Hello, I need help with this: – Assignment Following on the success of homework 2, your mother wants you to augment the tag sale price tracking program. In addition to storing the price of each item sold, she wants to store the name of the item sold. Create a class called TagSaleItem, with the following variables (properties) and Get/Set members (functions) Double itemPrice String itemName Add a textbox to your form to input the name of the item at the same time as the price. The previous program used an array to store a list of prices. It would be awesome if this program used an array of objects to store a list of items… but we’ll get to that in a later assignment. For now the assignment is just to create a class and a single object and demonstrate how to use it (using the Get/Set methods) Code for homework 2 that is referenced: Imports System.IO Public Class FrmMain Dim prices(0) As Double ' Array to store prices of items sold Dim totalSales As Double ' Total amount of money collected Dim totalItems As Integer ' Total number of items sold Dim averageSalePrice As Double ' Average sale price Private Sub FrmMain_Load(sender As Object, a As EventArgs) Handles MyBase.Load Dim fileName As String = "sales_data.txt" If File.Exists(fileName) Then ' Open the file for reading Dim sr As New StreamReader(fileName) Dim i As Integer = 0 ' Read each line and store it in the array Do While sr.Peek() >= 0 ReDim Preserve prices(i) prices(i) = sr.ReadLine() i += 1 totalSales += prices(i - 1) totalItems += 1 Loop ' Close the file after reading sr.Close() End If ' Update the statistics in the GUI averageSalePrice = totalSales / totalItems lblTotalSales.Text = "Total Sales: " + totalSales.ToString() lblTotalItems.Text = "Total Items: " + totalItems.ToString() lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString() End Sub Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click Dim fileName As String = "sales_data.txt" ' Open the file for writing Dim sw As New StreamWriter(fileName, False) ' Write each price in the array to a new line in the file For i As Integer = 0 To prices.Length - 1 sw.WriteLine(prices(i)) Next ' Close the file after writing sw.Close() Me.Close() End Sub Private Sub btnSubmitPrice_Click(sender As Object, e As EventArgs) Handles btnSubmitPrice.Click Dim price As Double = InputBox("Enter Price: ", "Enter a Number") ' Add the price to the array and update the statistics ReDim Preserve prices(prices.Length + 1) prices(prices.Length - 1) = price totalSales += price totalItems += 1 averageSalePrice = totalSales / totalItems ' Display the statistics lblTotalSales.Text = "Total Sales: " + totalSales.ToString() lblTotalItems.Text = "Total Items: " + totalItems.ToString() lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString() End Sub End Class
Hello, I need help with this:
– Assignment
Following on the success of homework 2, your mother wants you to augment the tag sale price tracking
program. In addition to storing the price of each item sold, she wants to store the name of the item sold.
Create a class called TagSaleItem, with the following variables (properties) and Get/Set members
(functions)
Double itemPrice
String itemName
Add a textbox to your form to input the name of the item at the same time as the price.
The previous program used an array to store a list of prices. It would be awesome if this program used
an array of objects to store a list of items… but we’ll get to that in a later assignment. For now the
assignment is just to create a class and a single object and demonstrate how to use it (using the Get/Set
methods)
Code for homework 2 that is referenced:
Imports System.IO
Public Class FrmMain
Dim prices(0) As Double ' Array to store prices of items sold
Dim totalSales As Double ' Total amount of money collected
Dim totalItems As Integer ' Total number of items sold
Dim averageSalePrice As Double ' Average sale price
Private Sub FrmMain_Load(sender As Object, a As EventArgs) Handles MyBase.Load
Dim fileName As String = "sales_data.txt"
If File.Exists(fileName) Then
' Open the file for reading
Dim sr As New StreamReader(fileName)
Dim i As Integer = 0
' Read each line and store it in the array
Do While sr.Peek() >= 0
ReDim Preserve prices(i)
prices(i) = sr.ReadLine()
i += 1
totalSales += prices(i - 1)
totalItems += 1
Loop
' Close the file after reading
sr.Close()
End If
' Update the statistics in the GUI
averageSalePrice = totalSales / totalItems
lblTotalSales.Text = "Total Sales: " + totalSales.ToString()
lblTotalItems.Text = "Total Items: " + totalItems.ToString()
lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString()
End Sub
Private Sub btnExit_Click(sender As Object, e As EventArgs) Handles btnExit.Click
Dim fileName As String = "sales_data.txt"
' Open the file for writing
Dim sw As New StreamWriter(fileName, False)
' Write each price in the array to a new line in the file
For i As Integer = 0 To prices.Length - 1
sw.WriteLine(prices(i))
Next
' Close the file after writing
sw.Close()
Me.Close()
End Sub
Private Sub btnSubmitPrice_Click(sender As Object, e As EventArgs) Handles btnSubmitPrice.Click
Dim price As Double = InputBox("Enter Price: ", "Enter a Number")
' Add the price to the array and update the statistics
ReDim Preserve prices(prices.Length + 1)
prices(prices.Length - 1) = price
totalSales += price
totalItems += 1
averageSalePrice = totalSales / totalItems
' Display the statistics
lblTotalSales.Text = "Total Sales: " + totalSales.ToString()
lblTotalItems.Text = "Total Items: " + totalItems.ToString()
lblAverageSalePrice.Text = "Average Sale Price: " + averageSalePrice.ToString()
End Sub
End Class


Trending now
This is a popular solution!
Step by step
Solved in 4 steps

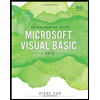
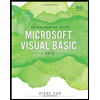
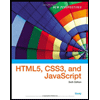