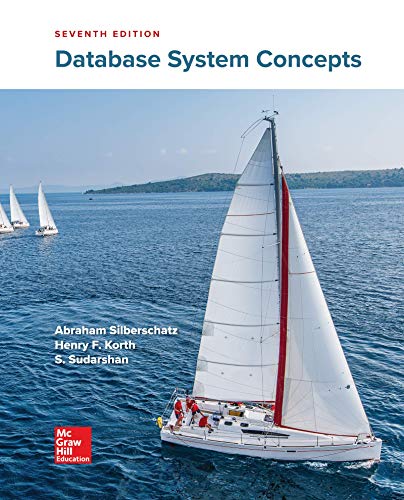
How to make this Python code run?
# RollDie.py
"""Graphing frequencies of die rolls with Seaborn."""
import matplotlib.pyplot as plt
import numpy as np
import random
import seaborn as sns
import sys
# use list comprehension to create a list of rolls of a six-sided die
rolls = [random.randrange(1, 7) for i in range(int(sys.argv[1]))]
# NumPy uniq nue function returns unique faces and frequency of each face
values, frequencies = np.unique(rolls, return_counts=True)
title = f'Rolling a Six-Sided Die {len(rolls):,} Times'
sns.set_style('whitegrid') # white backround with gray grid lines
axes = sns.barplot(values, frequencies, palette='bright') # create bars
axes.set_title(title) # set graph title
axes.set(xlabel='Die Value', ylabel='Frequency') # label the axes
# scale y-axis by 10% to make room for text above bars
axes.set_ylim(top=max(frequencies) * 1.10)
# display frequency & percentage above each patch (bar)
for bar, frequency in zip(axes.patches, frequencies):
text_x = bar.get_x() + bar.get_width() / 2.0
text_y = bar.get_height()
text = f'{frequency:,}\n{frequency / len(rolls):.3%}'
axes.text(text_x, text_y, text,
fontsize=11, ha='center', va='bottom')
plt.show() # display graph

You need to call the script RollDie.py from command line as shown below
In the directory where the script is saved, open command line, then type
python3 RollDie.py 1000
Step by stepSolved in 2 steps with 1 images

- ive try this code and did the inputs and its not right . can someone help me ,, fullName=str(input())##List is for storing firstname,middlename,lastname separately,List is initially an empty listList=[]##Take an empty string wordword=""##Start traversing fullNamefor char in fullName: ##If any character is a space(' ') then,add the string stored in word variable in List if(char==' '): List.append(word) ##And again make word an empty string word="" ##Otherwise,add current character with word else: word=word+char ##After completing this for loop,add the string stored in word variable in ListList.append(word)##result will store the final result,it is initially an empty stringresult=""##If there are only firstname and lastname then,if(len(List)==2): ##Add lastname then add ", " then add first character of firstname then add ".", with result result=List[1]+", "+List[0][0]+"."##If there are firstname,middlename and lastname…arrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardPlease send the code text Thank you.arrow_forward
- In the following program skeleton, replace the < insert code here> line with theappropriate code. The resulting program should display the initial list of songs,prompt the user for a string of text to be replaced and then prompt for new text toreplace it. After making the replacement, it should display the updated list of songs.import java.util.Scanner;public class UpdateSongs{public static void main ( String [] args){Scanner stdIn = new Scanner(System.in);String songs =“ 1. Welcome to Your Life – Grouplove\n” +“ 2. Sedona – Houndmouth\n” +“3. Imagine – John Lennon\n” +“4. Bohemian Rhapsody –Queen\n”;String oldText, newText;< insert code here>}}Sample Session:1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Lennon4. Bohemian Rhapsody –QueenEnter text to replace: LennonEnter new text: Dean1. Welcome to Your Life – Grouplove”2. Sedona – Houndmouth3. Imagine – John Dean4. Bohemian Rhapsody –Queenarrow_forwardIn Python: Create a function Matrix_transpose() utilizing List comprehension techniques that takes a 2d list containing user generated/input elements of type int as its argument then returns the reference to a new 2d list that is the transpose of the original. Assume the matrix is rectangular. Show the funtion works with different values. Do not use any python packages to create the funtion.arrow_forwardplease use your own code and just a picturearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
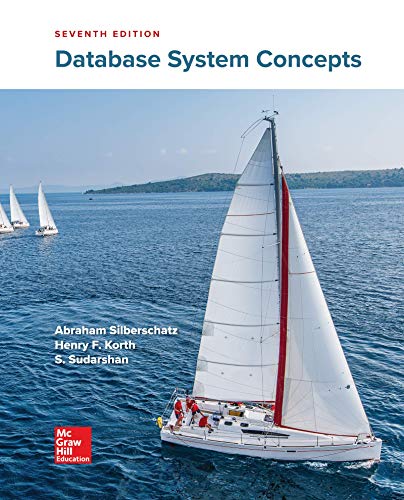
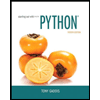
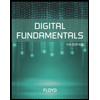
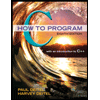
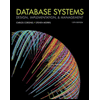
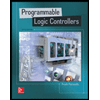