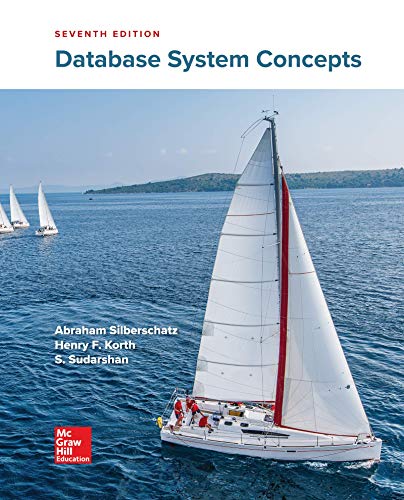
Concept explainers
Systems
Given a binary file containing the scores of 50 students in a class on Systems Programming course, compute the following statistics. Design the program such that the file should be specified as command line argument.
1. Maximum score
2. Min score
3. Average score.
4. Standard deviation
5. Number of students who got an “A Grade (90 <= score <= 100)
6. Number of students who got a “B” grade (80 <= score <90)
7. Number of students who got a “C” grade (70 <= score <80)
8. Number of students who got a “D” grade (60 <= score <70)
9. Number of students failed (score < 60)
Write a separate function in a separate file for each of the above and a header file and ‘scores.c’ for main function.
Menu:
1: for Max score, 2: for Min score, 3: ---- 9: for Number of students failed.
10. for Exit, 0: for continue.
The data file scores.dat is attached to the assignment. You may download the file and copy into Cygwin with the following command:
cp “C:\Users\loginname\Downloads\scores.dat” scores.dat
OR you can use the attached C-program to generate your own scores.dat file
1. Statement of the problem (as given above)
2. Program Design (function hierarchy diagram) and program code (weight 0.2)
3. Compilation results (copy from unix window and paste it to MSWORD file)
4. Show the results (copy from unix window and paste it to MSWORD file)
5. If the program has bugs, show you used debugger and show how you fixed the program
(Get a screen shot and paste it to MSWORD file)
6. Conclusions
prog scores.c
#include <stdio.h> #include <stdlib.h> #include <math.h> int main() { FILE *fp; int i; fp = fopen("scores.dat","wb"); int scr[50]; for (i=0; i < 50; i++) scr[i] = 50+random()%100; fwrite(scr,50, sizeof(int),fp); return 0; }
create your own scores.dat file
in C language please

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 16 images

- GDOT has contacted you to help write code in C++ to control the railroad signals (barriers with flashing lights and sounds across railroad tracks) in Georgia. You must create a Railroad Signal class with three hidden attributes (Up, Down and Signal), two constructors (a default that sets Up to on, Down and signal to off, and an overloaded that sets all Up to off, Down and Signal to on) and a method that changes the position and activates or deactivates the Signal.arrow_forwardprogram5_1.pyWrite a program that operates like a cashier terminal in a grocery store. It begins by prompting for the number of different items being purchased and then starts a loop. In the loop, the program should prompt for the item description, price and quantity of each item being purchased. These three values should be passed as arguments to a custom function that is defined in a separate module file. The imported function should print the subtotal for the item and return it to main. The total should be printed in main after the loop ends.arrow_forwardWrite a program in C++ that allows the user to create files of random data. They should be offered a menu of possibilities: 1) create random Whole number data file 2) create random Decimal number data file 3) create random Character data file 4) Quit programarrow_forward
- Files Class Activity Write a program to write your first name (hard coded) to the screen and possibly to a file whose name is passed into the program from the command line. It can be written in upper or lower case or mixed case as the default. Usage: writer [-f filename] If the -f option is included with a filename, then create a file with that name or overwrite a previously existing file and write your name in on the first line. If the -f option is not included, then only write your name to the screen followed by a newline. Use a copy of the original getopt to parse the command line. Be sure to test whether a file open succeeds or not and close any file you open when done.arrow_forwardUser Input Program and Analysis (Last answer to this question was incorrect.) Demonstrate an understanding of C++ programming concepts by completing the following: Program: Create a C++ program that will obtain input from a user and store it into the provided CSC450_CT5_mod5.txt Download CSC450_CT5_mod5.txtfile. Your program should append it to the provided text file, without deleting the existing data: Store the provided data in the CSC450_CT5_mod5.txt file. Create a reversal method that will reverse all of the characters in the CSC450_CT5_mod5.txt file and store the result in a CSC450-mod5-reverse.txt file. Program Analysis: Given your program implementation, discuss and identify the possible security vulnerabilities that may exist. If present, discuss solutions to minimize the vulnerabilities. Discuss and identify possible problems that can result in errors for string manipulation of data. Your analysis should be 1-2 pages in length. TXT FILE INFORMATION (Txt file should NOT…arrow_forwardjust do the first step. and the program should be in python and includ as many different functions as possible .arrow_forward
- Please use Replit to complete in C programming language. PLEASE FOLLOW guidelines CLOSELY AND SHOW OUTPUTSarrow_forwardFile redirection allows you to redirect standard input (keyboard) and instead read from a file specified at the command prompt. It requires the use of the < operator (e.g. java MyProgram < input_file.txt) True or Falsearrow_forwardjava Program Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage I do not want a goodbye system out in the program and it has to pass all the test cases. Test Case 1 Please enter the file name or type QUIT to exit:\ninput1.txtENTERTotal: 11.800\nAverage: 2.950\n Test Case 2 Please enter the file name or type QUIT to exit:\ninput2.txtENTERTotal: 17.300\nAverage: 3.460\n Test Case 3 Please enter the file name or type QUIT to exit:\ninput3.txtENTERTotal: 1.124\nAverage: 1.124\n Test Case 4 Please enter the file name or type QUIT to exit:\ninput4.txtENTERFile input4.txt is empty.\n Test Case 5 Please enter the file name or type QUIT…arrow_forward
- C++ formatting please Write a program (not a function) that reads from a file named "data.csv". This file consists of a list of countries and gold medals they have won in various Olympic events. Write to standard out, the top 5 countries with the most gold medals. You can assume there will be no ties. Expected Output: 1: United States with 1022 gold medals 2: Soviet Union with 440 gold medals 3: Germany with 275 gold medals 4: Great Britain with 263 gold medals 5: China with 227 gold medalsarrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage double_input1.txt double_input2.txt PLEASE MODIFY THIS CODE, SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL THE TEST CASES, BECAUSE WHEN I UPLOAD IT TO HYPERGRADE IT DOES NOT PASS THE TEST CASES. IT HAS TO PASS ALL THE TEST CASES. I PROVIDED THE CORRECT OUTPUT AS A SCREENSHOT AS A REFERRENCE. import java.io.BufferedReader;import java.io.FileReader;import java.io.IOException;import java.util.InputMismatchException;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String fileName; do {…arrow_forward#function read file and display reportdef displayAverage(fileName):#open the fileinfile = open(fileName, "r")#variable declaration and initializationtotal = 0count = 0#read filefor num in infile:total = total + float(num)count = count + 1#close the fileinfile.close()#calculate averageaverage = total / count#display the resultprint("Average {:.1f}".format(average)) #method callingdisplayAverage("numbers.txt")arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
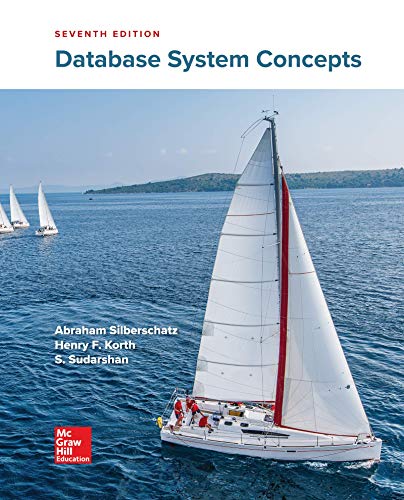
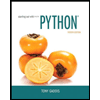
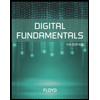
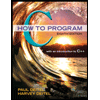
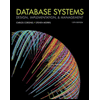
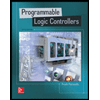