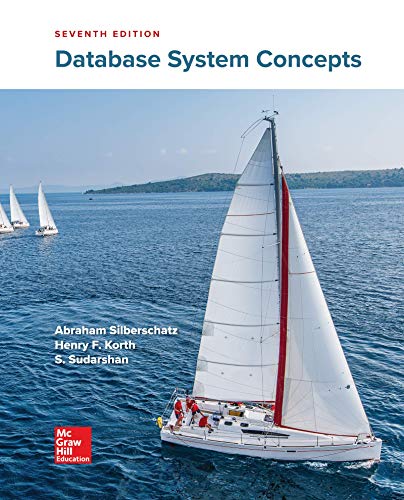
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
How many squares are drawn if foo(0,0,5,2) is called?
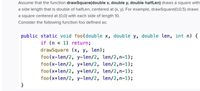
Transcribed Image Text:The provided text describes a function in an educational context regarding computer programming and graphics.
---
**Explanation of the Function:**
The text defines a recursive function `foo` that uses an auxiliary function called `drawSquare`. The `drawSquare(double x, double y, double halfLen)` function is responsible for drawing a square centered at coordinates `(x, y)` with a side length that is double the given `halfLen`. For instance, `drawSquare(0,0,5)` would draw a square centered at the origin `(0,0)` with a side length of `10`.
**Detailed Breakdown of the Code:**
```java
public static void foo(double x, double y, double len, int n) {
if (n < 1) return;
drawSquare(x, y, len);
foo(x - len/2, y - len/2, len/2, n - 1);
foo(x - len/2, y + len/2, len/2, n - 1);
foo(x + len/2, y + len/2, len/2, n - 1);
foo(x + len/2, y - len/2, len/2, n - 1);
}
```
**Function Behavior:**
- **Base Case:** The recursion stops when `n < 1`. In this case, the function simply returns without doing anything.
- **Recursive Case:**
- First, it draws a square centered at `(x, y)` with side length `len`.
- Then, it makes four recursive calls to itself, each time subdividing the current square into four smaller squares and recentering.
- The square's center for the recursive calls is adjusted by half of the current length (`len/2`) to cover all four quadrants:
- Bottom-left
- Top-left
- Top-right
- Bottom-right
This method is often used to create fractal patterns or divide graphical space efficiently.
---
This explanation provides a detailed view of the recursive structure and graphical operations performed by the `foo` function, essential for understanding recursion and graphical transformations in programming.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- You are given a 2 by n grid, where the cell on row i column j contains a non-negative number ai,j . You can start at either cell in the lefttmost column, and your goal is to reach either cell in the rightmost column by a sequence of moves. You can move to an adjacent cell (if it exists) in each of the 4 cardinal directions (up,down, left and right). A path achieves a score equal to the sum of values in its cells. Note that a cell which is used twice in a path only counts its value once to the score of that path.Design an algorithm which runs in O(n) time and finds a path of minimum score from the leftmost column to the rightmost column.arrow_forwardWhat is the worst case running time for finding the kth value in an array?arrow_forwardThis challenge is based on the classic videogame "Snake". Assume the game screen is an n * n square, and the snake starts the game with length 1 (i.e. just the head) positioned on the top left corner. For example, if n = 7 the game looks something like this: BEGINNING In this version of the game, the length of the snake doubles each time it eats food (e.g. if the length is 4, after eating it becomes 8). Create a function that takes the siden of the game screen and returns the number of times the snake can eat before it runs out of space in the game screen. Examples FIRST MOVE snakefill (3) snakefill (6) - 5 snakefill (24) → 9 3arrow_forward
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
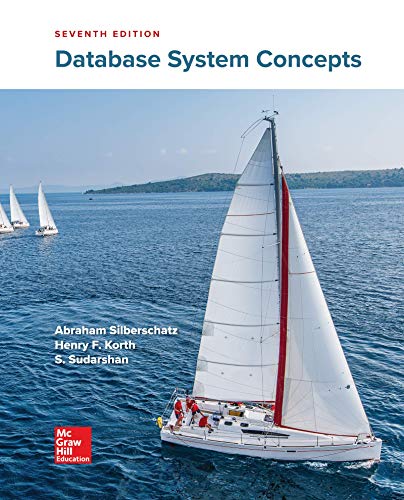
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
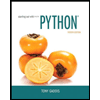
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
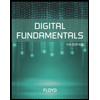
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
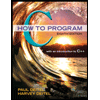
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
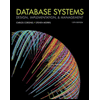
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
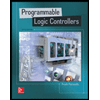
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education