How to find the largest number in java?
How to find the largest number in java?

Programing instructions:
- Create a class.
- Create a function getLargest() to find the largest number from the array.
- In the main, create an array.
- Call the function and print the value returned by the function.
Program:
//Class
public class Example{
//Method to find the largest element
public static int getLargest(int[] a, int total){
//Assign value of first element in the largest variable.
int largest = a[0];
//Use loop to traverse the array
for (int i = 1; i < total; i++)
{
//Check whether the current element is greater than the value stored in the largest
if (a[i] > largest)
{
//Assign the value of current element in the largest
largest = a[i];
}
}
//Return the largest
return largest;
}
//main method
public static void main(String args[]){
//Array
int a[]={44,66,99,77,33,22,55};
//Size of the array
int size = a.length;
//Call the function and print the value returned by the function
System.out.println("Largest: "+ getLargest(a,size));
}}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

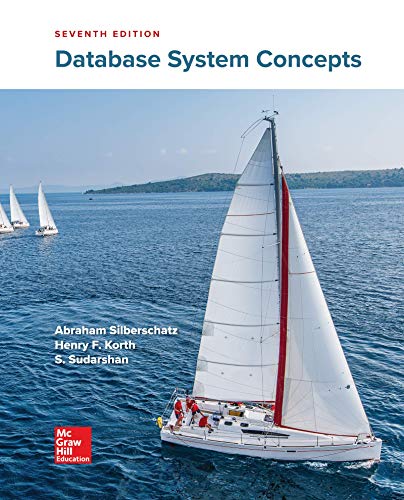
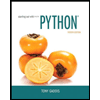
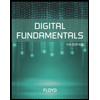
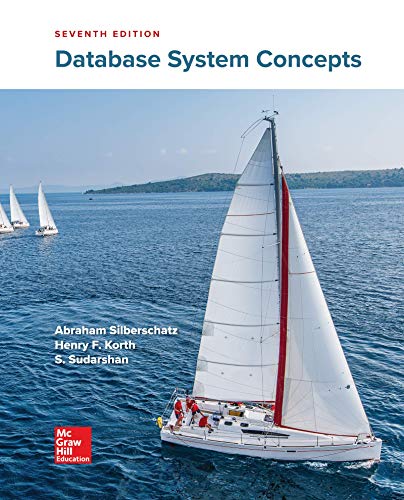
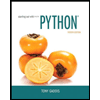
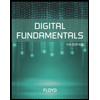
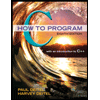
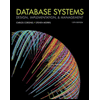
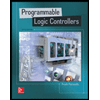