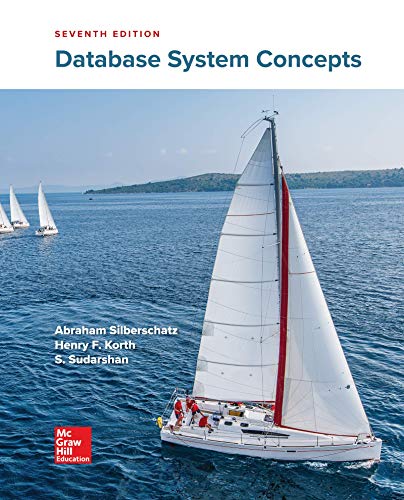
Concept explainers
Please help me fix the errors, I've been try to fix it but it gives me new errors. I can only edit the code in studentMain.cpp, I cannot edit the code in bubble.cpp, bubble.h, and student.h.
//bubble.cpp
#include <stdlib.h>
#include "student.h"
void bubble(student *array[], int size)
{
int x;
int y;
student *temp = NULL;
for(x = 0; x < size; x++) {
for(y = 0; y < size-1; y++) {
if(array[y]->mean > array[y+1]->mean) {
temp = array[y+1];
array[y+1] = array[y];
array[y] = temp;
}
}
}
return;
}
//bubble.h
void bubble(student *array[], int size);
//student.h
typedef struct student_info{
char *first;
char *last;
int exam1;
int exam2;
int exam3;
float mean;
} student;
//studentMain.cpp
#include <stdio.h>
#include <stdlib.h>
#include "student.h"
#include "bubble.h"
int main()
{
// Create an array of 19 student pointers
student *students[19];
// Allocate space for each student in the array
for (int i = 0; i < 19; i++) {
students[i] = (student *)malloc(sizeof(student));
}
// Read in the data from the file
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
for (int i = 0; i < 19; i++) {
fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3);
// Calculate the student's mean
students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0;
}
fclose(fp);
// Sort the students using the bubble sort
bubble(students, 19);
// Find the mean, minimum, maximum, and median of the grades
float mean = 0.0;
float min = students[0]->mean;
float max = students[18]->mean;
float median = students[9]->mean;
for (int i = 0; i < 19; i++) {
mean += students[i]->mean;
}
mean /= 19.0;
// Print the class statistics
printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median);
// Print the student averages
for (int i = 0; i < 19; i++) {
printf("%s %s %f\n", students[i]->first, students[i]->last);
printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean);
}
// Free the allocated memory for each student
for (int i = 0; i < 19; i++) {
free(students[i]);
}
return 0;
}
![studentMain.cpp: In function 'int main()':
studentMain.cpp:40:20: warning: format 'f' expects a matching 'double' argument
printf("%s %s f\n", students[i]->first, students[i]->last);
[-Wformat=]
40 |
I
|
|
double
bubble.h:1:13: error: variable or field 'bubble' declared void
1 | void bubble (student *array[], int size);
برای ما برای ار برس دار
bubble.h:1:13: error: 'student' was not declared in this scope
bubble.h:1:22: error: 'array' was not declared in this scope
1 | void bubble (student *array[], int size);
bubble.h:1:28: error: expected primary-expression before ¹]' token
1 | void bubble (student *array[], int size);
bubble.h:1:31: error: expected primary-expression before 'int'
1 | void bubble (student *array[], int size);
I
الای ارسی مالی](https://content.bartleby.com/qna-images/question/f76fb667-0622-451e-86fc-d3866765f8e5/9e8d027e-7a21-45bf-aa68-6b959e726bf6/5tgp4y_thumbnail.png)

Hello! I understand that diving into code can sometimes be overwhelming, especially when you're faced with error messages that might seem cryptic. However, no worries – we'll work through them step by step. Your code consists of the main student file, a utility for the bubble sort, and two headers that structure the functionalities. Let's systematically identify the issues and rectify them in studentMain.cpp
.
Step by stepSolved in 3 steps

Hi, it is still giving me the same 5 errors as last time even after modifying the code. I tried reordering #include "student.h" and #include "bubble.h" in studentMain.cpp but it gave me more errors. Is this happening because I am compiling with the wrong command, I am compiling it all together like this: g++ studentMain.cpp bubble.cpp bubble.h student.h grades.txt. I have the modified code below and a reminder that I can only edit the code in the studentMain.cpp file:
//studentMain.cpp
#include <stdio.h>
#include <stdlib.h>
#include "student.h"
#include "bubble.h"
int main()
{
// Create an array of 19 student pointers
student *students[19];
// Allocate space for each student in the array
for (int i = 0; i < 19; i++) {
students[i] = (student *)malloc(sizeof(student));
}
// Read in the data from the file
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
for (int i = 0; i < 19; i++) {
fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3);
// Calculate the student's mean
students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0;
}
fclose(fp);
// Sort the students using the bubble sort
bubble(students, 19);
// Find the mean, minimum, maximum, and median of the grades
float mean = 0.0;
float min = students[0]->mean;
float max = students[18]->mean;
float median = students[9]->mean;
for (int i = 0; i < 19; i++) {
mean += students[i]->mean;
}
mean /= 19.0;
// Print the class statistics
printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median);
// Print the student averages
for (int i = 0; i < 19; i++) {
printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean);
}
// Free the allocated memory for each student
for (int i = 0; i < 19; i++) {
free(students[i]);
}
return 0;
}
//bubble.cpp
#include <stdlib.h>
#include "student.h"
void bubble(student *array[], int size)
{
int x;
int y;
student *temp = NULL;
for(x = 0; x < size; x++) {
for(y = 0; y < size-1; y++) {
if(array[y]->mean > array[y+1]->mean) {
temp = array[y+1];
array[y+1] = array[y];
array[y] = temp;
}
}
}
return;
}
//bubble.h
void bubble(student *array[], int size);
//student.h
typedef struct student_info{
char *first;
char *last;
int exam1;
int exam2;
int exam3;
float mean;
} student;
//grades.txt
CSCE1040
Erica Sanders 75 89 67
Kelley Cummings 74 70 79
Jamie Reynolds 64 52 66
Shawna Huff 80 88 61
Muriel Holmes 81 74 79
Marion Harmon 77 64 69
Catherine Moss 51 80 73
Kristin Fernandez 86 69 81
Elsa Alvarado 63 77 67
Cora Spencer 76 79 71
Valerie Olson 85 78 79
Anne Singleton 85 87 65
Rene Boone 85 85 77
James Morgan 69 86 51
Cedric Haynes 72 73 88
Elijah Snyder 65 92 91
Roger Howard 79 95 71
Archie Black 70 81 63
Melvin Watkins 66 67 72
![bubble.h:1:13: error: variable or field 'bubble' declared void
1 | void bubble (student *array[], int size);
م م م م کی برسی مالی
bubble.h:1:13: error: 'student' was not declared in this scope
bubble.h:1:22: error: 'array' was not declared in this scope
1 | void bubble (student *array[], int size);
عا م کی برسی مالی
bubble.h:1:28: error: expected primary-expression before ¹]' token
1 | void bubble (student *array[], int size);
I
bubble.h:1:31: error: expected primary-expression before 'int'
1 | void bubble (student *array[], int size);
I](https://content.bartleby.com/qna-images/question/f76fb667-0622-451e-86fc-d3866765f8e5/e935ebba-2484-472a-ab97-32141dcbefd5/5312jt_thumbnail.png)
Hi, it is still giving me the same 5 errors as last time even after modifying the code. I tried reordering #include "student.h" and #include "bubble.h" in studentMain.cpp but it gave me more errors. Is this happening because I am compiling with the wrong command, I am compiling it all together like this: g++ studentMain.cpp bubble.cpp bubble.h student.h grades.txt. I have the modified code below and a reminder that I can only edit the code in the studentMain.cpp file:
//studentMain.cpp
#include <stdio.h>
#include <stdlib.h>
#include "student.h"
#include "bubble.h"
int main()
{
// Create an array of 19 student pointers
student *students[19];
// Allocate space for each student in the array
for (int i = 0; i < 19; i++) {
students[i] = (student *)malloc(sizeof(student));
}
// Read in the data from the file
FILE *fp = fopen("students.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
for (int i = 0; i < 19; i++) {
fscanf(fp, "%s %s %d %d %d", students[i]->first, students[i]->last, &students[i]->exam1, &students[i]->exam2, &students[i]->exam3);
// Calculate the student's mean
students[i]->mean = (students[i]->exam1 + students[i]->exam2 + students[i]->exam3) / 3.0;
}
fclose(fp);
// Sort the students using the bubble sort
bubble(students, 19);
// Find the mean, minimum, maximum, and median of the grades
float mean = 0.0;
float min = students[0]->mean;
float max = students[18]->mean;
float median = students[9]->mean;
for (int i = 0; i < 19; i++) {
mean += students[i]->mean;
}
mean /= 19.0;
// Print the class statistics
printf("CSCE 1040 MEAN %.2f MIN: %.2f MAX: %.2f MEDIAN: %.2f\n", mean, min, max, median);
// Print the student averages
for (int i = 0; i < 19; i++) {
printf("%s %s %f\n", students[i]->first, students[i]->last, students[i]->mean);
}
// Free the allocated memory for each student
for (int i = 0; i < 19; i++) {
free(students[i]);
}
return 0;
}
//bubble.cpp
#include <stdlib.h>
#include "student.h"
void bubble(student *array[], int size)
{
int x;
int y;
student *temp = NULL;
for(x = 0; x < size; x++) {
for(y = 0; y < size-1; y++) {
if(array[y]->mean > array[y+1]->mean) {
temp = array[y+1];
array[y+1] = array[y];
array[y] = temp;
}
}
}
return;
}
//bubble.h
void bubble(student *array[], int size);
//student.h
typedef struct student_info{
char *first;
char *last;
int exam1;
int exam2;
int exam3;
float mean;
} student;
//grades.txt
CSCE1040
Erica Sanders 75 89 67
Kelley Cummings 74 70 79
Jamie Reynolds 64 52 66
Shawna Huff 80 88 61
Muriel Holmes 81 74 79
Marion Harmon 77 64 69
Catherine Moss 51 80 73
Kristin Fernandez 86 69 81
Elsa Alvarado 63 77 67
Cora Spencer 76 79 71
Valerie Olson 85 78 79
Anne Singleton 85 87 65
Rene Boone 85 85 77
James Morgan 69 86 51
Cedric Haynes 72 73 88
Elijah Snyder 65 92 91
Roger Howard 79 95 71
Archie Black 70 81 63
Melvin Watkins 66 67 72
![bubble.h:1:13: error: variable or field 'bubble' declared void
1 | void bubble (student *array[], int size);
م م م م کی برسی مالی
bubble.h:1:13: error: 'student' was not declared in this scope
bubble.h:1:22: error: 'array' was not declared in this scope
1 | void bubble (student *array[], int size);
عا م کی برسی مالی
bubble.h:1:28: error: expected primary-expression before ¹]' token
1 | void bubble (student *array[], int size);
I
bubble.h:1:31: error: expected primary-expression before 'int'
1 | void bubble (student *array[], int size);
I](https://content.bartleby.com/qna-images/question/f76fb667-0622-451e-86fc-d3866765f8e5/e935ebba-2484-472a-ab97-32141dcbefd5/5312jt_thumbnail.png)
- Step 1: Read your files! You should now have 3 files. Read each of these files, in the order listed below. staticarray.h -- contains the class definition for StaticArray, which makes arrays behave a little more like Python lists O In particular, pay attention to the private variables. Note that the array has been defined with a MAX capacity but that is not the same as having MAX elements. Which variable indicates the actual number of elements in the array? staticarray.cpp -- contains function definitions for StaticArray. Right now, that's just the print() member function. 0 Pay attention to the print() function. How many elements is it printing? main.cpp -- client code to test your staticarray class. Note that the multi-line comment format (/* ... */) has been used to comment out everything in main below the first print statement. As you proceed through the lab, you will need to move the starting comment (/*) to a different location. Other than that, no changes ever need to be made to…arrow_forwardAlert dont submit AI generated answer.arrow_forwardCan you help me please: have the program know when numbers out of the array boundaries are received and mitigate those problems. The given code intentionally induces numbers out of bounds and the student’s portion must correct for this. As always use a header file and implementation file to do those calculations. Remember not to change the given code below in any way Design and implement the class myArray that solves the array index out of bounds problem and also allows the user to begin the array index starting at any integer, positive or negative. Every object of type myArray is an array of type int. During execution, when accessing an array component, if the index is out of bounds, the program must terminate with an appropriate error message. Consider the following statements:myArray list(5); //Line 1 myArraymyList(2, 13); //Line 2myArray yourList(-5, 9); //Line 3The statement in Line 1 declares list to be an…arrow_forward
- Bonus Question: For the same array as in the last question, explain what this statement does: x = &x[2]; Hint: draw a memory diagram to help yourself find the correct answer.arrow_forwardIn javacode: Use ArrayList to create an array called myAL of type integer. --Fill the array with the values 5, 10, 15, 22, 33. --Print the array (use enhanced for loop). --Insert the value 25 between 10 and 15 and print the array.--Remove 2 elements on index 1 and 3 and then print the array.--Print if the array contains the value 123 or not.--Print the index of the element 22.--Print the size of the array.arrow_forwardPart 2: Coding 1. Write a complete Java program named FindAverage that contains the following: a. A main method that asks the user to provide the number of rows and columns for a 2- dimensional array of integers. b. A main method calls the getArray() method that creates the 2D array of specified size and populates it with random values from 0 to 100. c. A main method prints the elements of the 2D array created by getArray(). d. A main method calls the printAverage method that will: i. Receive the two-dimensional array as input ii. Calculate the average of elements in this array iii. Display the average on the console. The average of all elements should be formatted as xxx.XX. Hint: you can print the 2D array at any point in the program using either traditional for loop, foreach loop or available methods in java.util package. e. Add comments to your program. Start your program with the following header: *** *** ***** /**** ***arrow_forward
- Please assist with the attached question using Java language. Thanks.arrow_forwardJava programming help please. Main and other class of code is below. Thank you so so much. i have to Write a program that does the following: You have an array of integers with positive and negative values. using one ourArray object (will post code of "ourArrary" below) , and in two loops maximum, How can you reorganize the content of the array so that all negative numbers are on one side and the positive numbers are on the other side. [-3, 2, -4, 5, -6, -8,-9, 7, 13] The output should be [-3, -4, -6, -8, -9, 2, 5, 7, 13] Our array code: public class ourArray<T> implements iQueue{ private T[] Ar; private int size = 0; private int increment = 100; //default constructor @SuppressWarnings("unchecked") public ourArray () { Ar = (T[]) new Object[100]; size = 0; } //constructor that accepts the size @SuppressWarnings("unchecked") public ourArray (int n) { Ar = (T[]) new Object[n]; size = 0; } //constructor that specifies the initial capacity and the increment…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
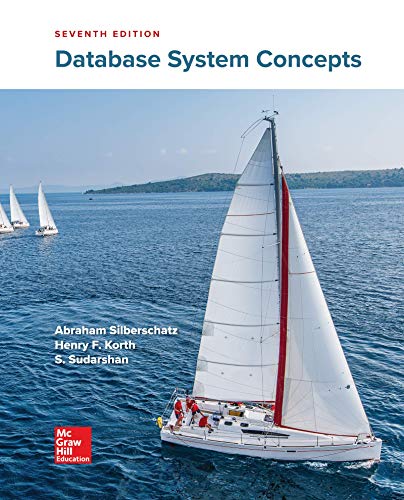
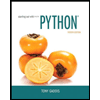
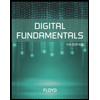
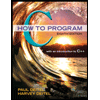
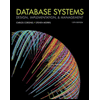
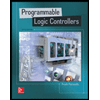