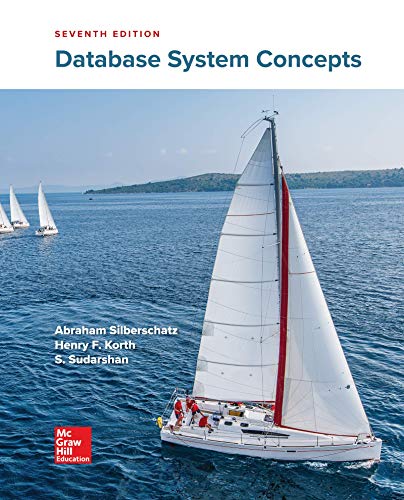
Help writing the SuccessInLife Class In Java, I will figure out driver class later
Class Fields/Variables: your class should have the following fields:
A static class variable/field that keeps track of how many event objects have been
created (0 by default).
The following instance variables/fields:
A 7 digit alpha numeric unique bar code for each event
The location of the event, which starts with the name of the building followed
by the room number
Event name
Event type, which can be one of the following
Quantity, which represents how many seats are requested
The event capacity (max number of spots allowed for this event)
The number of seats already taken
You should select the appropriate data type for each variable above. Make all instance
variables private and make the static class variable public.
Constructors: in addition to the default constructor, the class should have the following
overloaded constructors:
a constructor that receives the event name and event type as parameters, and initializes
the corresponding instance variables with those parameters. By default, it should set
the number of seats already taken to zero. Then, it should call the following methods in
order:
o calcMaximumSeats method by passing the event type as a parameter.
o generateBarCode method
Another constructor that receives the event name, event type, and event capacity as
parameters. This constructor does exactly what the previous constructor does, except
that it overrides the default capacity calculated by the calcMaximumSeats method.
For example, if the calcMaximumSeats method decides that the seminar in Fitch Hall holds up to 100 spots, the event planner might need to reduce that
capacity to 50 (due to budget constraints for example). You should use constructor
chaining instead of repeating code.
Methods:
The class should have the following private methods
A private method calcMaximumSeats that takes the event type as a parameter and
initializes the location and capacity variables based on the event type as follows:
For the job fair event type, you may set the capacity to a very large number (e.g.,
100000000)
A private generateBarCode method that does not take any arguments and generates a
bar code according to the following
Generates a 5-digit random number
Concatenates the first character of the event name, the first letter of the event
location, and the 5 digit number. For example, if name is Success in Life and
location is Library Hall 28, the generated code would be SLH89443.
The method does not return any value. It just assigns the generated code to the bar
code instance variable.
The ScienceFair class should have the following public methods.
Event type Location (Room) Capacity (Maximum
Seats)
Workshop Library Hall 28 30
Seminar Fitch Hall 151 100
Career fair Exhibit Hall Lobby Unlimited
• Getters and setters for all instance variables, event name, location, and
number of remining seats available. The method should take care of formatting the output
as shown in the sample output below. The method should be smart enough to display seat
, seats, or FULL depending on the return value of the
getAvailableSeats method
SLH23354 Success in Life Library Hall 28 FULL
LFH56742 Life Seminar Fitch Hall 151 20 seats
SLH23355 Succeful Communication Library 28 1 seat
JEH49492 Job Fair. Exhibit Hall Lobby Unlimited
A getAvailableSeats method that returns the difference between the capacity
(maximum number of spots) and the number of seats already taken.
Two overloaded register methods:
that handles group registration by taking the quantity as a parameter.
If the event type is career fair, the method should not check the remaining
seats. The method should increase the number of seats already taken by
quantity and return true. If not, the method should return false and leave
the number of seats already taken unchanged.
For other event types, it should check the number remaining seats. If
remaining seats is > 0, the method should increase the number of seats
already taken by quantity and return true. If not, the method should return
false and leave the number of seats already taken unchanged.
One that handles individual registration. This method should not take any
parameters, and should assume that the requested quantity is 1 by default.
If the event type is career fair, the method should not check the remaining
seats. The method should increase the number of seats already taken by 1
and return true. If not, the method should return false and leave the number
of seats already taken unchanged
For other event types, it should check the number remaining seats.
If remaining seats is > 0, the method should increase the number of
seats already taken by 1 and return true. If not, the method should
return false and leave the number of seats already taken unchanged.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- The non-static class level variables and non-static methods of a class are known as a. instance members b. object behaviors c. object attributes O d. object referencesarrow_forwardjavacoding please thank you!arrow_forward1. Create a class as described below: Class Name: Room Fields: standard room fee amenities total cost Constructors:A default constructor to initialize the fields. The standard room fee field should be set to $200 and the amenities field to zero. Another constructor with one argument. It should assign this value to the standard room fee and the amenities field to the default value of $75. Methods: getStandardFee() - This methods returns the standard room fee field value. getAmenities() - This method returns the amenities field value. getTotalCost() - This method returns the total cost field value. calcTotalCost() - This method calculated the total cost for an instance of the class setStandard Fee() - This method has one argument representing the standard room fee. setAmenities() - This method has one argument representing the amenities for the room.arrow_forward
- For your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardin c# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book.The Corner’s Turn() method should display You turn corners to go around the block.The Pancake's Turn() method should display You turn a pancake when it's done on one side.The Leaf's Turn() method should display A leaf turns colors in the fall.i keep getting the errors Unit TestIncompletePage class defined Build StatusBuild FailedBuild OutputCompilation failed: 3 error(s), 0 warnings NtTest4ac148a9.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?NtTest4ac148a9.cs(23,7): error CS0246:…arrow_forwardin C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forward
- Create a class of Dog, some of the differences you might have listed out maybe breed, age, size, color, etc. Then create characteristics (breed, age, size, color) can form a data member for your object. Then list out the common behaviors of these dogs like sleep, sit, eat, etc. Design the class dogs with objects and methods.arrow_forwardin C# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall.arrow_forwardWhen you want to listen for an event, you can implement an appropriate for your class Handler Listener Abstract class Superclassarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
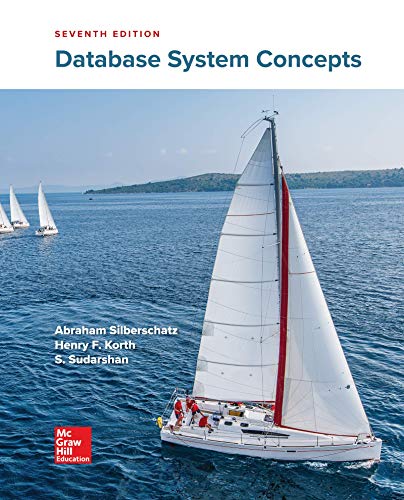
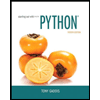
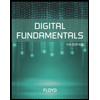
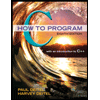
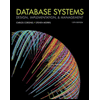
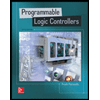