i need help for this code to create it in seperate files within the same file for each class. basically multiple files with the classes organized on visual studios. also could you include a flowchart for this code #include #include // Base class: Citizen class Citizen { protected: std::string name; public: Citizen(const std::string & _name) : name(_name) {} virtual ~Citizen() {} virtual void payTaxes() const = 0; virtual void speak() const = 0; }; // Derived class: Wolf class Wolf { public: void howl() const { std::cout << "The wolf howls." << std::endl; } void growl() const { std::cout << "The wolf growls." << std::endl; } }; // Derived class: Human class Human : public Citizen { public: Human(const std::string & _name) : Citizen(_name) {} void payTaxes() const override { std::cout << name << " pays taxes in dollars." << std::endl; } void speak() const override { std::cout << name << " says: 'Hello!'" << std::endl; } }; // Derived class: Troll class Troll : public Citizen { public: Troll(const std::string & _name) : Citizen(_name) {} void payTaxes() const override { std::cout << name << " pays taxes in gems." << std::endl; } void speak() const override { std::cout << name << " says: 'Grunt!'" << std::endl; } }; // Derived class: Dwarf class Dwarf : public Citizen { public: Dwarf(const std::string & _name) : Citizen(_name) {} void payTaxes() const override { std::cout << name << " pays taxes in gold." << std::endl; } void speak() const override { std::cout << name << " says: 'Greetings!'" << std::endl; } }; // Derived class: Werewolf class Werewolf : public Human, public Wolf { public: Werewolf(const std::string & _name) : Human(_name) {} void payTaxes() const override { std::cout << name << " pays taxes as a werewolf." << std::endl; } void speak() const override { std::cout << name << " says: 'Awoooo!'" << std::endl; } }; // Derived class: Zombie class Zombie : public Human { public: Zombie(const std::string & _name) : Human(_name) {} void payTaxes() const override { std::cout << name << " is undead and does not pay taxes." << std::endl; } void speak() const override { std::cout << name << " says: 'Braaaaains!'" << std::endl; } }; // Derived class: Vampire class Vampire : public Human { public: Vampire(const std::string & _name) : Human(_name) {} void payTaxes() const override { std::cout << name << " pays taxes in blood." << std::endl; } void speak() const override { std::cout << name << " says: 'I vant to suck your blood!'" << std::endl; } }; // Derived class: Nobbs class Nobbs : public Human { public: Nobbs(const std::string & _name) : Human(_name) {} void payTaxes() const override { std::cout << name << " pays taxes as a noble." << std::endl; } void speak() const override { std::cout << name << " says: 'Your Highness!'" << std::endl; } }; int main() { const int numCitizens = 6; Citizen* citizens[numCitizens] = { new Human("John"), new Troll("Grug"), new Dwarf("Gimli"), new Werewolf("Jacob"), new Zombie("Zed"), new Vampire("Dracula") }; for (int i = 0; i < numCitizens; ++i) { citizens[i]->payTaxes(); citizens[i]->speak(); std::cout << std::endl; } // Cleanup for (int i = 0; i < numCitizens; ++i) { delete citizens[i]; } return 0; }
i need help for this code to create it in seperate files within the same file for each class. basically multiple files with the classes organized on visual studios. also could you include a flowchart for this code
#include <iostream>
#include <string>
// Base class: Citizen
class Citizen {
protected:
std::string name;
public:
Citizen(const std::string & _name) : name(_name) {}
virtual ~Citizen() {}
virtual void payTaxes() const = 0;
virtual void speak() const = 0;
};
// Derived class: Wolf
class Wolf {
public:
void howl() const {
std::cout << "The wolf howls." << std::endl;
}
void growl() const {
std::cout << "The wolf growls." << std::endl;
}
};
// Derived class: Human
class Human : public Citizen {
public:
Human(const std::string & _name) : Citizen(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes in dollars." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Hello!'" << std::endl;
}
};
// Derived class: Troll
class Troll : public Citizen {
public:
Troll(const std::string & _name) : Citizen(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes in gems." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Grunt!'" << std::endl;
}
};
// Derived class: Dwarf
class Dwarf : public Citizen {
public:
Dwarf(const std::string & _name) : Citizen(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes in gold." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Greetings!'" << std::endl;
}
};
// Derived class: Werewolf
class Werewolf : public Human, public Wolf {
public:
Werewolf(const std::string & _name) : Human(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes as a werewolf." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Awoooo!'" << std::endl;
}
};
// Derived class: Zombie
class Zombie : public Human {
public:
Zombie(const std::string & _name) : Human(_name) {}
void payTaxes() const override {
std::cout << name << " is undead and does not pay taxes." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Braaaaains!'" << std::endl;
}
};
// Derived class: Vampire
class Vampire : public Human {
public:
Vampire(const std::string & _name) : Human(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes in blood." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'I vant to suck your blood!'" << std::endl;
}
};
// Derived class: Nobbs
class Nobbs : public Human {
public:
Nobbs(const std::string & _name) : Human(_name) {}
void payTaxes() const override {
std::cout << name << " pays taxes as a noble." << std::endl;
}
void speak() const override {
std::cout << name << " says: 'Your Highness!'" << std::endl;
}
};
int main() {
const int numCitizens = 6;
Citizen* citizens[numCitizens] = {
new Human("John"),
new Troll("Grug"),
new Dwarf("Gimli"),
new Werewolf("Jacob"),
new Zombie("Zed"),
new Vampire("Dracula")
};
for (int i = 0; i < numCitizens; ++i) {
citizens[i]->payTaxes();
citizens[i]->speak();
std::cout << std::endl;
}
// Cleanup
for (int i = 0; i < numCitizens; ++i) {
delete citizens[i];
}
return 0;
}

Step by step
Solved in 3 steps with 1 images

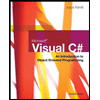
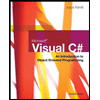