MIPS Simulator QtSpim: You are to have a complete program in MIPS assembly language that behaves exactly as the included C program. This program contains four functions in addition to the main() one. Your solution must contain all five C routines as they have been coded in the example. Make sure to run the program in MIPS and show the same output on MIPS as well to make sure there are no errors. Below is the five C routines and attached is the image of what the output must print out on QtSpim. #include int getMax(int arr[], int n) { int mx = arr[0]; for (int i = 1; i < n; i++) if (arr[i] > mx) mx = arr[i]; return mx; } void countSort(int arr[], int n, int exp) { int output[n]; int i, count[10] = { 0 }; for (i = 0; i < n; i++) count[(arr[i] / exp) % 10]++; for (i = 1; i < 10; i++) count[i] += count[i - 1]; for (i = n - 1; i >= 0; i--) { output[count[(arr[i] / exp) % 10] - 1] = arr[i]; count[(arr[i] / exp) % 10]--; } for (i = 0; i < n; i++) arr[i] = output[i]; } void radixSort(int arr[], int n) { int m = getMax(arr, n); for (int exp = 1; m / exp > 0; exp *= 10) countSort(arr, n, exp); } void printData(int arr[], int n) { for (int i = 0; i < n; i++) printf("%d \n", arr[i]); } int main() { int arr[] = {7, 9, 4, 3, 8, 1, 6, 2, 5}; int n = sizeof(arr) / sizeof(arr[0]); radixSort(arr, n); printData(arr, n); return 0; }
MIPS Simulator QtSpim: You are to have a complete program in MIPS assembly language that behaves exactly as the included C program. This program contains four functions in addition to the main() one. Your solution must contain all five C routines as they have been coded in the example. Make sure to run the program in MIPS and show the same output on MIPS as well to make sure there are no errors. Below is the five C routines and attached is the image of what the output must print out on QtSpim.
#include <stdio.h>
int getMax(int arr[], int n)
{
int mx = arr[0];
for (int i = 1; i < n; i++)
if (arr[i] > mx)
mx = arr[i];
return mx;
}
void countSort(int arr[], int n, int exp)
{
int output[n];
int i, count[10] = { 0 };
for (i = 0; i < n; i++)
count[(arr[i] / exp) % 10]++;
for (i = 1; i < 10; i++)
count[i] += count[i - 1];
for (i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
for (i = 0; i < n; i++)
arr[i] = output[i];
}
void radixSort(int arr[], int n)
{
int m = getMax(arr, n);
for (int exp = 1; m / exp > 0; exp *= 10)
countSort(arr, n, exp);
}
void printData(int arr[], int n)
{
for (int i = 0; i < n; i++)
printf("%d \n", arr[i]);
}
int main()
{
int arr[] = {7, 9, 4, 3, 8, 1, 6, 2, 5};
int n = sizeof(arr) / sizeof(arr[0]);
radixSort(arr, n);
printData(arr, n);
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

I got errors when I ran the code above. The tutor simply just pasted the image I posted. I need the actual code for MIPS that prints out the eact same output as it would print out in C. Give me the actual code for MIPS.
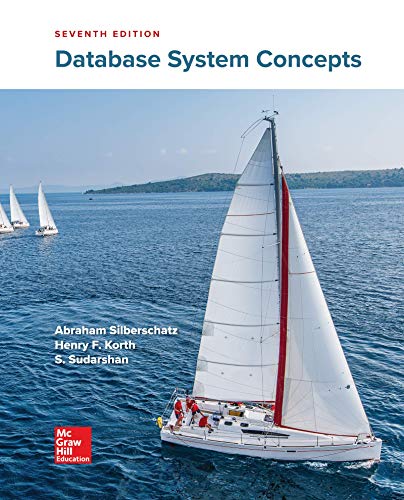
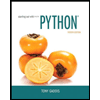
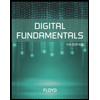
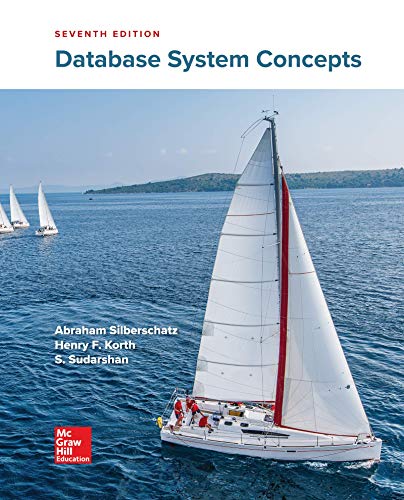
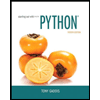
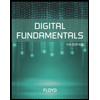
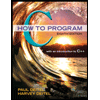
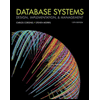
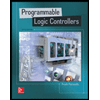