Given a string , return the longest unique substring that occurs within . A unique substring is a substring within which DOES NOT have any repeating characters. As an example, "xd" is unique but "xxd" is not.
LAB RESTRICTIONS, PLEASE READ:
- Do not add any imports, the ones that you need will be given to you.
- Do not use recursion.
- Do not use try-except statements, you should be able to anticipate
or prevent any errors from happening at all!
- Code in python
- Should work for given doctest
def longest_unique_substring(s: str) -> str:
"""
Given a string <s>, return the longest unique substring that occurs within
<s>.
A unique substring is a substring within <s> which DOES NOT have any
repeating characters. As an example, "xd" is unique but "xxd" is not.
If there are two equal length unique substrings within <s>, return the one
that starts first (i.e., begins at a smaller index).
>>> longest_unique_substring('aab')
'ab'
"""

Step by step
Solved in 4 steps with 2 images

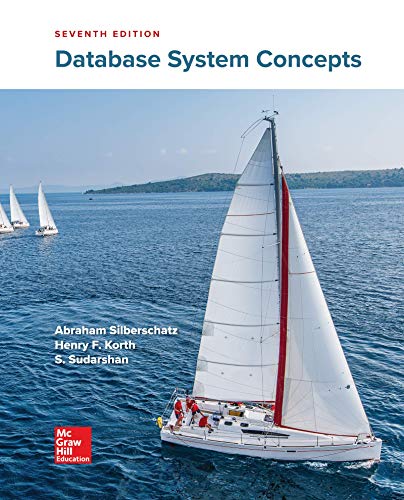
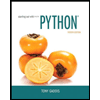
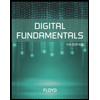
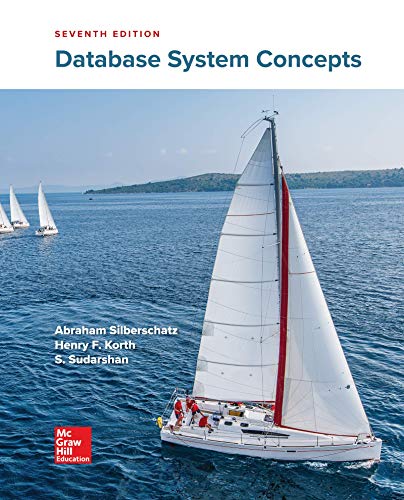
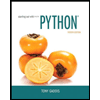
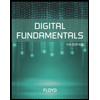
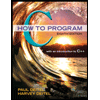
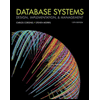
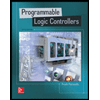