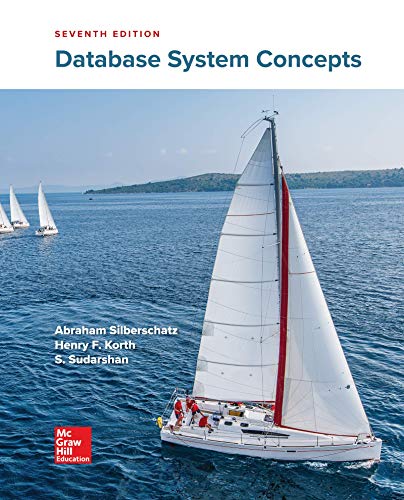
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
How do I turn this code into a flowchart?
Python code:
#defining the tuples
tup1 = ("$")
tup2 = ("$")
tup3 = ("PAYROLL TRANSACTIONS")
#creating the first function to creat the heading "PAYROLL TRANSACTIONS"
def print_heading():
print("\t","\t", tup1, tup3, tup2, "\t","\t")
print("\n")
return
#calling the function that was just made
print_heading()
#creating the second function to make a line between the heading and the data, making the chart look cleaner (this function will be called later)
def break_line():
print("_____________________________________________________")
print("\n")
return
#opening the employees file and calling it "Filea"
Filea = open('Employees.csv')
#reading the lines and copying them to a new variable, emp index 0
a = Filea.readlines()
aHeading = a[0]
#delete first line from line list we just made
del a[0]
#split is dividing this string into a list, making our columns and rows
aHeading = aHeading.split(",")
#opening the timesheet file and doing the same thing we just did above for this file
timeFile = open('Timesheets.csv')
time = timeFile.readlines()
timeHeading = time[0]
del time[0]
timeHeading = timeHeading.split(",")
#making am employee info list
aInfo= []
#for loop
for row in range(len(a)):
#removing new line from the end of each row
data = a[row]
#spliting all of the data into a list
data = data.split(",")
timeData = time[row]
timeData = timeData.split(",")
#appending/putting together the data . timedata has the hours and data has the other info
thechart = []
thechart.append(data[0])
thechart.append(data[1])
thechart.append(data[2])
thechart.append(timeData[1])
thechart.append(data[4])
#calculating the gross pay using the data we just put together in the last group of code
if(data[5] == 'Y'):
gpay = (40*float(data[4]))+((float(timeData[1]) - 40)*float(data[4])*1.5)
else:
gpay = float(timeData[1]) * float(data[4])
thechart.append(gpay)
#adding employee data into the chart!
aInfo.append(thechart)
#printing the format
print("Emp No","First","\t"," Last","\t", " Hours","Wage","Gross Pay")
#calling break line function from beginning
break_line()
#print all employee information
for e in aInfo:
print("{:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- # Question 4def costume_rating(costume_color, bling_value): """ Returns a function that calculates the number of candies to return based on the costume color, bling value, and phrase length. Args: costume_color (str): The color of your costume. Must be one of 'blue', 'red', 'green', 'yellow', 'purple', or 'orange'. bling_value (int): Represents how shiny your costume is. Must be a positive integer. Returns: A function that takes in one parameter: phrase (str): The phrase said by the trick-or-treater. >>> costume_rater = costume_rating('blue', 5) >>> costume_rater('19 Character Phrase') 85 >>> costume_rater('e') 0 >>> costume_rater('seven c') 1 >>> costume_rater = costume_rating('orange', 8) >>> costume_rater('hello i want candy') 14 >>> costume_rater = costume_rating('yellow', 5) >>> costume_rater('please give me…arrow_forward/* Programming concept: structures Program should: be a C program, define a structure to store the year, month, day, and high temperature. write a function get_data that has a parameter that is a pointer to a struct and reads from the user into the struct pointed to by the parameter. write a function print_data that takes the struct pointer as a parameter and prints the data. in main, declare a struct, call the get_data function, call the print_data function. */ #include <stdio.h> int main(int argc, char *argv[]) { return 0;}arrow_forwardC#(Sharp): I made my code and design below. Anybody can help me some correction and add some design button and code. "Need to make C# step by step design and code "Calculator where make a calculator program that can do add, subtract, multiply, divide and square root. It should have a memory save/restore function for one number. There should be a way to set the number of fraction digits displayed". Code: using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms; namespace AktCalc { public partial class Form1 : Form { string last = ""; bool minus = false; bool plus = false; bool divide = false; bool multiply = false; string memory = ""; public Form1() { InitializeComponent(); } private void button2_Click(object sender, EventArgs e) { if (textBox1.Text.Contains(",")) { return; } else { textBox1.Text += ","; } } private void…arrow_forward
- Data Management Functions - Name format. Python*a) Define a function nameFormat() with parameters first, middle, and last.-This function prints the first name, the middle initial and the last name using proper title format.-Call the function nameFormat with these positional arguments: john stu smith-Call the function nameFormat with these keyword arguments: last=‘kennedy’, first=‘john’, middle=‘fitzgerald’ Example Output John S. SmithJohn F. Kennedyarrow_forward# Exercise 8: Create a function that takes the sum of three numbers and returns the value # Make sure to use the function and display the result # When you are done, get 3 numbers from a user and then print out the sum using your functionarrow_forwardC# languagearrow_forward
- program Credit Card Validator - Takes in a credit card number from a common credit card vendor (Visa, MasterCard, American Express, Discover) and validates it to make sure that it is a valid number (look into how credit cards use a checksum). please use #include <iostream>arrow_forwardlanguage = pythonarrow_forwardInstructions The python "try" keyword is very powerful in that it can, among other things, prevent a program from ending abnormally because of invalid numeric input. Write a python program with two functions/modules that does the following: .main() accepts input and calls a function to test if the input is a number and displays a message regarding the result of that numeric test • numTest() is passed an input string, tests to see if the string is numeric and returns the necessary information to main() . a NULL input (just pressing the enter key) ends the program . DO NOT USE THE BUILTIN PYTHON FUNCTION FOR NUMERIC TESTING Be sure to use clear prompts/labeling for input and output.arrow_forward
- Don't use ai Add explanationarrow_forward// LargeSmall.cpp - This program calculates the largest and smallest of three integer values. #include <iostream> using namespace std; int main() { // This is the work done in the housekeeping() function // Declare and initialize variables here int largest; // Largest of the three values int smallest; // Smallest of the three values // Prompt the user to enter 3 integer values // Write assignment, add conditional statements here as appropriate // This is the work done in the endOfJob() function // Output largest and smallest number. cout << "The largest value is " << largest << endl; cout << "The smallest value is " << smallest << endl; return 0; }arrow_forwardpractice python part 3arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
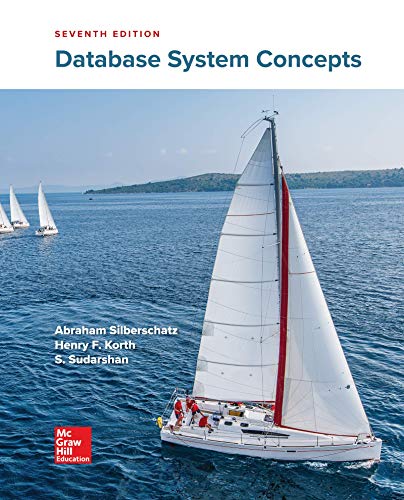
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
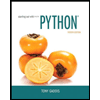
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
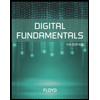
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
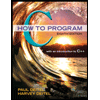
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
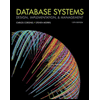
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
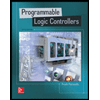
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education