ent and demonstrate a disk-based buffer pool class based on the LRU buffer pool replacement strategy. Disk blocks are numbered consecutively from the beginning of the file with the first block numbered as 0. Assume that blocks are 4096 bytes in size. Use the supplied C++ files to implement your LRU Buffer Pool based on the instructions below. Implement a BufferBlock class using the supplied BufferBlockADT.h Your Buffer Block must inherit BufferBlockADT or you will not get credit for your work. All BufferBlockADT virtual functions mus
Implement and demonstrate a disk-based buffer pool class based on the LRU buffer pool replacement strategy. Disk blocks are numbered consecutively from the beginning of the file with the first block numbered as 0. Assume that blocks are 4096 bytes in size. Use the supplied C++ files to implement your LRU Buffer Pool based on the instructions below.
- Implement a BufferBlock class using the supplied BufferBlockADT.h
- Your Buffer Block must inherit BufferBlockADT or you will not get credit for your work.
- All BufferBlockADT virtual functions must be implemented in BufferBlock
- Block Size: 4096 bytes
- Add an instance variable to your buffer block implementation to store the block id; i.e., “int blockID.”
- Implement a Buffer Pool by inheriting BufferPoolADT (BufferPoolADT.h) – implement all of BufferPoolADT’s functions
- Your buffer pool should consist of 5 buffer blocks
- Your buffer pool should manage the buffers using the LRU strategy
- Your buffer pool should be named LRUBufferPool and the file containing the LRUBufferPool class should be named LRUBufferPool.h
- Use the provided main.cpp and the included test file mydatafile.txt to test your program.
/* Assuming you initialize your buffer pool with blocks 0-4 and
* open the test file as a binary file, your output for the first
* three tests (file position 5030, 16500, and 24640) should look
* like the following:
*/
My data for block 1 is: "ment all o"
My buffer block order from most recently used to LRU is:
1, 0, 2, 3, 4,
My data for block 4 is: "e for the "
My buffer block order from most recently used to LRU is:
4, 1, 0, 2, 3,
My data for block 6 is: "ent a Buff"
My buffer block order from most recently used to LRU is:
6, 4, 1, 0, 2,
![E#include "constants.h"
#include "LRUBufferPool.h"
using namespace std;
Bint main() {
//initialize buffer pool
LRUBufferPool* bp = new LRUBufferPool("mydatafile.txt", POOL_SIZE, BLOCKSIZE);
//get data from the buffer
char* data = new char[10];
bp->getBytes (data, 10, 5030);
printchars(data, 10, 5030/BLOCKSIZE);
bp->printBufferBlockorder ();
/*Output should be something like the following:
My data for block 1 is: "ment all o"
My buffer block order from most recently used to LRU is:
1, 0, 2, 3, 4,
//re-initialize the char array and get the next block of data
initializecharArray(10, data);
bp->getBytes (data, 10, 16500);
printchars (data, 10, 16500/BLOCKSIZE);
bp->printBufferBlockorder ();
/*Output should be something like the following:
My data for block 4 is: "e for the
My buffer block order from most recently used to LRU is:
4, 1, 0, 2, 3,
*/
//re-initialize the char array and get the next block of data
initializecharArray(10, data);
bp->getBytes (data, 10, 24640);
printchars(data, 10, 24640/BLOCKSIZE);
bp- >printBufferBlockorder();
/*Output should be something like the following:
My data for block 6 is: "ent a Buff"
My buffer block order from most recently used to LRU is:
6, 4, 1, 0, 2,
* /
//re-initialize the char array and get the next block of data
initializecharArray(10, data);
bp->getBytes(data, 10, 28700);
printchars (data, 10, 28700/BLOCKSIZE);
bp->printBufferBlockOrder ();
//re-initialize the char array and get the next block of data
initializecharArray(10, data);
bp->getBytes(data, 10, 16600);
printchars(data, 10, 16600/BLOCKSIZE);
bp->printBufferBlockOrder ();
//close program
cout <« endl << endl;
system("pause");
return 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F546860fe-98c5-4cbb-83c9-6bb3ac138cc4%2F365fb89c-0a25-4809-96d7-a6834fb36f49%2Fem6sr3a_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

How do you implement the BufferBlock class and the LRUBufferPool class?
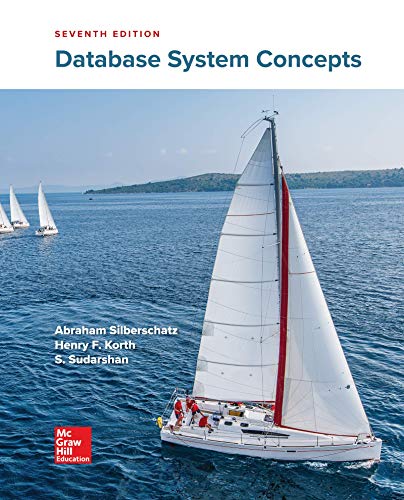
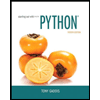
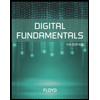
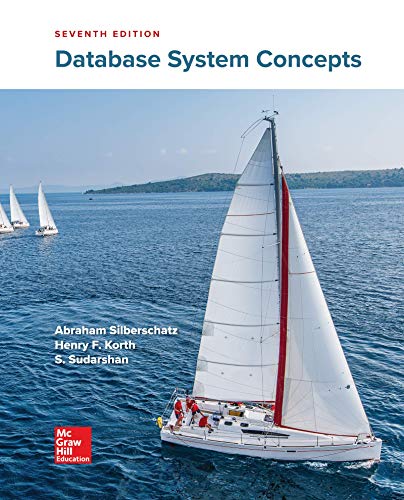
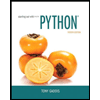
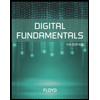
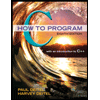
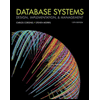
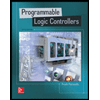