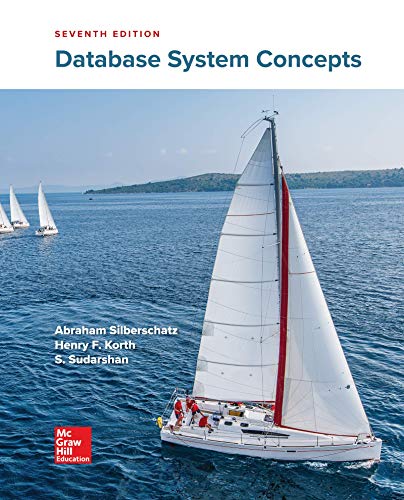
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Hello! I need some help with my Java homework. Please use Eclipse with JavaFX
Please add comments on the program so I can understand what the code is doing and learn
Don't use Liang's BallPane class. Just draw a Circle.
15.3 (Move the ball)
Write a program that moves the ball in a pane. You should define a pane class for displaying the ball and provide the methods for moving the ball left, right, up, and down, as shown in Figure 15.26c. Check the boundary to prevent the ball from moving out of sight completely.
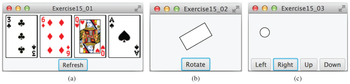
Transcribed Image Text:### Interactive GUI Exercises
#### Figure (a): Exercise15_01
This figure displays a graphical user interface (GUI) window titled "Exercise15_01." In the window, there are four playing cards shown:
- 3 of Clubs
- 6 of Diamonds
- Queen of Hearts
- Ace of Spades
Below the cards, there is a button labeled "Refresh," which likely allows the user to shuffle or change the displayed cards.
#### Figure (b): Exercise15_02
This figure showcases a GUI window titled "Exercise15_02" featuring a rectangle. The rectangle is displayed at an angle, suggesting it can be rotated. Below it, there is a button labeled "Rotate," likely used to change the orientation of the rectangle.
#### Figure (c): Exercise15_03
This figure presents a GUI window called "Exercise15_03" that includes a small circle. There are four directional buttons labeled "Left," "Right," "Up," and "Down," which are probably used to move the circle around within the window.
These exercises demonstrate basic GUI interactions involving elements like playing cards, shapes, and movement controls.
Expert Solution

arrow_forward
Step 1
//MoveTheBall.java
import javafx.application.Application;
import static javafx.application.Application.launch;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.input.KeyCode;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.Pane;
import javafx.scene.layout.VBox;
import javafx.scene.paint.Color;
import javafx.scene.shape.Circle;
import javafx.stage.Stage;
public class MoveTheBall extends Application {
//for holdong the ball create a Pane class
private class Ball extends Pane {
//distance which the ball moves at once
private final int MOVE_DISTANCE = 10;
//radius of the ball
private final int RADIUS = 15;
//Circle object representing the ball
private Circle circle;
//constructor method for initializing the Ball at specific x,y coordinates
public Ball(double x, double y) {
//initializing Circle, setting white fill and black stroke
circle = new Circle(x, y, RADIUS);
circle.setFill(Color.WHITE);
circle.setStroke(Color.BLACK);
//adding to this pane
getChildren().add(circle);
}
//move the ball to the left
public void moveLeft() {
//move only if the ball does not exceed the boundary
if ((circle.getCenterX() - MOVE_DISTANCE) >= RADIUS) {
circle.setCenterX(circle.getCenterX() - MOVE_DISTANCE);
}
}
//move the ball to the right
public void moveRight() {
//move only if the ball does not exceed the boundary
if ((circle.getCenterX() + MOVE_DISTANCE) <= (getWidth() - RADIUS)) {
circle.setCenterX(circle.getCenterX() + MOVE_DISTANCE);
}
}
//move the ball up
public void moveUp() {
//move only if the ball does not exceed the boundary
if ((circle.getCenterY() - MOVE_DISTANCE) >= RADIUS) {
circle.setCenterY(circle.getCenterY() - MOVE_DISTANCE);
}
}
//move the ball down
public void moveDown() {
//move only if the ball does not exceed the boundary
if ((circle.getCenterY() + MOVE_DISTANCE) <= (getHeight()- RADIUS)) {
circle.setCenterY(circle.getCenterY() + MOVE_DISTANCE);
}
}
}
@Override
public void start(Stage primaryStage) {
Ball ball =new Ball(100,100); //create a Ball at (100,100)
Button left = new Button("Left"); //create four buttons
Button right = new Button("Right");
Button up = new Button("Up");
Button down = new Button("Down");
//create an HBox to hold left and right buttons with 10px spacing
HBox hbox = new HBox(10, left, right, up, down);
hbox.setAlignment(Pos.CENTER); //center aligning the components
hbox.setPadding(new Insets(10)); //adding some padding space around the hbox
//adding action listeners to call appropriate functions in Ball class
left.setOnAction(e -> ball.moveLeft());
right.setOnAction(e -> ball.moveRight());
up.setOnAction(e -> ball.moveUp());
down.setOnAction(e -> ball.moveDown());
BorderPane root = new BorderPane(); //creating a BorderPane
root.setCenter(ball); //adding ball to center and hbox to bottom
root.setBottom(hbox);
Scene scene = new Scene(root); //set up the scene
primaryStage.setScene(scene); //setting and displaying the scene
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Q.1: With C#, using the Visual Studio, create a class named Shape with properties named Height, Width, and a method Area. Add two classes that derive from it- Rectangle and Square. Add any additional melmbers you feel are appropriate and implement the Area method correctly. In the RectangleTest class, instantiate objects of Rectangle and Square and call the properties and Area methods of both derived clases.arrow_forwardI need help with the content on the image below. The Code is Java.arrow_forwardtask 13.1 Follow these steps:● Modify the existing Animal.java file for this task.● Using the Lion class template, as shown in this pdf file, expand the class tohave features specific to a lion:○ Add a field for lion type (cub, male, female).○ Add a method in this class which sets the lion type based on itsweight (note that the weight is a derived field from the superclass).○ If the weight is less than 80kg, it’s type should be a cub. If less than120kg, it should be female. If greater than 120kg, it is a male.○ Include a method that will print out a description of a lion object.● Compile, save and run your file.Compulsory Task 2Follow these steps:● Modify the existing Animal.java file for this task.● Create a class called ‘Cheetah’ that:○ Inherits from the Animal class.○ Makes use of at least one static field which needs to have a staticsetter and getter.○ Contains a constructor.○ Contains a toString() method.○ Has an array as one of its fields.● Create an application class. Within the…arrow_forward
- Hi, I need help with Java code. Please see attached screenshot. Thank you! Input isarrow_forwardCan you do it in Javaarrow_forwardPlease write in java. Add comments but make the comments short. No need for really long comments. Please keep code very neat and simple , dont add anything unneccesary in the code if you weren't instructed to do so. If youve answered this before please dont copy and paste from a previous question! (Rewrite it in another way)..... Also be sure to read the instructions below carefully. The Instructions attached below will explain everything you'll be doing. NO THIS is not a homework assignment, it's practice work. Please type the code out so that I can copy and paste it into my IDE and see the output for my self but also still provide a screenshot of your output!arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
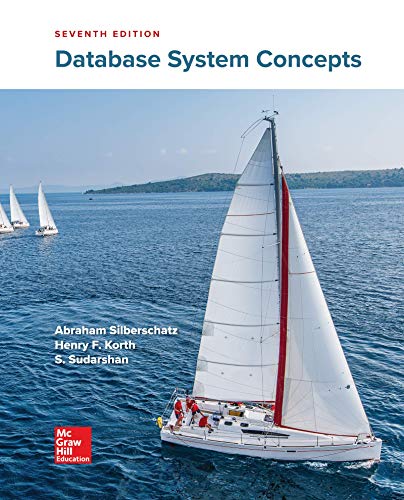
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
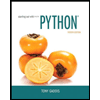
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
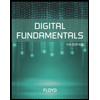
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
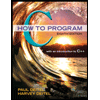
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
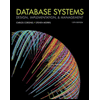
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
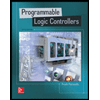
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education