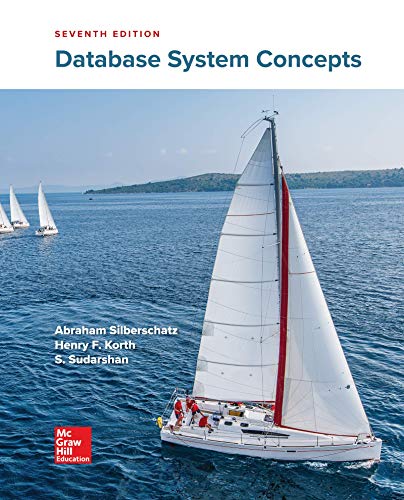
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters.
![Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the
student's GPA (double). Assume student's name has a maximum length of 20 characters.
Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as
listed below:
• Student InitStudent() - initializes name to "Louie" and gpa to 1.0
• Student SetName(char *name, Student s) - sets the student's name
• Student SetGPA(double gpa, Student s) - sets the student's GPA
• void GetName(char* studentName, Student s) - return the student's name in studentName
• double GetGPA(Student s) - returns the students GPA
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes:
Felix/3.7
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
4 #include "Student.h"
5
6 int main() {
8
9
10
11
12
13
14
15
16
47895
17
18
19 }
Current file: main.c _
Student student = InitStudent ();
char name [20];
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA (student));
student = SetName("Felix", student);
student SetGPA (3.7, student);
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA(student));
return 0;](https://content.bartleby.com/qna-images/question/5779ee7a-d811-43a6-ba75-7d41285b789d/72eeba0d-942f-4d35-8bf1-53386d927729/lma64t_thumbnail.png)
Transcribed Image Text:Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the
student's GPA (double). Assume student's name has a maximum length of 20 characters.
Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as
listed below:
• Student InitStudent() - initializes name to "Louie" and gpa to 1.0
• Student SetName(char *name, Student s) - sets the student's name
• Student SetGPA(double gpa, Student s) - sets the student's GPA
• void GetName(char* studentName, Student s) - return the student's name in studentName
• double GetGPA(Student s) - returns the students GPA
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes:
Felix/3.7
File is marked as read only
1 #include <stdio.h>
2 #include <string.h>
4 #include "Student.h"
5
6 int main() {
8
9
10
11
12
13
14
15
16
47895
17
18
19 }
Current file: main.c _
Student student = InitStudent ();
char name [20];
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA (student));
student = SetName("Felix", student);
student SetGPA (3.7, student);
GetName(name, student);
printf("%s/%.11f\n", name, GetGPA(student));
return 0;
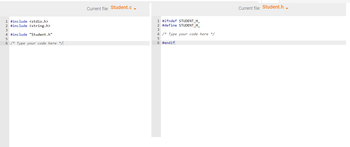
Transcribed Image Text:1 #include <stdio.h>
2 #include <string.h>
3
4 #include "Student.h"
5
6 /* Type your code here */
Current file: Student.c
1 #ifndef STUDENT H
2 #define STUDENT_H_
3
4 /* Type your code here */
5
6 #endif
Current file: Student.h
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Describe how to make an array of struct elements.arrow_forward2) Assign missing properties to the cats 3) Create two more Cats using two constructors that were not yet used 4) Create an array of size 4 of Cat data type, add all 4 Cats into your array. 5) Print information about all 4 Cats formatted in a table form using for-each looparrow_forwardR4arrow_forward
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
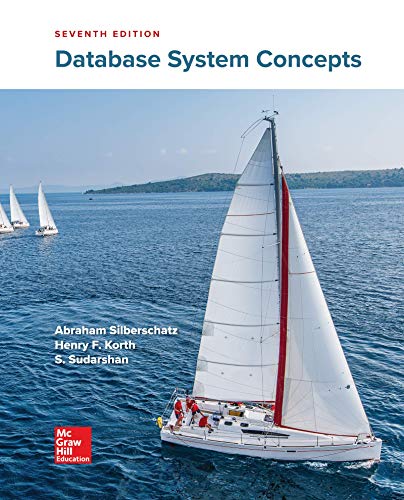
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
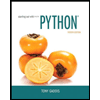
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
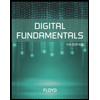
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
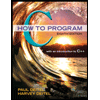
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
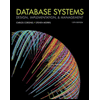
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
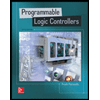
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education