given two integers n and k. You are approached to pick greatest number of particular integers from 1 to n so that there is no subset of picked numbers with aggregate equivalent to k. A subset of a set is a set that can be gotten from starting one by eliminating a few (potentially all or none) components of it.
Correct answer will be upvoted else downvoted. Computer science.
You are given two integers n and k. You are approached to pick greatest number of particular integers from 1 to n so that there is no subset of picked numbers with aggregate equivalent to k.
A subset of a set is a set that can be gotten from starting one by eliminating a few (potentially all or none) components of it.
Input
The main line contains the number of experiments T (1≤T≤100).
Every one of the following T lines contains two integers n and k (1≤k≤n≤1000) — the depiction of experiments.
Output
For each experiment output two lines. In the main line output a solitary integer m — the number of picked integers.
In the subsequent line output m particular integers from 1 to n — the picked numbers.
In case there are various replies, print any. You can print the numbers in any request

Step by step
Solved in 3 steps with 1 images

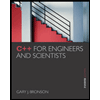
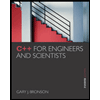