I am writing this program (in Java). The program takes input from user and accumulates and ending balance by making depostis or withdrawals. Inside of differentOptions method when the user enters option W for withdrawal, my code is nor working properly. If the amount entered is less than the ending balance the user should not be alow to do the withdrawal and there is supposed to be a deduction of $20 from the ending balance. However my code is allowing the user to do the withdrawal even when the balance is 0. In addition, in the same loop, I want to display a message that says "please try again " in case user enters for example a letter, but I am getting an error message. I copied and pasted my code here and also attached pictures. import java.util.*; public class AccountTransactionProject { public static void main(String[] args) { Scanner console= new Scanner(System.in); welcomeMenu(); differentOperations(); } public static void displayMenu() { // method that prints all the options of the main menu to the user System.out.println(); System.out.println("Enter your choice: "); System.out.println("\tB See Balance"); System.out.println("\tD Make Deposit"); System.out.println("\tW Make a Withdrawal"); System.out.println("\tQ Quit "); } public static char getInput() { // method that takes the first character of the option entered //from the displayMenu method and converts it to upper case Scanner console= new Scanner(System.in); String temp= console.next().toUpperCase(); return temp.charAt(0); } public static void welcomeMenu() { //method that welcomes the user and ask if they want to //continue using the program or exit it Scanner console= new Scanner(System.in); System.out.println("Hello! This program is going to help you to keep track " + "of your account balance.\nYou will be able to make deposits, " + "withdrawals and see you balance.\n"); System.out.println("Would you like to continue? Enter yes.\nNo help needed " + "at this time? Enter no.\n"); } public static void differentOperations() { /* method that takes answer from welcomeMethod and starts * taking data from user to do operations or quit the program * when requested by user * */ Scanner console= new Scanner(System.in); String option= console.next(); if(option.equalsIgnoreCase("no")){ System.out.println("You are exiting this program, see you later!"); } else { System.out.println("Lets get started!"); displayMenu(); char choiceEntered= getInput(); double endingBalance= 0; double balance= 0; double amount= 0; while(choiceEntered != 'Q') { //endingBalance= balance; if(choiceEntered == 'B') { System.out.println("Your ending balance is: " + balance); } else if(choiceEntered == 'D') { System.out.println("Make a deposit"); System.out.println("How much do you want to deposit? "); amount= console.nextDouble(); if(amount > 0) { balance += amount; } else { System.out.println("Invalid input, try again."); } } else if(choiceEntered== 'W') { System.out.println("Make a withdrawal"); System.out.println("Enter amount for withdrawal: "); double withDrawalAmount= console.nextDouble(); if(withDrawalAmount >= endingBalance) { endingBalance -= withDrawalAmount; System.out.println("Withdrawal approved. You are all set!"); }else if(withDrawalAmount < endingBalance) { System.out.println("Insuficient funds. Your withdrawal has been cancelled."); System.out.println("A $20 fee will be deducted from your balance."); endingBalance -= 20; }else { System.out.println("Invalid input. Please try again!"); } } else { System.out.println("Invalid input. Please try again!"); } displayMenu(); choiceEntered= getInput(); } System.out.println("You are exiting this program. See you later!"); } } }
I am writing this
Inside of differentOptions method when the user enters option W for withdrawal, my code is nor working properly. If the amount entered is less than the ending balance the user should not be alow to do the withdrawal and there is supposed to be a deduction of $20 from the ending balance. However my code is allowing the user to do the withdrawal even when the balance is 0. In addition, in the same loop, I want to display a message that says "please try again " in case user enters for example a letter, but I am getting an error message.
I copied and pasted my code here and also attached pictures.
import java.util.*;
public class AccountTransactionProject {
public static void main(String[] args) {
Scanner console= new Scanner(System.in);
welcomeMenu();
differentOperations();
}
public static void displayMenu() {
// method that prints all the options of the main menu to the user
System.out.println();
System.out.println("Enter your choice: ");
System.out.println("\tB See Balance");
System.out.println("\tD Make Deposit");
System.out.println("\tW Make a Withdrawal");
System.out.println("\tQ Quit ");
}
public static char getInput() {
// method that takes the first character of the option entered
//from the displayMenu method and converts it to upper case
Scanner console= new Scanner(System.in);
String temp= console.next().toUpperCase();
return temp.charAt(0);
}
public static void welcomeMenu() {
//method that welcomes the user and ask if they want to
//continue using the program or exit it
Scanner console= new Scanner(System.in);
System.out.println("Hello! This program is going to help you to keep track "
+ "of your account balance.\nYou will be able to make deposits, "
+ "withdrawals and see you balance.\n");
System.out.println("Would you like to continue? Enter yes.\nNo help needed "
+ "at this time? Enter no.\n");
}
public static void differentOperations() {
/* method that takes answer from welcomeMethod and starts
* taking data from user to do operations or quit the program
* when requested by user
* */
Scanner console= new Scanner(System.in);
String option= console.next();
if(option.equalsIgnoreCase("no")){
System.out.println("You are exiting this program, see you later!");
}
else {
System.out.println("Lets get started!");
displayMenu();
char choiceEntered= getInput();
double endingBalance= 0;
double balance= 0;
double amount= 0;
while(choiceEntered != 'Q') {
//endingBalance= balance;
if(choiceEntered == 'B') {
System.out.println("Your ending balance is: " + balance);
}
else if(choiceEntered == 'D') {
System.out.println("Make a deposit");
System.out.println("How much do you want to deposit? ");
amount= console.nextDouble();
if(amount > 0) {
balance += amount;
}
else {
System.out.println("Invalid input, try again.");
}
}
else if(choiceEntered== 'W') {
System.out.println("Make a withdrawal");
System.out.println("Enter amount for withdrawal: ");
double withDrawalAmount= console.nextDouble();
if(withDrawalAmount >= endingBalance) {
endingBalance -= withDrawalAmount;
System.out.println("Withdrawal approved. You are all set!");
}else if(withDrawalAmount < endingBalance) {
System.out.println("Insuficient funds. Your withdrawal has been cancelled.");
System.out.println("A $20 fee will be deducted from your balance.");
endingBalance -= 20;
}else {
System.out.println("Invalid input. Please try again!");
}
}
else {
System.out.println("Invalid input. Please try again!");
}
displayMenu();
choiceEntered= getInput();
}
System.out.println("You are exiting this program. See you later!");
}
}
}



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

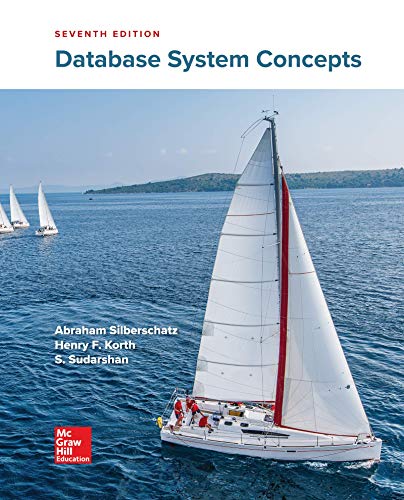
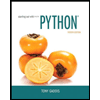
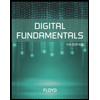
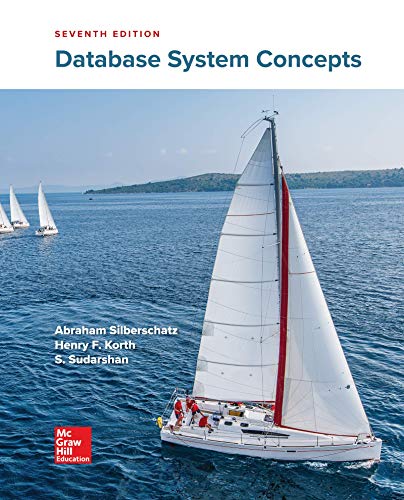
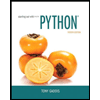
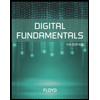
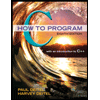
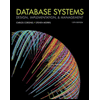
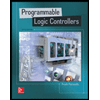