Give complete java code for a class named Bug that models a bug moving along a horizontal line. The bug can only move right or left. (i.e the bug is moving only along the x-axis). Initially the bug is facing right, but it can turn to change its direction. (i.e. if the bug is facing right, and then receives a turn command, the bug will now be facing left). In each move, its position changes by one unit in the current direction. The Bug class will need instance variables to store the current position of the bug, as well as the current direction it is facing. You need to declare and define a constructor that accepts an initial position, and initializes both instance variables. You must declare and define a turn () method, a move () method, and a getPosition () method. The following shows code that would be contained in a BugTester class. You do NOT need to write the BugTester class, just the Bug class as described above. new Bug (10);//creates new bug at position 10.facing Bug bugsy right bugsy. move (); //Now the position is 11 bugsy turn (); //Now facing left kugsy.move (); //Now the position is 10 %3D
Give complete java code for a class named Bug that models a bug moving along a horizontal line. The bug can only move right or left. (i.e the bug is moving only along the x-axis). Initially the bug is facing right, but it can turn to change its direction. (i.e. if the bug is facing right, and then receives a turn command, the bug will now be facing left). In each move, its position changes by one unit in the current direction. The Bug class will need instance variables to store the current position of the bug, as well as the current direction it is facing. You need to declare and define a constructor that accepts an initial position, and initializes both instance variables. You must declare and define a turn () method, a move () method, and a getPosition () method. The following shows code that would be contained in a BugTester class. You do NOT need to write the BugTester class, just the Bug class as described above. new Bug (10);//creates new bug at position 10.facing Bug bugsy right bugsy. move (); //Now the position is 11 bugsy turn (); //Now facing left kugsy.move (); //Now the position is 10 %3D
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help writing this code

Transcribed Image Text:## Java Bug Class Exercise
### Introduction
In this exercise, you will write a complete Java code for a class named `Bug` that models a bug moving along a horizontal line. The bug can only move right or left along the x-axis.
### Requirements
- **Initial direction**: The bug initially faces right.
- **Direction change**: The bug can turn to change its direction. For instance, if the bug is facing right, and then receives a turn command, the bug will now be facing left.
- **Movement**: Each move changes the bug's position by one unit in the current direction.
### Class Details
The `Bug` class requires instance variables to store:
1. The **current position** of the bug.
2. The **current direction** it is facing.
### Tasks
1. Declare and define a **constructor** that accepts an initial position and initializes both instance variables.
2. Define the following methods:
- `turn()`: Changes the bug's direction.
- `move()`: Moves the bug one unit in the current direction.
- `getPosition()`: Returns the current position of the bug.
### Example
The following code snippet demonstrates the usage of the `Bug` class within a `BugTester` class. You only need to implement the `Bug` class as described.
```java
Bug bugsy = new Bug(10); // Creates new bug at position 10, facing right
bugsy.move(); // Now the position is 11
bugsy.turn(); // Now facing left
bugsy.move(); // Now the position is 10
```
### Explanation
- **Bug instantiation**: A new `Bug` is created at position 10, facing right.
- **Move**: The bug moves right, changing its position to 11.
- **Turn**: The bug turns to face left.
- **Move again**: The bug moves left, changing its position back to 10.
This exercise helps practice basic object-oriented programming by implementing a simple class with specified behaviors and methods.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
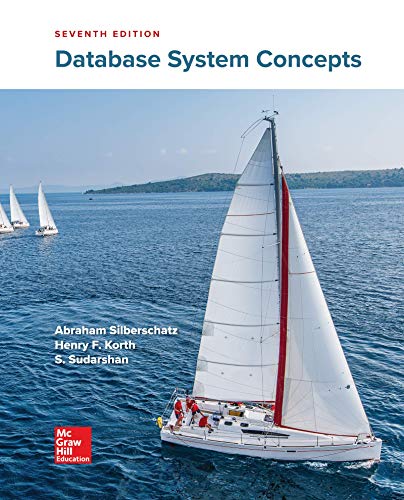
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
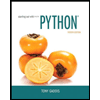
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
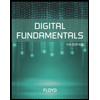
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
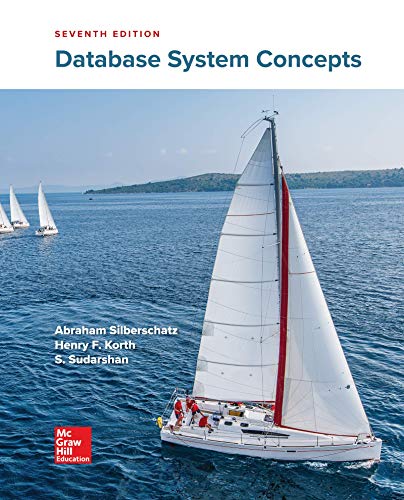
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
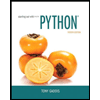
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
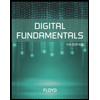
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
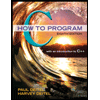
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
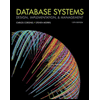
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
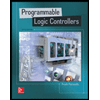
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education