I need help creating a Java code where when the user types 2 the program would just show the number of throws it took. The rest of the Java code is fine but I can't input the elseif when the user types 2 Output is when the user types 2 when the program prints to "Just print the answer" Java code: import java.util.Scanner; public class Dice_Face_In_a_row_FallProject_2 { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int diceFaceNumber, numTimes, numThrows; char choice; do { System.out.println(" This game throws a dice until a particular dice face number"); System.out.println(" appears in a row a certain number of times."); System.out.println("Please enter the version you want:"); System.out.println(" 1) Trace the game."); System.out.println(" 2) Just give the answer"); System.out.println("Please enter 1 or 2"); Scanner input = new Scanner(System.in); int a = input.nextInt(); if (a == 1) { System.out.println("Please enter the dice face number you would like to be repeated. "); diceFaceNumber = input.nextInt(); System.out.println("How many times you want that die face number to appear in a row? : "); numTimes = input.nextInt(); computeNumThrows(diceFaceNumber, numTimes); } else (a == 2){ int numThrows = 0; int count = 0; while (count < numTimes) { int die = (int)(Math.random() * 6) + 1; numThrows++; System.out.println("Please enter the dice face number you would like to be repeated. "); diceFaceNumber = input.nextInt(); System.out.println("How many times you want that die face number to appear in a row? : "); numTimes = input.nextInt(); } System.out.println("Would you like to play the game again? "); System.out.println("Please enter Y for yes or N for no)"); choice = scanner.next().charAt(0); } while (choice == 'Y'); } private static void computeNumThrows(int diceFaceNumber, int numTimes) { int numThrows = 0; int count = 0; while (count < numTimes) { int die = (int)(Math.random() * 6) + 1; numThrows++; System.out.println("you got a " + die); if (die == diceFaceNumber) { count++; System.out.println("Number of times in a row so far is " + count); System.out.println("Number of throws is " + numThrows); } else { int c = 0; System.out.println("Number of times in a row so far is " + c); System.out.println("Number of throws is " + numThrows); } } } }
I need help creating a Java code where when the user types 2 the program would just show the number of throws it took. The rest of the Java code is fine but I can't input the elseif when the user types 2
Output is when the user types 2 when the program prints to "Just print the answer"
Java code:
import java.util.Scanner;
public class Dice_Face_In_a_row_FallProject_2 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int diceFaceNumber, numTimes, numThrows;
char choice;
do {
System.out.println(" This game throws a dice until a particular dice face number");
System.out.println(" appears in a row a certain number of times.");
System.out.println("Please enter the version you want:");
System.out.println(" 1) Trace the game.");
System.out.println(" 2) Just give the answer");
System.out.println("Please enter 1 or 2");
Scanner input = new Scanner(System.in);
int a = input.nextInt();
if (a == 1) {
System.out.println("Please enter the dice face number you would like to be repeated. ");
diceFaceNumber = input.nextInt();
System.out.println("How many times you want that die face number to appear in a row? : ");
numTimes = input.nextInt();
computeNumThrows(diceFaceNumber, numTimes);
}
else (a == 2){
int numThrows = 0;
int count = 0;
while (count < numTimes) {
int die = (int)(Math.random() * 6) + 1;
numThrows++;
System.out.println("Please enter the dice face number you would like to be repeated. ");
diceFaceNumber = input.nextInt();
System.out.println("How many times you want that die face number to appear in a row? : ");
numTimes = input.nextInt();
}
System.out.println("Would you like to play the game again? ");
System.out.println("Please enter Y for yes or N for no)");
choice = scanner.next().charAt(0);
} while (choice == 'Y');
}
private static void computeNumThrows(int diceFaceNumber, int numTimes) {
int numThrows = 0;
int count = 0;
while (count < numTimes) {
int die = (int)(Math.random() * 6) + 1;
numThrows++;
System.out.println("you got a " + die);
if (die == diceFaceNumber) {
count++;
System.out.println("Number of times in a row so far is " + count);
System.out.println("Number of throws is " + numThrows);
} else {
int c = 0;
System.out.println("Number of times in a row so far is " + c);
System.out.println("Number of throws is " + numThrows);
}
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

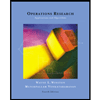
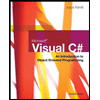
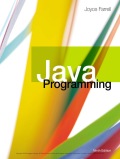
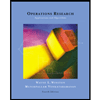
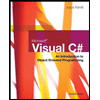
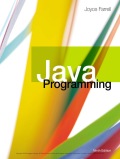