2Text;
document.getElementById("degrees_computed").value = "";
}
/****************************
* event handler functions *
*****************************/
const convertTemp = () => {
let degree = document.getElementById("degrees_entered").value;
degree = parseFloat(degree);
if (isNaN(degree)) {
document.getElementById("message").innerHTML =
"Please enter a valid degree!.";
}
else {
let result;
document.getElementById("message").innerHTML = "";
let check = document.getElementById("to_celsius");
if (check.checked) {
result = calculateCelsius(degree);
} else {
result = calculateFahrenheit(degree);
}
document.getElementById("degrees_computed").value = Math.round(result);
}
};
const toCelsius = () => toggleDisplay("Enter F degrees:", "Degrees Celsius:");
const toFahrenheit = () => toggleDisplay("Enter C degrees:", "Degrees Fahrenheit:");
document.addEventListener("DOMContentLoaded", () => {
// add event handlers
$("#convert").addEventListener("click", convertTemp);
$("#to_celsius").addEventListener("click", toCelsius);
$("#to_fahrenheit").addEventListener("click", toFahrenheit);
// move focus
$("#degrees_entered").focus();
});
Expert Solution

arrow_forward
Step 1
Please refer to the following step for the complete solution to the problem above.
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Edit the code to add a password and use json.stringify to store all the info correctly. Not as an object but to convert it to a string representation. Existing code: register.html <html><head><title>Registration Page</title><script src="./js/register.js"></script></head><body<br /><br /><form>Email:<input type="text" id="email" name="email"/> <br> <br> Phone:<input type="text" id="phone" name="phone"/> <br> <br> Address:<textarea id="address" name="address"> </textarea> <br> <br> City:<input type="text" id="city" name="city"/> <br> <br> State:<input type="text" id="state" name="state"/> <br> <br> ZIP Code:<input type="text" id="zip" name="zip"/> <br> <br> Contact Me:<input type="radio" id="contact" name="contact" value="text"/> Text <input type="radio" id="contact" name="contact"…arrow_forwardI need them to react similarly to what's going on in this gif I also put the problem in here as well that I'm having a hard time with <!DOCTYPE html><html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>homework 14</title> <style> /*formatting.css */ .sticky-note-title { /* DON'T EDIT ME */ padding: 1rem; font-size: 2rem;}.sticky-note-body { /* DON'T EDIT ME */ transform: rotate(0); padding: 1rem; font-size: 1.5rem;}.sticky-board { /* DON'T EDIT ME */ margin-top: 2em; display: flex; padding: 3em; flex-wrap: wrap; gap: 1em; justify-content: center;}.sticky-note { flex-shrink: 0; width: 20em; height: 20em; /* Add styles here */ }#first { transform: rotate(75deg); transition: transform 500ms; }#second { transform: rotate(75deg); transition: transform 500ms; }…arrow_forwardThis is my footer code for an HTML 5 page (part of my assignment), but the footer doesn't show up. It just displays the text. Not sure what the issue is. body > footer address { background-color: rgb(222,128,60); color: white; color: rgba(255, 255, 255, 0.7); font: normal small-caps bold 0.9em/3em Quicksand, Verdana, Geneva, sans-serif; text-align: center;}arrow_forward
- Using comments in the code, can you provide a line by line explination as to what the below HTML file is doing? The file relates to a WebGL program if that helps you. Please & thank you HTML File: <!DOCTYPE html> <html> <head> <meta http-equiv="Content-Type" content="text/html;charset=utf-8" > <title>3D Sierpinski Gasket</title> <script id="vertex-shader" type="x-shader/x-vertex"> #version 300 es in vec3 aPosition; in vec3 aColor; out vec4 vColor; void main() { gl_Position = vec4(aPosition, 1.0); vColor = vec4(aColor, 1.0); } </script> <script id="fragment-shader" type="x-shader/x-fragment"> #version 300 es precision mediump float; in vec4 vColor; out vec4 fColor; void main() { fColor = vColor; } </script> <script type="text/javascript" src="../Common/initShaders.js"></script> <script type="text/javascript" src="../Common/MVnew.js"></script> <script type="text/javascript"…arrow_forward<!-- Write your code here --> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <title> Chapter 1, Extend Your Knowledge <p> Benjamin Bain, 9/10/2021 <hr> <p> Guideline 1.1arrow_forwardthe html file is like this ---------------------------- <!DOCTYPE html> <html lang="en"> <head> <style>*{ background-color: gray;}a{ text-decoration: none; color: yellow; font-size: 20px;}a:hover{ transition: 1.1px;}input{ background-color: #fff;} .div1 { border:2pxoutsetred; background-color:lightblue; text-align:center; }.boxes{ float: left; width: 49%; border: 2px solid black; background-color: gray; color: #fff;} </style> <script> function maxLengthCheck(object) { if (object.value.length > object.maxLength) object.value = object.value.slice(0, object.maxLength) } function isNumeric (evt) { var theEvent = evt || window.event; var key = theEvent.keyCode || theEvent.which; key = String.fromCharCode (key); var regex = /[0-9]|\./; if ( !regex.test(key) ) { theEvent.returnValue = false; if(theEvent.preventDefault) theEvent.preventDefault(); } } function orderfunction(){ alert("Thank you for ordering from my…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
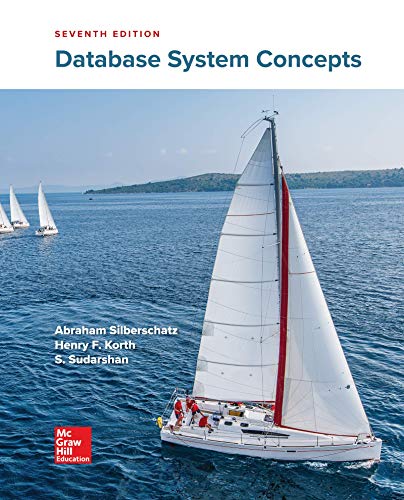
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
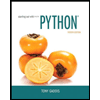
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
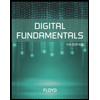
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
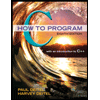
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
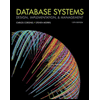
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
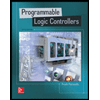
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education