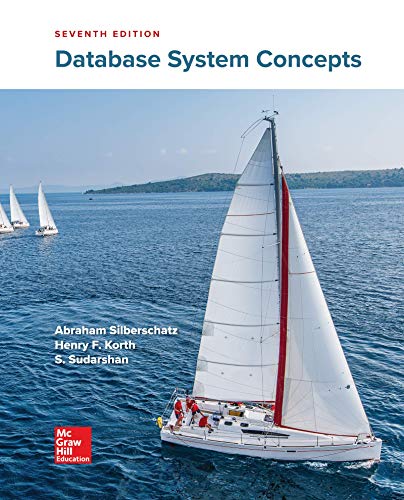
Hello, I am having some trouble with this homework question for my C++ course.
Define a Pet class that stores the pet’s name, age, and weight. Add appropriate constructors, accessor functions, and mutator functions. Use dynamic variable for name. Also define a function named getLifespan that returns a string with the value “unknown lifespan.” Then, write big three for Pet class. (Please indicate call of copy constructor, assignment operator, and destructor)
Use following main() to test your class.(DO NOT change the int main as any alleration will not be accepted)
int main(){
Pet p("Kitty",2,10);
cout<<p.getName()<<endl;
cout<<p.getAge()<<endl;
cout<<p.getWeight()<<endl;
cout<<p.getLifespan()<<endl;
Pet p1 = p, p2;
p1.setName("Doggy");
cout<<p1.getName()<<endl;
cout<<p1.getAge()<<endl;
cout<<p1.getWeight()<<endl;
cout<<p1.getLifespan()<<endl;
p2 = p1;
p2.setName("Teddy");
cout<<p2.getName()<<endl;
cout<<p2.getAge()<<endl;
cout<<p2.getWeight()<<endl;
cout<<p2.getLifespan()<<endl;
}
Output from main function above:
Kitty
2
10
Unknown lifespan
==> (Pet) copy constructor was called
Doggy
2
10
Unknown lifespan
==> (Pet) Assignment operator was called
Teddy
2
10
Unknown lifespan
==> (Pet) Destructor was called
==> (Pet) Destructor was called
==> (Pet) Destructor was called

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Hello, I'm working on this hangman simulation in C++. The code runs but it doesn't count wrong guesses after one letter is guessed correctly. Also, the body of the hangman comes out wrong. Could you help? #include<iostream>#include<cstring> // for string class functions#include<fstream>#include <cctype>using namespace std; int main(){ // define variable to get the response from user "Yes" or "No" string response; // Define index variable int w = 0; // define number of words that need to be guessed by the user assume 4 const int WORDS = 4; // loop do { // we will define the hangman body const char body[] = "o/|\\|\\"; // here we define the words string words[WORDS] = {"MACAW", "SADDLE", "TOASTER", "XENOICIDE"}; // fetch size or length string xword(words[w].length(),'*'); // define iterator to fetch the words string::iterator ix = xword.begin(); string::iterator i…arrow_forwardSubmit code in C++ CODE with comments and output screenshot is must to get Upvote. Thanksarrow_forwardThe static data members and static member functions aren't quite what I'm going for in this particular instance.arrow_forward
- C++ Code Step 1: Preparation For the moment, "comment out" the following under-construction code: In dynamicarray.h: All function prototypes except the constructors and destructor. Keep the member variables (but we will be replacing them shortly). In dynamicarray.cpp: All function implementations except the constructors and destructor. You should also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away. In main: Comment out all the code inside the RunPart1Tests function between the linesbool pass = true; and return pass; • Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return 0;" line. Step 2: Replacing member data and the two constructors You're going to replace the current member data (arr, len, and capacity) with a single vector of integers. Remember that vectors keep track of their own size and capacity, so…arrow_forwardStep 1: Preparation Comment out the following under-construction code: In dynamicaray.h: Al function prototypes except the constructors and destructor. Keep the member variables. In dynamicarray.cpp: All function implementations except the constructors and destructor. Also remove (not just comment out) INITIAL_CAP, and replace it with a hard-coded 10 inside the default constructor. This will also eventually go away. In main: Comment out all the code inside the RunPart1Tests function between the lines. Also in main: Comment out all the code in the main function starting with the "Equality comparison" comment and just before the "return O;" line. bool pass = true; and return pass; Step 2: Replacing member data and the two constructors Replace the current member data (arr, len, and capacity) with a single vector of integers. At the top of your h, include the vector library. This is the only place you should need to include it. Also in your .h, remove your current member data, replace…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
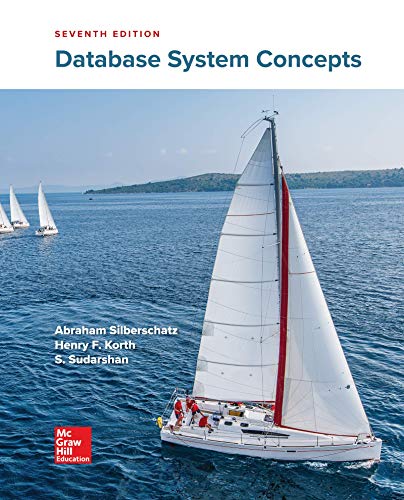
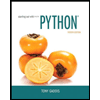
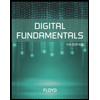
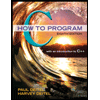
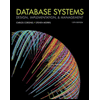
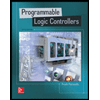