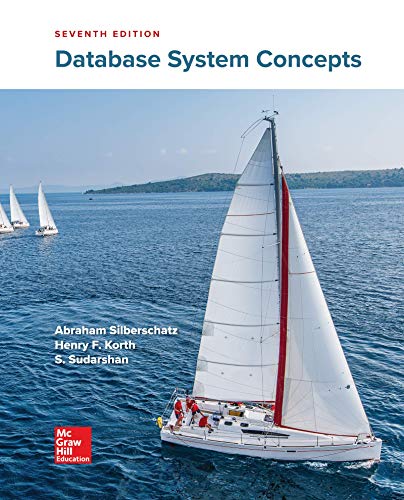
Given a problem and program, fill up the blank spaces in the program in order to make the program work correctly.
Health Center hospital has a registration form for the patients to register their details. Exceptions should be handled when the patient enters his/her details, because the users may enter invalid details into the form(For example age should have only positive values). Implement this concept by using Exception handling in c++.
The class named Register with the following constructor and methods.
Constructor | Description |
Register(string name,int age,string gender,int height,int weight) | Set the values passed as parameters. |
void display() | This method displays patient's name,age,gender,height,weight. |
Input and Output Format :
Refer sample input and output for formatting specifications.
All text in bold corresponds to input and the rest corresponds to output.
Sample Input and Output:
Enter your name
Nivya
Enter your age
-21
Exception: Age should be positive
Enter your age again
21
Enter your gender
Female
Enter your height(in centimeters)
156
Enter your weight(in kilograms)
-48
Exception: Weight should be positive
Enter your weight(in kilograms) again
48
Name : Nivya
Age : 21
Gender : Female
Height : 156 cm
Weight : 48 kg
----------- PLACE TO FILLUP IN BELOW PROGRAM AT LINE NUMBER 43 , 60 , 75 -----------------------------------------
1 #include<iostream>
|

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- This function determines how many computer instructions are necessary for each source-level statement.arrow_forwardThis variable keeps track of the total number of source-level statements as well as the total number of I/O operations.arrow_forwardThis function computes the number of instructions necessary for each source-level statement.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
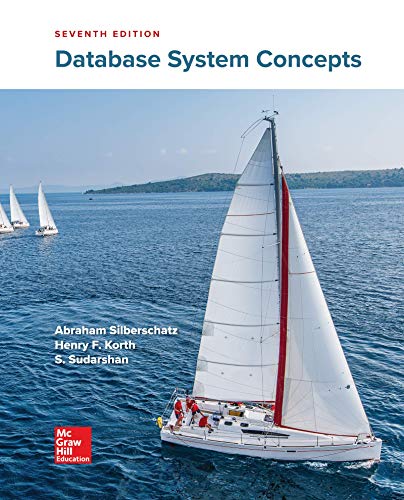
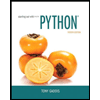
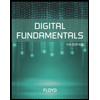
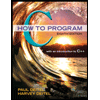
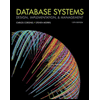
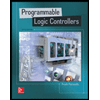