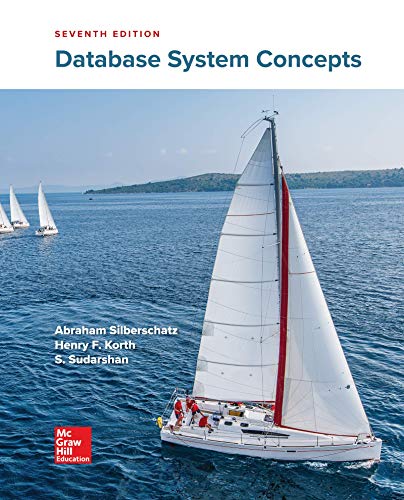
Given main(), define the Artist class (in file Artist.java) with constructors to initialize an artist's information, get methods, and a printInfo() method. The default constructor should initialize the artist's name to "unknown" and the years of birth and death to -1. printInfo() displays "Artist:", then a space, then the artist's name, then another space, then the birth and death dates in one of three formats:
- (XXXX to YYYY) if both the birth and death years are nonnegative
- (XXXX to present) if the birth year is nonnegative and the death year is negative
- (unknown) otherwise
Define the Artwork class (in file Artwork.java) with constructors to initialize an artwork's information, get methods, and a printInfo() method. The default constructor should initialize the title to "unknown", the year created to -1. printInfo() displays an artist's information by calling the printInfo() method in Artist.java, followed by the artwork's title and the year created. Declare a private field of type Artist in the Artwork class.
Ex: If the input is:
Pablo Picasso 1881 1973 Three Musicians 1921
the output is:
Artist: Pablo Picasso (1881 to 1973) Title: Three Musicians, 1921
Ex: If the input is:
Brice Marden 1938 -1 Distant Muses 2000
the output is:
Artist: Brice Marden (1938 to present) Title: Distant Muses, 2000
Ex: If the input is:
Banksy -1 -1 Balloon Girl 2002
the output is:
Artist: Banksy (unknown) Title: Balloon Girl, 2002

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- An object of the class Timer is used to indicate when a certain amount of time (in seconds) has elapsed, after which it provides a “DING". The required data field is remainingTime which is the number of seconds until the “DING". When a Timer object is constructed, it is set with the desired number of seconds. When the timer "ticks", it is one second closer to the "DING". This is represented with the tick) method. The dingChecko method indicates whether the timer should be "DINGING". Provide the code for the constructor method, and the methods getRemainingTime), tick(), and dingCheck). public class Timer // Methods // Data private int KeuainingTinei }arrow_forwardCreate a Class Pet with the following data members Identification: String species : String (e.g. cat, dog, fish etc) breed: String Age (in days): int Weight: float Dead: boolean 1. Provide a constructor with parameters for all instance variables. 2. Provide getters for all and setters for only breed, and weight 3. Provide a method growOld() that increases the age of the pet by one day. A dead pet wont grow old 4. Provide a method growHealthy(float w) that increases the weight of the pet by the given amount w. A dead pet cannot grow healthy. 5. Provide a method fallSick(float w) that reduces the weight of the pet by the given amount. The least weight a pet can have is 0 which will mean that the pet has died. If the value of weigh is 10 kg and the method is called with an argument of 11 kg then you will set it to 0 and set the dead to an appropriate value to mark the death of the pet 6. Provide a toString method that shows an appropriate well formatted string…arrow_forwardZOOM + Press Esc to exit full screen Create a new java project named CH46EX 1. Create a class named Student 1. 2 private instance data fields – student name, student id 2. 1 final and static constant for a COLLEGE, set to "Fullerton" Create one programmer written constructor that accepts parameters for two instance data fields during instantiation Create a static method named demoStaticMethod ()-see output 3. | - print message, print college 4. Create a non-static method named demoObjectMethod ()– see output - print message, college, student name, student id 2. Create an application named StudentDemo 1. Create/instantiate 1 student object 2. Call each method correctly This demo is a static method Fullerton 3. Expected Output > This demo is a non-static method Fullerton Name: Wyle Coyote ID: 456arrow_forward
- Assume the "Student" class has a constructor that assigns a string to an attribute called "name", has a setter method that assigns a string to the object's "name" attribute and a getter method that returns a Student object's name attribute. What will the following code print? Student student1 = new Student("Alex"); student1; Student student2 = Student student 3 = student2 Student2.setName("Aiden") ; Student3.setName("River"); System.out.println(student1.getName());arrow_forwardIn java language Class Design – Date V0.0 & V1.0 Create a new project for this lab and create a new class inside called Date. If you created a Date class last week, you may use it as a base. What does it mean to be a Date? What data items (called “state”) do you need to keep track of? Alternatively, what does a date have? (“has a” == composition) What can a Date do? What are the actions and verbs that can be applied to a date? Come up with one or two. These become the class’s methods. Try adding 3 data items to your Date to manage the month, day and year. Should these be local variables? Class-level (or instance) variables? Now let’s build a method to set the date This function should take 3 integers as input and assign these to your instance variables declared in the previous step. public void setDate(int m, int d, int y) { And, let’s build a method to report the date This function takes no input and uses the console to output the Date in the format…arrow_forwardCreate a new Teller Class Build a new Teller class(“Teller.java”). This class will be a sub-class of Employee. The Teller class should have all the same properties as an Employee as well as two new properties: hoursWorked and shift. HoursWorked is the total hours that this teller worked this week, and shift is either “day” or “evening” shift. Make sure to add 2 constructors and a display() method. Do not include a main() method in the Teller class. Test out this Teller class, by building a separate Tester class called TellerTester stored in a new file called ( “TellerTester.java”. This TellerTester class will only have a main() method, that will instantiate a Teller object, pass all the data to the Teller constructor, and lastly call the Teller display() method; just these 3 lines.arrow_forward
- Create a Point class to hold x and y values for a point. Create methods show(), add() and subtract() to display the Point x and y values, and add and subtract point coordinates. Create another class Shape, which will form the basis of a set of shapes. The Shape class will contain default functions to calculate area and circumference of the shape, and provide the coordinates (Points) of a rectangle that encloses the shape (a bounding box). These will be overloaded by the derived classes; therefore, the default methods for Shape will only print a simple message to standard output. Create a display() function for Shape, which will display the name of the class and all stored information about the class (including area, circumference and bounding box). Build the hierarchy by creating the Shape classes Circle, Rectangle and Triangle. Search the Internet for the rules governing these shapes, if necessary. For these three Shape classes, create default constructors, as well as constructors…arrow_forwardComplete the Car class by creating an attribute purchase_price (type int) and the method print_info() that outputs the car's information. Ex: If the input is: 2011 18000 2018 where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, then print_info() outputs: Car's information: Model year: 2011 Purchase price: $18000 Current value: $5770 Note: print_info() should use two spaces for indentation.arrow_forwardCreate a class called Elevator that can be moved between floors in an N-storey building. Elevator uses a constructor to initialize the number of floors (N) in the building when the object is instantiated. Elevator also has a default constructor that creates a five- (5) storey building. The Elevator class has a termination condition that requires the elevator to be moved to the main (i.e., first) floor when the object is cleaned up. Write a finalize() method that satisfies this termination condition and verifies the condition by printing a message to the output, Elevator ending: elevator returned to the first floor. In main(), test at least five (5) possible scenarios that can occur when Elevator is used in a building with many floors (e.g., create, move from one floor to another, etc.). Hint: Termination occurs when the instance is set to null. You may wish to investigate “garbage collection” for this exercise.arrow_forward
- a constructor that takes in an int, a string, and a double, constructor will assign them to the ID, name, and Averageinstance variables, then a no-argument (zero-parameter) constructor that will set the ID to the name to “No name assigned” and the Average to –1.0. With an accessor method for the name variable and a mutator method for the average variable. a toString() method that will display all three instance variables on a single line, with a " | " between each one. and then an equals() method that returns true only if all three instance variables are equalarrow_forwardFor this lab task, you will work with classes and objects. Create a class named text that works similar to the built-in string class. You will need to define a constructor that can be used to initialize objects of this new class with some literal text. Next, define three methods: to_upper() that will convert all characters to uppercase, reverse() that will reverse the text and length() that will return the length of the text. After you have completed this part, copy the following mainfunction to your code and check if the implementation is correct. int main() { text sample = "This is a sample text"; cout << sample.to_upper(); // This should display "THIS IS A SAMPLE TEXT" cout << endl;cout << sample.reverse(); // This should display "txet elpmas a si sihT"cout << endl; cout << sample.length(); // This should display 21 }arrow_forwardSammy's Seashore Supplies rents beach equipment such as kayaks, canoes, beach chairs, and umbrellas to tourists. In Chapter 3, you created a Rental class for the company. The Rental class contains two public final static fields that hold the number of minutes in an hour and the hourly rental rate ($40), and four private fields that hold a contract number, number of hours for the rental, number of minutes over an hour, and the price. It also contains two public set methods and four public get methods. Now, modify the Rental class to contain two overloaded constructors. One constructor accepts a contract number and number of minutes as parameters. Pass these values to the setContractNumber() and setHoursAndMinutes() methods, respectively. The setHoursAndMinutes() method will automatically calculate the hours, extra minutes, and price. The other constructor is a default constructor that passes "A000" and 0 to the two-parameter constructor. Save the file as Rental.java. b. In Chapter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
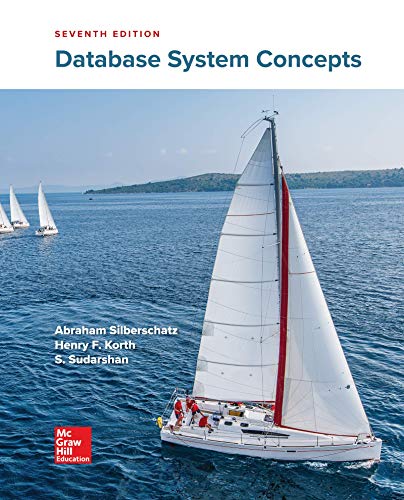
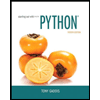
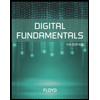
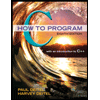
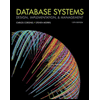
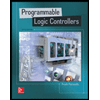