Given the following challenges, use append(), extend(), pop(), and insert()to change the contents of the lists. Suppose that we have the following list: Strawberry, Blueberry, Blackberry, Cranberry Define the list berries with the following elements shown in Snippet 5.11: Strawberry, Blueberry, Blackberry, Cranberry Using the built-in append() method, add the value Raspberry to the list berries. Using the built-in extend() method, extend an empty fruits list from the berries list that you created previously. Insert Mangoes into the fruits list at index 2 using the built-in method insert(). Remove the element at index 1 from the fruits list using the built-in method pop() and replace it with Apples, using the insert() built-in method. Finally, sort the elements in the fruits list by using the built-in method sort() with keyword arguments. Set the key argument to None and the reverse argument to False.
Need help with this:
Given the following challenges, use append(), extend(), pop(), and insert()to change the contents of the lists.
Suppose that we have the following list:
Strawberry, Blueberry, Blackberry, Cranberry
Define the list berries with the following elements shown in Snippet 5.11:
Strawberry, Blueberry, Blackberry, Cranberry
-
Using the built-in append() method, add the value Raspberry to the list berries.
-
Using the built-in extend() method, extend an empty fruits list from the berries list that you created previously.
-
Insert Mangoes into the fruits list at index 2 using the built-in method insert().
-
Remove the element at index 1 from the fruits list using the built-in method pop() and replace it with Apples, using the insert() built-in method.
-
Finally, sort the elements in the fruits list by using the built-in method sort() with keyword arguments. Set the key argument to None and the reverse argument to False.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

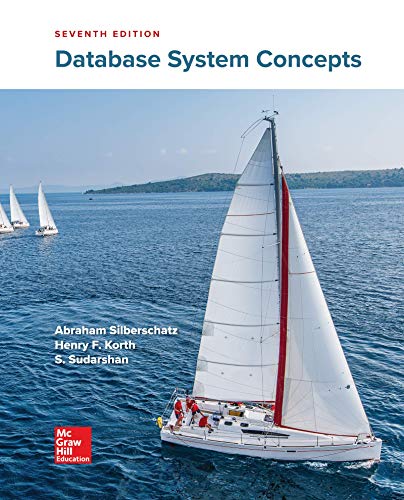
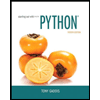
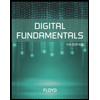
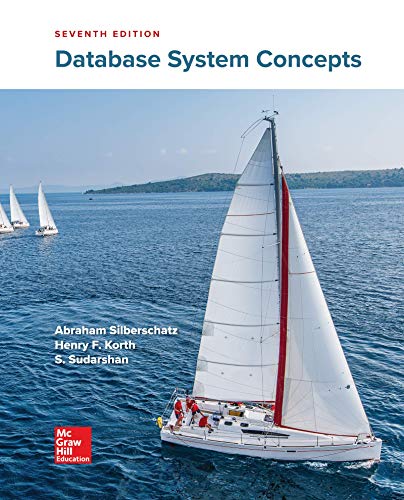
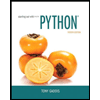
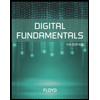
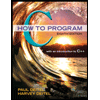
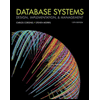
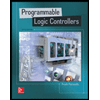