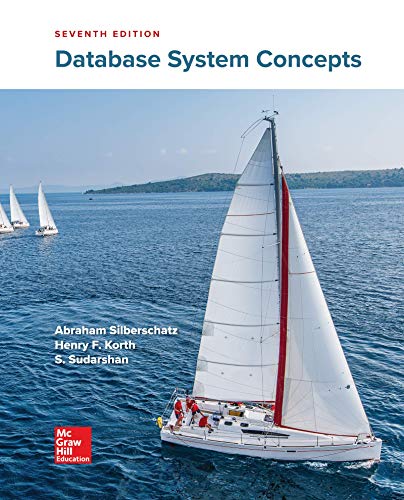
Concept explainers
Hi, I need some help with modifying this game. Due to the limit of 5,000 characters, I am unable to provide the proper indentation. I am using the pygame pummel the chimp.
#Import Modules
import os, pygame
from pygame.locals import *
from pygame.compat import geterror
if not pygame.font: print ('Warning, fonts disabled')
if not pygame.mixer: print ('Warning, sound disabled')
main_dir = os.path.split(os.path.abspath(__file__))[0]
data_dir = os.path.join(main_dir, 'data')
#functions to create our resources
def load_image(name, colorkey=None):
fullname = os.path.join(data_dir, name)
try:
image = pygame.image.load(fullname)
except pygame.error:
print ('Cannot load image:', fullname)
raise SystemExit(str(geterror()))
image = image.convert()
if colorkey is not None:
if colorkey is -1:
colorkey = image.get_at((0,0))
image.set_colorkey(colorkey, RLEACCEL)
return image, image.get_rect()
def load_sound(name):
class NoneSound:
def play(self): pass
if not pygame.mixer or not pygame.mixer.get_init():
return NoneSound()
fullname = os.path.join(data_dir, name)
try:
sound = pygame.mixer.Sound(fullname)
except pygame.error:
print ('Cannot load sound: %s' % fullname)
raise SystemExit(str(geterror()))
return sound
#classes for our game objects
class Fist(pygame.sprite.Sprite):
"""moves a clenched fist on the screen, following the mouse"""
def __init__(self):
pygame.sprite.Sprite.__init__(self) #call Sprite initializer
self.image, self.rect = load_image('fist.bmp', -1)
self.punching = 0
def update(self):
"move the fist based on the mouse position"
pos = pygame.mouse.get_pos()
self.rect.midtop = pos
if self.punching:
self.rect.move_ip(5, 10)
def punch(self, target):
"returns true if the fist collides with the target"
if not self.punching:
self.punching = 1
hitbox = self.rect.inflate(-5, -5)
return hitbox.colliderect(target.rect)
def unpunch(self):
"called to pull the fist back"
self.punching = 0
class Chimp(pygame.sprite.Sprite):
"""moves a monkey critter across the screen. it can spin the
monkey when it is punched."""
def __init__(self):
pygame.sprite.Sprite.__init__(self) #call Sprite intializer
self.image, self.rect = load_image('chimp.bmp', -1)
screen = pygame.display.get_surface()
self.area = screen.get_rect()
self.rect.topleft = 10, 10
self.move = 9
self.dizzy = 0
def update(self):
"walk or spin, depending on the monkeys state"
if self.dizzy:
self._spin()
else:
self._walk()
def _walk(self):
"move the monkey across the screen, and turn at the ends"
newpos = self.rect.move((self.move, 0))
if self.rect.left < self.area.left or \
self.rect.right > self.area.right:
self.move = -self.move
newpos = self.rect.move((self.move, 0))
self.image = pygame.transform.flip(self.image, 1, 0)
self.rect = newpos
def _spin(self):
"spin the monkey image"
center = self.rect.center
self.dizzy = self.dizzy + 12
if self.dizzy >= 360:
self.dizzy = 0
self.image = self.original
else:
rotate = pygame.transform.rotate
self.image = rotate(self.original, self.dizzy)
self.rect = self.image.get_rect(center=center)
def punched(self):
"this will cause the monkey to start spinning"
if not self.dizzy:
self.dizzy = 1
self.original = self.image
def main():
"""this function is called when the program starts.
it initializes everything it needs, then runs in
a loop until the function returns."""
#Initialize Everything
pygame.init()
screen = pygame.display.set_mode((468, 100))
pygame.display.set_caption('Monkey Fever')
pygame.mouse.set_visible(0)
#Create The Backgound
background = pygame.Surface(screen.get_size())
background = background.convert()
background.fill((250, 250, 250))
#Put Text On The Background, Centered
if pygame.font:
font = pygame.font.Font(None, 36)
text = font.render("Pummel The Chimp, And Win $$$", 1, (10, 10, 10))
textpos = text.get_rect(centerx=background.get_width()/2)
background.blit(text, textpos)
#Display The Background
screen.blit(background, (0, 0))
pygame.display.flip()
#Prepare Game Objects
clock = pygame.time.Clock()
whiff_sound = load_sound('whiff.wav')
punch_sound = load_sound('punch.wav')
chimp = Chimp()
fist = Fist()
allsprites = pygame.sprite.RenderPlain((fist, chimp))
#Main Loop
going = True
while going:
clock.tick(60)
#Handle Input Events
for event in pygame.event.get():
if event.type == QUIT:
going = False
elif event.type == KEYDOWN and event.key == K_ESCAPE:
going = False
elif event.type == MOUSEBUTTONDOWN:
if fist.punch(chimp):
punch_sound.play() #punch
chimp.punched()
else:
whiff_sound.play() #miss
elif event.type == MOUSEBUTTONUP:
fist.unpunch()
allsprites.update()
#Draw Everything
screen.blit(background, (0, 0))
allsprites.draw(screen)
pygame.display.flip()
pygame.quit()
#Game Over
#this calls the 'main' function when this script is executed
if __name__ == '__main__':
main()
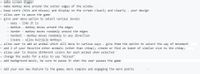

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Hello I need help with Some JavaScript.arrow_forwardPlease rewrite all of this animation code so that it performs the same functions in a java file instead of the files .css,.js, and .html (which is what I have) in visual studios. You would need to import javaFX since this would require GUI. My styles.css and Animation.html are attached as screenshots, and my script.js is below: script.js const dialogue = [ { character: 'Narrator', text: "In the dead of night, beneath a moon veiled by misty clouds, a lone figure trudged along a desolate forest path." }, { character: 'Narrator', text: "Unknown to her, she was not alone." }, { character: 'Human', text: "Who's there? Show yourself!" }, { character: 'Creature', text: "You venture into realms unknown, mortal. What brings you to these woods?" }, { character: 'Human', text: "I seek passage to the village beyond. I mean no harm." }, { character: 'Creature', text: "Harm is but a shadow in these woods, mortal." }, { character: 'Human', text: "What do you mean? Who are…arrow_forwardI am trying to loop through several FITS files that are located on my Desktop. This is the code I have so far. The final image that i need to produce is a "single plot". with multiple graphs overlayed (see image) import numpy as npimport matplotlib.pyplot as pltimport globfrom astropy.io import fitsfrom astropy.wcs import WCSfrom pathlib import Path%matplotlib inline%matplotlib widget plt.figure(figsize=(5,5))legends = [] def plot_fits_file(file_path): # used this data to test ---------- # lam = np.random.random(100) # flux = np.random.random(100) # -------------------- # below code will work when you have file # all the plot will be on single chart hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] file_path = Path(file_path)if file_path.exists(): plot_fits_file(file_path) plt.show()else:…arrow_forward
- simply fill in the blanks. To make it easier for you to enter the number, you may simply enter the number without having to indicate the base using the subscript. For example, you may enter AF instead of AFsixteen: Fill in the blanks. Provide the number immediately before and after the given number in the given base, respectively. No work to be shown for this problem. You may Click on Show Rich-Text Editor option for more formatting tools, such as subscript. 8D9FFsixteen. 1. F8DAOsixteen, 2. 3. 57100eight 4. , 100110000two, 4200seven, 5.arrow_forwardWhat special library is needed to create a character array? Group of answer choices iomanip string fstream no special libraryarrow_forwardCould you add lists or dictionaries to my code to manage the data the user entered!? Also, if you can make the code look better, please change it. Thanks!arrow_forward
- For this code please add these features making it be able to : #generate a blank image with black background, picture size is the same as frame #allow it to calculate center point #Draw white circles into the image generated at line 6, diameter is 6arrow_forwardburses.projectstem.org/courses/64525/assignments/9460863?module_item_id=18079077 Maps HH 50 Follow these steps to create your Warhol Grid: 1. Find or create an image (for this activity, a smaller starting image will produce higher quality results in a shorter amount of time). 2. Using the Python documentation as a guide, create a program that loads the image, filters three copies of it, and saves the result. Note: You will need to create the three filters using the filter() method. • Include multiple filters on at least two of your variants. One of your variants must apply a single filter multiple times with the use of a loop. For example, the top right image above uses a loop to blur the image 2 times (what if we did it 100 times?). U ▪ One of your images must apply at least two different filters to the same image. In the example image, the bottom right image includes a filter to smooth the image and then edge enhance them. L • Create the Warhol Grid using Python with the three images…arrow_forwardHow do we add text, shapes, and other annotations to Matplotib piots? O By passing an indcator variable indicating the quadrant of the gyraph for annotation to the correspor function or method By passing the (xy) coordinates to the corresponding function or method O By passing the asis labets for each object to the corresponding function or method O By passing a lst of strings to the corresponding function or methodarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
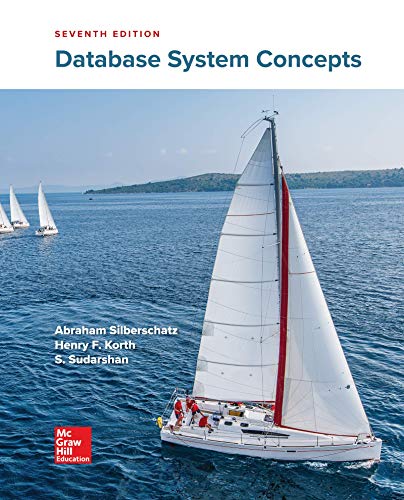
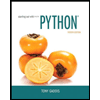
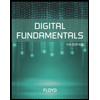
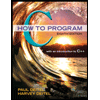
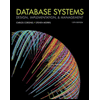
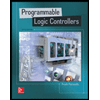