I have the code bellow. Now I'm ask to display the number of elements in the arrays read from files. Files contain 200 random numbers between them. The problem is if I pres enter at the last element in the text file the count will return 201 elements when in reality there are 200 and the empty space at the end. if I put -1 in the count then the problem is if a file has 200 numbers and no extra blank it. 2. I need to Find out for example how many times number 9 apears in file/array 1 and how many times in file/array2
I have the code bellow.
Now I'm ask to display the number of elements in the arrays read from files. Files contain 200 random numbers between them. The problem is if I pres enter at the last element in the text file the count will return 201 elements when in reality there are 200 and the empty space at the end. if I put -1 in the count then the problem is if a file has 200 numbers and no extra blank it.
2. I need to Find out for example how many times number 9 apears in file/array 1 and how many times in file/array2
Code:
#include <iostream>
#include <fstream>
using namespace std;
class Multiplicity { //Class
private:
//Inititate Values
const static int size = 300;
ifstream file1, file2;
int array1[size], array2[size];
void getData() {
file1.open("comFile111.txt"); //Open File 1
if (!file1.is_open()) { //Check is file was found
cout << "File 1 Not Found!" << endl;
exit(1);
}
file2.open("comFile222.txt"); //Open File 2
if (!file2.is_open()) { //Check is file was found
cout << "File 2 Not Found!" << endl;
exit(2);
}
}
void computeData() {
//Get the size of the first array by populating the first array using file1.
int size1 = readFile(array1, file1);
//Get the size of the second array by populating the second array using file2.
int size2 = readFile(array2, file2);
file1.close(); //Closing file used for array1
file2.close(); //Closing file used for array2
//Assigning pointers
int* ptr1 = array1; //Pointer for Array 1
int* ptr2 = array2; //Pointer for Array 2
compareSize(size1, size2);//Compare size of arrays if they are not the same a messaje will be display and program will end
bubbleSort(ptr1, size1); //Bubble sort Object call - Fully functionanl
cout << "The number of elements inside Array 1: " << sizeof(array1)/sizeof(array1[0]) << "\n" << endl;
bubbleSort(ptr2, size2); //Bubble sort Object call - Fully functionanl
cout << "The number of elements inside Array 2: " << countElements(ptr2, size2) << "\n" << endl;
compareElements(ptr1, ptr2, size1); //Call for object to compare elements in arrays usign pointers, and display the results of the comparasion
}
//Read files
int readFile(int* array, ifstream& fin)
{
int count = 0;
while (!fin.eof()) { //read file until end of file and passes values
fin >> *(array + count);
count++;
}
return count-1;
}
//Swap function used for bubble sort
void swap(int* xp, int* yp) {
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// Bubble Sort Functional - First thought if quick sort was not posible
void bubbleSort(int* array, int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (*(array + j) > *(array + j + 1)) {
swap((array + j), (array + j + 1));
}
}
}
}
//Compare Size of Arrays Object
void compareSize(int size1, int size2) {
if (size1 != size2) {
cout << "The amount of elements in the arrays are not the same, multiplicity is not posible." << endl;
exit(3);
}
}
//Compare Elements of Arrays Object
void compareElements(int* array1, int* array2, int s) {
for (int i = 0; i < s; i++) {
if (*(array1 + i) != *(array2 + i)) {
cout << "The elements in the arrays are not the same and multiplicity is not posible." << endl;
return;
}
}
cout << "The elements in the arrays are the same and multiplicity is posible." << endl;
return;
}
//Print Array function for testing
void printArray(int* arr, int size) {
//int count = 0;
for (int i = 0; i < size; i++) {
cout << *(arr +i ) << endl;
//count++;
}
//cout << "Size of Array: " << count << endl;
}
//Count Array Elements
int countElements(int* arr, int size) {
int count = 0;
for (int i = 0; i < size; i++) {
if (*(arr + 1) != * (arr + size)) {
count++;
}
}
return count;
}
public:
//Constructor
Multiplicity() {
getData(); // Calls for get data object
computeData(); //Calls for Compute data object for results
}
};
int main()
{
Multiplicity M; //Creats an instance of Class
return 0;
}

#include <iostream>
#include <fstream>
using namespace std;
class Multiplicity { //Class
private:
//Inititate Values
const static int size = 300;
ifstream file1, file2;
int array1[size], array2[size];
void getData() {
file1.open("comFile111.txt"); //Open File 1
if (!file1.is_open()) { //Check is file was found
cout << "File 1 Not Found!" << endl;
exit(1);
}
file2.open("comFile222.txt"); //Open File 2
if (!file2.is_open()) { //Check is file was found
cout << "File 2 Not Found!" << endl;
exit(2);
}
}
void computeData() {
//Get the size of the first array by populating the first array using file1.
int size1 = readFile(array1, file1);
int array1_with_size_size1[size1];
copy(array1, array1 + size1, array1_with_size_size1);
//Get the size of the second array by populating the second array using file2.
int size2 = readFile(array2, file2);
int array2_with_size_size2[size2];
copy(array2, array2 + size2+1, array2_with_size_size2);
file1.close(); //Closing file used for array1
file2.close(); //Closing file used for array2
//Assigning pointers
int* ptr1 = array1_with_size_size1; //Pointer for Array 1
int* ptr2 = array2_with_size_size2; //Pointer for Array 2
compareSize(size1, size2);//Compare size of arrays if they are not the same a messaje will be display and program will end
bubbleSort(ptr1, size1); //Bubble sort Object call - Fully functionanl
cout << "The number of elements inside Array 1: " << sizeof(array1_with_size_size1)/sizeof(array1_with_size_size1[0]) << "\n" << endl;
bubbleSort(ptr2, size2); //Bubble sort Object call - Fully functionanl
cout << "The number of elements inside Array 2: " << sizeof(array2_with_size_size2)/sizeof(array2_with_size_size2[0]) << "\n" << endl;
compareElements(ptr1, ptr2, size1); //Call for object to compare elements in arrays usign pointers, and display the results of the comparison
compareElements(ptr1, ptr2, size1, 10);
}
//Read files
int readFile(int* array, ifstream& fin)
{
int count = 0;
string data;
while (!fin.eof()) { //read file until end of file and passes values
//fin >> data;
getline(fin, data);
//cout << data << endl;
if (data == "") break;
*(array + count) = stoi(data);
count++;
}
cout << endl << endl;
return count;
}
//Swap function used for bubble sort
void swap(int* xp, int* yp) {
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// Bubble Sort Functional - First thought if quick sort was not posible
void bubbleSort(int* array, int size) {
for (int i = 0; i < size - 1; i++) {
for (int j = 0; j < size - i - 1; j++) {
if (*(array + j) > *(array + j + 1)) {
swap((array + j), (array + j + 1));
}
}
}
}
//Compare Size of Arrays Object
void compareSize(int size1, int size2) {
if (size1 != size2) {
cout << "The amount of elements in the arrays are not the same, multiplicity is not posible." << endl;
exit(3);
}
}
//Compare Elements of Arrays Object
void compareElements(int* array1, int* array2, int s) {
for (int i = 0; i < s; i++) {
if (*(array1 + i) != *(array2 + i)) {
cout << "The elements in the arrays are not the same and multiplicity is not posible." << endl;
return;
}
}
cout << "The elements in the arrays are the same and multiplicity is posible." << endl;
return;
}
//Compare Elements of Arrays Object
void compareElements(int* array1, int* array2, int s, int target) {
int count1 = 0, count2 = 0;
for (int i = 0; i < s; i++) {
if (*(array1 + i) == target)
count1++;
if (*(array2 + i) == target)
count2++;
}
cout << "The element " << target << " appeared " << count1 << " time(s) in array1" << endl;
cout << "The element " << target << " appeared " << count2 << " time(s) in array2" << endl;
return;
}
//Print Array function for testing
void printArray(int* arr, int size) {
//int count = 0;
for (int i = 0; i < size; i++) {
cout << *(arr +i ) << endl;
//count++;
}
//cout << "Size of Array: " << count << endl;
}
//Count Array Elements
int countElements(int* arr, int size) {
int count = 0;
for (int i = 0; i < size; i++) {
if (*(arr + 1) != * (arr + size)) {
count++;
}
}
return count;
}
public:
//Constructor
Multiplicity() {
getData(); // Calls for get data object
computeData(); //Calls for Compute data object for results
}
};
int main()
{
Multiplicity M; //Creats an instance of Class
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

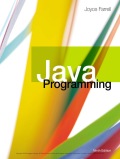
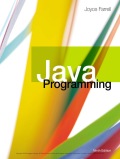