I have my code here and I am getting errors (I have also attached my code): " import java.util.Compiler; public class Test Score { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Ënter the first exam Score: "); Double Score1 = scan.nextDouble(); System.out.println("Enter the second exam Score: "); Double Score2 = scan.nextDouble(); System.out.println("Enter the third exam Score: "); Double Score3 = scan.nextDouble(); System.out.println("Enter the fourth exam Score: "); Double Score4 = scan.nextDouble(); System.out.println("Enter the fifth exam Score: "); Double Score5 = scan.nextDouble(); Double total = Score1 + Score2 + Score3 + Score4 + Score5 Double average = total/5; if (average >= 90) { System.out.printf("your average was a: %f and recieved an A in this Class", average ); } else if (average >= 80) { System.out.printf("your average was a: %f and recieved an B in this class", average ); } else if (average >= 70) { System.out.printf("your average was a: %f and recieved an C in this class", average ); } else if (average >= 60) { System.out.printf("your average was a: %f and recieved an D in this class", average ); } else { System.out.printf("your average was a: %f and recieved an F in this class", average ); } }" What is wrong with my code? Also, how can I input this information: " Write a program that ask the user to enter a list of positive scores until the user enters a negative score to terminate the input. You need to store these scores in an array. You can assume the maximum number of scores the user may enter is 50. However, you need to keep track of the actual number of scores entered. Write 2 methods: 1. calculateAverage(): this method takes the list of scores and return the average score. 2. countPerfectScores(): this method takes the list of scores and return the number of perfect scores (100). The main program reads the input and calls these methods and print the results. Please make sure you write a comment line to document what your method does."
I have my code here and I am getting errors (I have also attached my code): "
import java.util.Compiler;
public class Test Score {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
System.out.println("Ënter the first exam Score: ");
Double Score1 = scan.nextDouble();
System.out.println("Enter the second exam Score: ");
Double Score2 = scan.nextDouble();
System.out.println("Enter the third exam Score: ");
Double Score3 = scan.nextDouble();
System.out.println("Enter the fourth exam Score: ");
Double Score4 = scan.nextDouble();
System.out.println("Enter the fifth exam Score: ");
Double Score5 = scan.nextDouble();
Double total = Score1 + Score2 + Score3 + Score4 + Score5
Double average = total/5;
if (average >= 90) {
System.out.printf("your average was a: %f and recieved an A in this Class", average );
} else if (average >= 80) {
System.out.printf("your average was a: %f and recieved an B in this class", average );
} else if (average >= 70) {
System.out.printf("your average was a: %f and recieved an C in this class", average );
} else if (average >= 60) {
System.out.printf("your average was a: %f and recieved an D in this class", average );
} else {
System.out.printf("your average was a: %f and recieved an F in this class", average );
}
}"
What is wrong with my code?
Also, how can I input this information: "
Write a program that ask the user to enter a list of positive scores until the user enters a negative score to terminate the input. You need to store these scores in an array. You can assume the maximum number of scores the user may enter is 50. However, you need to keep track of the actual number of scores entered. Write 2 methods:
1. calculateAverage(): this method takes the list of scores and return the average score.
2. countPerfectScores(): this method takes the list of scores and return the number of perfect scores (100).
The main program reads the input and calls these methods and print the results.
Please make sure you write a comment line to document what your method does."
![Test.java
+
1. import java.util.Compiler;
public class Test Score {
8
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25-
26
27 ▾
28
29 ▾
30
31 ▾
32
33▾
34
35
36
37
public static void main(String[]args) {
Scanner scan= new Scanner(System.in);
System.out.println("Enter the first exam Score: ");
Double Score1 = scan.nextDouble();
System.out.println("Enter the second exam Score: ");
Double Score2 = scan.nextDouble();
System.out.println("Enter the third exam Score: ");
Double Score3 = scan.nextDouble();
System.out.println("Enter the fourth exam Score: ");
Double Score4 = scan.nextDouble();
System.out.println("Enter the fifth exam Score: ");
Double Score5 = scan.nextDouble();
Double total = Scorel + Score2+ Score3 + Score4 + Score5
Double average = total/5;
if (average >= 90) {
System.out.printf("your average was a: %f and recieved an A in this Class", average );
} else if (average >= 80) {
System.out.printf("your average was a: %f and recieved an B in this class", average );
} else if (average >= 70) {
System.out.printf("your average was a: %f and recieved an C in this class", average );
else if (average >=60) {
System.out.printf("your average was a: %f and recieved an D in this class", average );
System.out.printf("your average was a: %f and recieved an F in this class", average );
} else {
}
Test Score.java /
STDIN
Input for the program (Optional)
Output:
Program did not output anything!
NEW
JAVA V
RUN ▶
:
זי](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbc0ef4be-b393-4fab-a253-cbedc48af370%2Fa5385d67-3607-470e-9054-edb6e7650587%2Fkl5m8vy_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

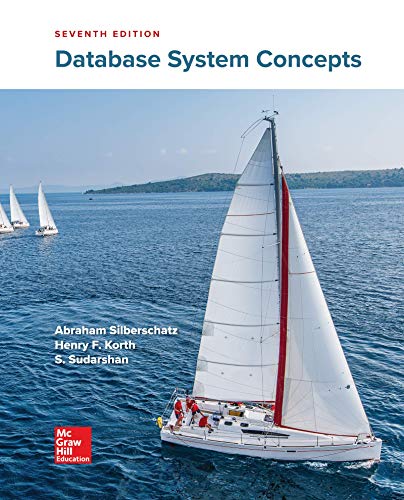
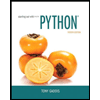
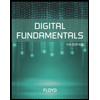
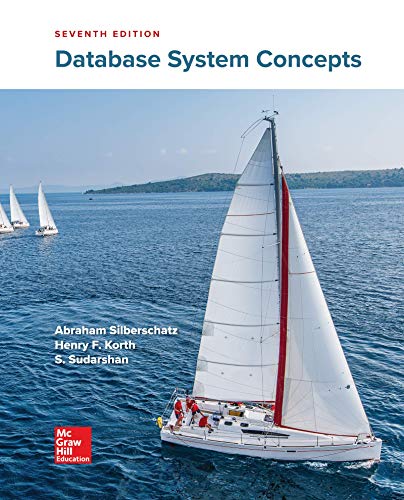
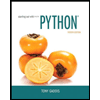
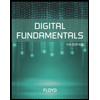
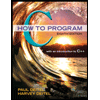
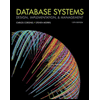
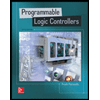